panel.util Package#
util
Package#
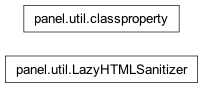
Various general utilities used in the panel codebase.
- class panel.util.LazyHTMLSanitizer(**kwargs)[source]#
Bases:
object
Wraps bleach.sanitizer.Cleaner lazily importing it on the first call to the clean method.
- panel.util.abbreviated_repr(value, max_length=25, natural_breaks=(',', ' '))[source]#
Returns an abbreviated repr for the supplied object. Attempts to find a natural break point while adhering to the maximum length.
- panel.util.base_version(version: str) str [source]#
Extract the final release and if available pre-release (alpha, beta, release candidate) segments of a PEP440 version, defined with three components (major.minor.micro).
Useful to avoid nbsite/sphinx to display the documentation HTML title with a not so informative and rather ugly long version (e.g.
0.13.0a19.post4+g0695e214
). Use it inconf.py
:version = release = base_version(package.__version__)
Return the version passed as input if no match is found with the pattern.
- panel.util.datetime_as_utctimestamp(value)[source]#
Converts a datetime to a UTC timestamp used by Bokeh internally.
- panel.util.decode_token(token: str, signed: bool = True) dict[str, Any] [source]#
Decodes a signed or unsigned JWT token.
- panel.util.flatten(line)[source]#
Flatten an arbitrarily nested sequence.
Inspired by: pd.core.common.flatten
- Parameters:
line (sequence) – The sequence to flatten
Notes
This only flattens list, tuple, and dict sequences.
- Returns:
flattened
- Return type:
generator
- panel.util.full_groupby(l, key=<function <lambda>>)[source]#
Groupby implementation which does not require a prior sort
- panel.util.fullpath(path: Union[AnyStr, PathLike]) Union[AnyStr, PathLike] [source]#
Expanduser and then abspath for a given path
- panel.util.indexOf(obj, objs)[source]#
Returns the index of an object in a list of objects. Unlike the list.index method this function only checks for identity not equality.
- panel.util.param_name(name: str) str [source]#
Removes the integer id from a Parameterized class name.
- panel.util.param_reprs(parameterized, skip=None)[source]#
Returns a list of reprs for parameters on the parameterized object. Skips default and empty values.
- panel.util.parse_query(query: str) dict[str, Any] [source]#
Parses a url query string, e.g. ?a=1&b=2.1&c=string, converting numeric strings to int or float types.
- panel.util.styler_update(styler, new_df)[source]#
Updates the todo items on a pandas Styler object to apply to a new DataFrame.
- Parameters:
styler (pandas.io.formats.style.Styler) – Styler objects
new_df (pd.DataFrame) – New DataFrame to update the styler to do items
- Returns:
todos
- Return type:
list
checks
Module#
- panel.util.checks.is_holoviews(obj: Any) bool [source]#
Whether the object is a HoloViews type that can be rendered.
- panel.util.checks.is_parameterized(obj) bool [source]#
Whether an object is a Parameterized class or instance.
parameters
Module#
- panel.util.parameters.edit_readonly(parameterized: Parameterized) Iterator [source]#
Temporarily set parameters on Parameterized object to readonly=False to allow editing them.
- panel.util.parameters.extract_dependencies(function)[source]#
Extract references from a method or function that declares the references.
warnings
Module#
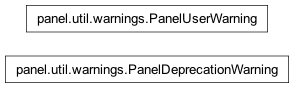
- exception panel.util.warnings.PanelDeprecationWarning[source]#
Bases:
DeprecationWarning
A Panel-specific
DeprecationWarning
subclass. Used to selectively filter Panel deprecations for unconditional display.- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- exception panel.util.warnings.PanelUserWarning[source]#
Bases:
UserWarning
A Panel-specific
UserWarning
subclass. Used to selectively filter Panel warnings for unconditional display.- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- panel.util.warnings.find_stack_level() int [source]#
Find the first place in the stack that is not inside Panel and Param. Inspired by: pandas.util._exceptions.find_stack_level