panel.io Package#
io
Package#
The io module contains utilities for loading JS components, embedding model state, and rendering panel objects.
- class panel.io.PeriodicCallback(*, callback, count, counter, log, period, running, timeout, name)[source]#
Bases:
Parameterized
Periodic encapsulates a periodic callback which will run both in tornado based notebook environments and on bokeh server. By default the callback will run until the stop method is called, but count and timeout values can be set to limit the number of executions or the maximum length of time for which the callback will run. The callback may also be started and stopped by setting the running parameter to True or False respectively.
callback
= param.Callable(allow_None=True, allow_refs=False, label=’Callback’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1445a6e50>)The callback to execute periodically.
counter
= param.Integer(allow_refs=False, default=0, inclusive_bounds=(True, True), label=’Counter’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144156390>)Counts the number of executions.
count
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Count’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14488a5d0>)Number of times the callback will be executed, by default this is unlimited.
log
= param.Boolean(allow_refs=False, default=True, label=’Log’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14488a1d0>)Whether the periodic callback should log its actions.
period
= param.Integer(allow_refs=False, default=500, inclusive_bounds=(True, True), label=’Period’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144889e10>)Period in milliseconds at which the callback is executed.
timeout
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Timeout’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14488a250>)Timeout in milliseconds from the start time at which the callback expires.
running
= param.Boolean(allow_refs=False, default=False, label=’Running’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144889d50>)Toggles whether the periodic callback is currently running.
- class panel.io.Resources(*args, absolute=False, notebook=False, **kwargs)[source]#
Bases:
Resources
- panel.io.hold(doc: Document, policy: HoldPolicyType = 'combine', comm: Comm | None = None)[source]#
Context manager that holds events on a particular Document allowing them all to be collected and dispatched when the context manager exits. This allows multiple events on the same object to be combined if the policy is set to ‘combine’.
- Parameters:
doc (Document) – The Bokeh Document to hold events on.
policy (HoldPolicyType) – One of ‘combine’, ‘collect’ or None determining whether events setting the same property are combined or accumulated to be dispatched when the context manager exits.
comm (Comm) – The Comm to dispatch events on when the context manager exits.
- panel.io.immediate_dispatch(doc: bokeh.document.document.Document | None = None)[source]#
Context manager to trigger immediate dispatch of events triggered inside the execution context even when Document events are currently on hold.
- Parameters:
doc (Document) – The document to dispatch events on (if None then state.curdoc is used).
- panel.io.ipywidget(obj: Any, doc=None, **kwargs: Any)[source]#
Returns an ipywidget model which renders the Panel object.
Requires jupyter_bokeh to be installed.
- Parameters:
obj (object) – Any Panel object or object which can be rendered with Panel
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
**kwargs (dict) – Keyword arguments passed to the pn.panel utility function
- Return type:
Returns an ipywidget model which renders the Panel object.
- panel.io.profile(name, engine='pyinstrument')[source]#
A decorator which may be added to any function to record profiling output.
- Parameters:
name (str) – A unique name for the profiling session.
engine (str) – The profiling engine, e.g. ‘pyinstrument’, ‘snakeviz’ or ‘memray’
- panel.io.push(doc: Document, comm: Comm, binary: bool = True, msg: any = None) None [source]#
Pushes events stored on the document across the provided comm.
- panel.io.push_notebook(*objs: Viewable) None [source]#
A utility for pushing updates to the frontend given a Panel object. This is required when modifying any Bokeh object directly in a notebook session.
- Parameters:
objs (panel.viewable.Viewable) –
- panel.io.serve(panels: TViewableFuncOrPath | Mapping[str, TViewableFuncOrPath], port: int = 0, address: Optional[str] = None, websocket_origin: Optional[str | list[str]] = None, loop: Optional[IOLoop] = None, show: bool = True, start: bool = True, title: Optional[str] = None, verbose: bool = True, location: bool = True, threaded: bool = False, admin: bool = False, **kwargs) StoppableThread | Server [source]#
Allows serving one or more panel objects on a single server. The panels argument should be either a Panel object or a function returning a Panel object or a dictionary of these two. If a dictionary is supplied the keys represent the slugs at which each app is served, e.g. serve({‘app’: panel1, ‘app2’: panel2}) will serve apps at /app and /app2 on the server.
Reference: https://panel.holoviz.org/user_guide/Server_Configuration.html#serving-multiple-apps
- Parameters:
panel (Viewable, function or {str: Viewable or function}) – A Panel object, a function returning a Panel object or a dictionary mapping from the URL slug to either.
port (int (optional, default=0)) – Allows specifying a specific port
address (str) – The address the server should listen on for HTTP requests.
websocket_origin (str or list(str) (optional)) –
A list of hosts that can connect to the websocket.
This is typically required when embedding a server app in an external web site.
If None, “localhost” is used.
loop (tornado.ioloop.IOLoop (optional, default=IOLoop.current())) – The tornado IOLoop to run the Server on
show (boolean (optional, default=True)) – Whether to open the server in a new browser tab on start
start (boolean(optional, default=True)) – Whether to start the Server
title (str or {str: str} (optional, default=None)) – An HTML title for the application or a dictionary mapping from the URL slug to a customized title
verbose (boolean (optional, default=True)) – Whether to print the address and port
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
threaded (boolean (default=False)) – Whether to start the server on a new Thread
admin (boolean (default=False)) – Whether to enable the admin panel
kwargs (dict) – Additional keyword arguments to pass to Server instance
- panel.io.unlocked() Iterator [source]#
Context manager which unlocks a Document and dispatches ModelChangedEvents triggered in the context body to all sockets on current sessions.
- panel.io.with_lock(func: Callable) Callable [source]#
Wrap a callback function to execute with a lock allowing the function to modify bokeh models directly.
- Parameters:
func (callable) – The callable to wrap
- Returns:
wrapper – Function wrapped to execute without a Document lock.
- Return type:
callable
admin
Module#

- class panel.io.admin.Data(*, data, name)[source]#
Bases:
Parameterized
data
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Data’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144947450>)
- class panel.io.admin.LogDataHandler(data)[source]#
Bases:
StreamHandler
- close()[source]#
Tidy up any resources used by the handler.
This version removes the handler from an internal map of handlers, _handlers, which is used for handler lookup by name. Subclasses should ensure that this gets called from overridden close() methods.
- emit(record)[source]#
Emit a record.
If a formatter is specified, it is used to format the record. The record is then written to the stream with a trailing newline. If exception information is present, it is formatted using traceback.print_exception and appended to the stream. If the stream has an ‘encoding’ attribute, it is used to determine how to do the output to the stream.
- filter(record)[source]#
Determine if a record is loggable by consulting all the filters.
The default is to allow the record to be logged; any filter can veto this and the record is then dropped. Returns a zero value if a record is to be dropped, else non-zero.
Changed in version 3.2: Allow filters to be just callables.
- format(record)[source]#
Format the specified record.
If a formatter is set, use it. Otherwise, use the default formatter for the module.
- handle(record)[source]#
Conditionally emit the specified logging record.
Emission depends on filters which may have been added to the handler. Wrap the actual emission of the record with acquisition/release of the I/O thread lock. Returns whether the filter passed the record for emission.
- handleError(record)[source]#
Handle errors which occur during an emit() call.
This method should be called from handlers when an exception is encountered during an emit() call. If raiseExceptions is false, exceptions get silently ignored. This is what is mostly wanted for a logging system - most users will not care about errors in the logging system, they are more interested in application errors. You could, however, replace this with a custom handler if you wish. The record which was being processed is passed in to this method.
application
Module#

Extensions for Bokeh application handling.
- class panel.io.application.Application(*args, **kwargs)[source]#
Bases:
Application
Extends Bokeh Application with ability to add global session creation callbacks, support for the admin dashboard and the ability to globally define a template.
- create_document() Document [source]#
Creates and initializes a document using the Application’s handlers.
- property handlers: tuple[Handler, ...]#
The ordered list of handlers this Application is configured with.
- property metadata: dict[str, Any] | None#
Arbitrary user-supplied metadata to associate with this application.
- on_server_loaded(server_context: ServerContext) None [source]#
Invoked to execute code when a new session is created.
This method calls
on_server_loaded
on each handler, in order, with the server context passed as the only argument.
- on_server_unloaded(server_context: ServerContext) None [source]#
Invoked to execute code when the server cleanly exits. (Before stopping the server’s
IOLoop
.)This method calls
on_server_unloaded
on each handler, in order, with the server context passed as the only argument.Warning
In practice this code may not run, since servers are often killed by a signal.
- async on_session_created(session_context)[source]#
Invoked to execute code when a new session is created.
This method calls
on_session_created
on each handler, in order, with the session context passed as the only argument.May return a
Future
which will delay session creation until theFuture
completes.
- async on_session_destroyed(session_context: SessionContext) None [source]#
Invoked to execute code when a session is destroyed.
This method calls
on_session_destroyed
on each handler, in order, with the session context passed as the only argument.Afterwards,
session_context.destroyed
will beTrue
.
- process_request(request: HTTPServerRequest) dict[str, Any] [source]#
Processes incoming HTTP request returning a dictionary of additional data to add to the session_context.
- Parameters:
request – HTTP request
- Returns:
A dictionary of JSON serializable data to be included on the session context.
- property safe_to_fork: bool#
- property static_path: str | None#
Path to any (optional) static resources specified by handlers.
browser
Module#

Defines a BrowserInfo component exposing the browser window and navigator APIs.
- class panel.io.browser.BrowserInfo(*, dark_mode, device_pixel_ratio, language, timezone, timezone_offset, webdriver, name)[source]#
Bases:
Syncable
The Location component can be made available in a server context to provide read and write access to the URL components in the browser.
dark_mode
= param.Boolean(allow_None=True, allow_refs=False, label=’Dark mode’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144bc2990>)Whether the user prefers dark mode.
device_pixel_ratio
= param.Number(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Device pixel ratio’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144ba6990>)Provides the ratio of the resolution in physical pixels to the resolution in CSS pixels for the current display device.
language
= param.String(allow_None=True, allow_refs=False, label=’Language’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144bc2c50>)The preferred language of the user, usually the language of the browser UI.
timezone
= param.String(allow_None=True, allow_refs=False, label=’Timezone’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144bc25d0>)The timezone configured as the local timezone of the user.
timezone_offset
= param.Number(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Timezone offset’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144bc3a10>)The time offset from UTC in minutes.
webdriver
= param.Boolean(allow_None=True, allow_refs=False, label=’Webdriver’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144bc2610>)Indicates whether the user agent is controlled by automation.
- get_root(doc: Document | None = None, comm: Comm | None = None, preprocess: bool = True) Model [source]#
Returns the root model and applies pre-processing hooks
- Parameters:
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
comm (pyviz_comms.Comm) – Optional pyviz_comms when working in notebook
preprocess (boolean (default=True)) – Whether to run preprocessing hooks
- Return type:
Returns the bokeh model corresponding to this panel object
cache
Module#
Implements memoization for functions with arbitrary arguments
- panel.io.cache.cache(func=None, hash_funcs=None, max_items=None, policy='LRU', ttl=None, to_disk=False, cache_path='./cache', per_session=False)[source]#
Memoizes functions for a user session. Can be used as function annotation or just directly.
For global caching across user sessions use pn.state.as_cached.
- Parameters:
func (callable) – The function to cache.
hash_funcs (dict or None) – A dictionary mapping from a type to a function which returns a hash for an object of that type. If provided this will override the default hashing function provided by Panel.
max_items (int or None) – The maximum items to keep in the cache. Default is None, which does not limit number of items stored in the cache.
policy (str) –
- A caching policy when max_items is set, must be one of:
FIFO: First in - First out
LRU: Least recently used
LFU: Least frequently used
ttl (float or None) – The number of seconds to keep an item in the cache, or None if the cache should not expire. The default is None.
to_disk (bool) – Whether to cache to disk using diskcache.
cache_dir (str) – Directory to cache to on disk.
per_session (bool) – Whether to cache data only for the current session.
- panel.io.cache.compute_hash(func, hash_funcs, args, kwargs)[source]#
Computes a hash given a function and its arguments.
- Parameters:
func (callable) – The function to cache.
hash_funcs (dict) – A dictionary of custom hash functions indexed by type
args (tuple) – Arguments to hash
kwargs (dict) – Keyword arguments to hash
callbacks
Module#

Defines callbacks to be executed on a thread or by scheduling it on a running bokeh server.
- class panel.io.callbacks.PeriodicCallback(*, callback, count, counter, log, period, running, timeout, name)[source]#
Bases:
Parameterized
Periodic encapsulates a periodic callback which will run both in tornado based notebook environments and on bokeh server. By default the callback will run until the stop method is called, but count and timeout values can be set to limit the number of executions or the maximum length of time for which the callback will run. The callback may also be started and stopped by setting the running parameter to True or False respectively.
callback
= param.Callable(allow_None=True, allow_refs=False, label=’Callback’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c8ea10>)The callback to execute periodically.
counter
= param.Integer(allow_refs=False, default=0, inclusive_bounds=(True, True), label=’Counter’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144d066d0>)Counts the number of executions.
count
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Count’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c45dd0>)Number of times the callback will be executed, by default this is unlimited.
log
= param.Boolean(allow_refs=False, default=True, label=’Log’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c45f10>)Whether the periodic callback should log its actions.
period
= param.Integer(allow_refs=False, default=500, inclusive_bounds=(True, True), label=’Period’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c44110>)Period in milliseconds at which the callback is executed.
timeout
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Timeout’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c69350>)Timeout in milliseconds from the start time at which the callback expires.
running
= param.Boolean(allow_refs=False, default=False, label=’Running’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x144c46490>)Toggles whether the periodic callback is currently running.
convert
Module#

- class panel.io.convert.MockSessionContext(*args, document=None, **kwargs)[source]#
Bases:
SessionContext
- property destroyed: bool#
If
True
, the session has been discarded and cannot be used.A new session with the same ID could be created later but this instance will not come back to life.
- property id: ID#
The unique ID for the session associated with this context.
- property server_context: ServerContext#
The server context for this session context
- with_locked_document(*args)[source]#
Runs a function with the document lock held, passing the document to the function.
Subclasses must implement this method.
- Parameters:
func (callable) – function that takes a single parameter (the Document) and returns
None
or aFuture
- Returns:
a
Future
containing the result of the function
- class panel.io.convert.Request(headers: 'dict', cookies: 'dict', arguments: 'dict')[source]#
Bases:
object
- panel.io.convert.convert_apps(apps: Union[str, PathLike, List[str | os.PathLike]], dest_path: str | os.PathLike | None = None, title: str | None = None, runtime: Literal['pyodide', 'pyscript', 'pyodide-worker', 'pyscript-worker'] = 'pyodide-worker', requirements: Union[List[str], Literal['auto'], PathLike] = 'auto', prerender: bool = True, build_index: bool = True, build_pwa: bool = True, pwa_config: Dict[Any, Any] = {}, max_workers: int = 4, panel_version: Union[Literal['auto', 'local'], str] = 'auto', http_patch: bool = True, inline: bool = False, compiled: bool = False, verbose: bool = True)[source]#
- apps: str | List[str]
The filename(s) of the Panel/Bokeh application(s) to convert.
- dest_path: str | pathlib.Path
The directory to write the converted application(s) to.
- title: str | None
A title for the application(s). Also used to generate unique name for the application cache to ensure.
- runtime: ‘pyodide’ | ‘pyscript’ | ‘pyodide-worker’
The runtime to use for running Python in the browser.
- requirements: ‘auto’ | List[str] | os.PathLike | Dict[str, ‘auto’ | List[str] | os.PathLike]
The list of requirements to include (in addition to Panel). By default automatically infers dependencies from imports in the application. May also provide path to a requirements.txt
- prerender: bool
Whether to pre-render the components so the page loads.
- build_index: bool
Whether to write an index page (if there are multiple apps).
- build_pwa: bool
Whether to write files to define a progressive web app (PWA) including a manifest and a service worker that caches the application locally
- pwa_config: Dict[Any, Any]
Configuration for the PWA including (see https://developer.mozilla.org/en-US/docs/Web/Manifest)
display: Display options (‘fullscreen’, ‘standalone’, ‘minimal-ui’ ‘browser’)
orientation: Preferred orientation
background_color: The background color of the splash screen
theme_color: The theme color of the application
- max_workers: int
The maximum number of parallel workers
panel_version: ‘auto’ | ‘local’] | str
- ‘ The panel version to include.
- http_patch: bool
Whether to patch the HTTP request stack with the pyodide-http library to allow urllib3 and requests to work.
- inline: bool
Whether to inline resources.
- compiled: bool
Whether to use the compiled and faster version of Pyodide.
- panel.io.convert.script_to_html(filename: str | os.PathLike | IO, requirements: Union[Literal['auto'], List[str]] = 'auto', js_resources: Union[Literal['auto'], List[str]] = 'auto', css_resources: Optional[Union[Literal['auto'], List[str]]] = 'auto', runtime: Literal['pyodide', 'pyscript', 'pyodide-worker', 'pyscript-worker'] = 'pyodide', prerender: bool = True, panel_version: Union[Literal['auto', 'local'], str] = 'auto', manifest: str | None = None, http_patch: bool = True, inline: bool = False, compiled: bool = True) str [source]#
Converts a Panel or Bokeh script to a standalone WASM Python application.
- Parameters:
filename (str | Path | IO) – The filename of the Panel/Bokeh application to convert.
requirements ('auto' | List[str]) – The list of requirements to include (in addition to Panel).
js_resources ('auto' | List[str]) – The list of JS resources to include in the exported HTML.
css_resources ('auto' | List[str] | None) – The list of CSS resources to include in the exported HTML.
runtime ('pyodide' | 'pyscript') – The runtime to use for running Python in the browser.
prerender (bool) – Whether to pre-render the components so the page loads.
panel_version ('auto' | str) – The panel release version to use in the exported HTML.
http_patch (bool) – Whether to patch the HTTP request stack with the pyodide-http library to allow urllib3 and requests to work.
inline (bool) – Whether to inline resources.
compiled (bool) – Whether to use pre-compiled pyodide bundles.
datamodel
Module#

- class panel.io.datamodel.Parameterized(*, default: Union[T, UndefinedType, IntrinsicType] = Intrinsic, help: str | None = None)[source]#
Bases:
Property
Accept a Parameterized object.
This property only exists to support type validation, e.g. for “accepts” clauses. It is not serializable itself, and is not useful to add to Bokeh models directly.
- accepts(tp: Union[type[bokeh.core.property.bases.Property[Any]], Property[Any]], converter: Callable[[Any], T]) Property[T] [source]#
Declare that other types may be converted to this property type.
- Parameters:
tp (Property) – A type that may be converted automatically to this property type.
converter (callable) – A function accepting
value
to perform conversion of the value to this property type.
- Returns:
self
- asserts(fn: Callable[[HasProps, T], bool], msg_or_fn: Union[str, Callable[[HasProps, str, T], None]]) Property[T] [source]#
Assert that prepared values satisfy given conditions.
Assertions are intended in enforce conditions beyond simple value type validation. For instance, this method can be use to assert that the columns of a
ColumnDataSource
all collectively have the same length at all times.- Parameters:
fn (callable) – A function accepting
(obj, value)
that returns True if the value passes the assertion, or False otherwise.msg_or_fn (str or callable) – A message to print in case the assertion fails, or a function accepting
(obj, name, value)
to call in in case the assertion fails.
- Returns:
self
- is_valid(value: Any) bool [source]#
Whether the value passes validation
- Parameters:
value (obj) – the value to validate against this property type
- Returns:
True if valid, False otherwise
- make_descriptors(name: str) list[bokeh.core.property.descriptors.PropertyDescriptor[T]] [source]#
Return a list of
PropertyDescriptor
instances to install on a class, in order to delegate attribute access to this property.- Parameters:
name (str) – the name of the property these descriptors are for
- Returns:
list[PropertyDescriptor]
The descriptors returned are collected by the
MetaHasProps
metaclass and added toHasProps
subclasses during class creation.
- matches(new: T, old: T) bool [source]#
Whether two parameters match values.
If either
new
orold
is a NumPy array or Pandas Series or Index, then the result ofnp.array_equal
will determine if the values match.Otherwise, the result of standard Python equality will be returned.
- Returns:
True, if new and old match, False otherwise
- property readonly: bool#
Whether this property is read-only.
Read-only properties may only be modified by the client (i.e., by BokehJS in the browser).
- property serialized: bool#
Whether the property should be serialized when serializing an object.
This would be False for a “virtual” or “convenience” property that duplicates information already available in other properties, for example.
- themed_default(cls: type[bokeh.core.has_props.HasProps], name: str, theme_overrides: dict[str, Any] | None, *, no_eval: bool = False) T [source]#
The default, transformed by prepare_value() and the theme overrides.
- class panel.io.datamodel.ParameterizedList(*, default: Union[T, UndefinedType, IntrinsicType] = Intrinsic, help: str | None = None)[source]#
Bases:
Property
Accept a list of Parameterized objects.
This property only exists to support type validation, e.g. for “accepts” clauses. It is not serializable itself, and is not useful to add to Bokeh models directly.
- accepts(tp: Union[type[bokeh.core.property.bases.Property[Any]], Property[Any]], converter: Callable[[Any], T]) Property[T] [source]#
Declare that other types may be converted to this property type.
- Parameters:
tp (Property) – A type that may be converted automatically to this property type.
converter (callable) – A function accepting
value
to perform conversion of the value to this property type.
- Returns:
self
- asserts(fn: Callable[[HasProps, T], bool], msg_or_fn: Union[str, Callable[[HasProps, str, T], None]]) Property[T] [source]#
Assert that prepared values satisfy given conditions.
Assertions are intended in enforce conditions beyond simple value type validation. For instance, this method can be use to assert that the columns of a
ColumnDataSource
all collectively have the same length at all times.- Parameters:
fn (callable) – A function accepting
(obj, value)
that returns True if the value passes the assertion, or False otherwise.msg_or_fn (str or callable) – A message to print in case the assertion fails, or a function accepting
(obj, name, value)
to call in in case the assertion fails.
- Returns:
self
- is_valid(value: Any) bool [source]#
Whether the value passes validation
- Parameters:
value (obj) – the value to validate against this property type
- Returns:
True if valid, False otherwise
- make_descriptors(name: str) list[bokeh.core.property.descriptors.PropertyDescriptor[T]] [source]#
Return a list of
PropertyDescriptor
instances to install on a class, in order to delegate attribute access to this property.- Parameters:
name (str) – the name of the property these descriptors are for
- Returns:
list[PropertyDescriptor]
The descriptors returned are collected by the
MetaHasProps
metaclass and added toHasProps
subclasses during class creation.
- matches(new: T, old: T) bool [source]#
Whether two parameters match values.
If either
new
orold
is a NumPy array or Pandas Series or Index, then the result ofnp.array_equal
will determine if the values match.Otherwise, the result of standard Python equality will be returned.
- Returns:
True, if new and old match, False otherwise
- property readonly: bool#
Whether this property is read-only.
Read-only properties may only be modified by the client (i.e., by BokehJS in the browser).
- property serialized: bool#
Whether the property should be serialized when serializing an object.
This would be False for a “virtual” or “convenience” property that duplicates information already available in other properties, for example.
- themed_default(cls: type[bokeh.core.has_props.HasProps], name: str, theme_overrides: dict[str, Any] | None, *, no_eval: bool = False) T [source]#
The default, transformed by prepare_value() and the theme overrides.
- transform(value: Any) T [source]#
Change the value into the canonical format for this property.
- Parameters:
value (obj) – the value to apply transformation to.
- Returns:
transformed value
- Return type:
obj
- validate(value, detail=True)[source]#
Determine whether we can set this property from this value.
Validation happens before transform()
- Parameters:
value (obj) – the value to validate against this property type
detail (bool, options) –
whether to construct detailed exceptions
Generating detailed type validation error messages can be expensive. When doing type checks internally that will not escape exceptions to users, these messages can be skipped by setting this value to False (default: True)
- Returns:
None
- Raises:
ValueError if the value is not valid for this property type –
- panel.io.datamodel.construct_data_model(parameterized, name=None, ignore=[], types={})[source]#
Dynamically creates a Bokeh DataModel class from a Parameterized object.
- Parameters:
parameterized (param.Parameterized) – The Parameterized class or instance from which to create the DataModel
name (str or None) – Name of the dynamically created DataModel class
ignore (list(str)) – List of parameters to ignore.
types (dict) – A dictionary mapping from parameter name to a Parameter type, making it possible to override the default parameter types.
- Return type:
DataModel
- panel.io.datamodel.create_linked_datamodel(obj, root=None)[source]#
Creates a Bokeh DataModel from a Parameterized class or instance which automatically links the parameters bi-directionally.
- Parameters:
obj (param.Parameterized) – The Parameterized class to create a linked DataModel for.
- Return type:
DataModel instance linked to the Parameterized object.
django
Module#
document
Module#

- class panel.io.document.MockSessionContext(*args, document=None, **kwargs)[source]#
Bases:
SessionContext
- property destroyed: bool#
If
True
, the session has been discarded and cannot be used.A new session with the same ID could be created later but this instance will not come back to life.
- property id: ID#
The unique ID for the session associated with this context.
- property server_context: ServerContext#
The server context for this session context
- with_locked_document(*args)[source]#
Runs a function with the document lock held, passing the document to the function.
Subclasses must implement this method.
- Parameters:
func (callable) – function that takes a single parameter (the Document) and returns
None
or aFuture
- Returns:
a
Future
containing the result of the function
- class panel.io.document.Request(headers: 'dict', cookies: 'dict', arguments: 'dict')[source]#
Bases:
object
- panel.io.document.freeze_doc(doc: Document, model: HasProps, properties: Dict[str, Any], force: bool = False)[source]#
Freezes the document model references if any of the properties are themselves a model.
- panel.io.document.immediate_dispatch(doc: bokeh.document.document.Document | None = None)[source]#
Context manager to trigger immediate dispatch of events triggered inside the execution context even when Document events are currently on hold.
- Parameters:
doc (Document) – The document to dispatch events on (if None then state.curdoc is used).
- panel.io.document.unlocked() Iterator [source]#
Context manager which unlocks a Document and dispatches ModelChangedEvents triggered in the context body to all sockets on current sessions.
- panel.io.document.with_lock(func: Callable) Callable [source]#
Wrap a callback function to execute with a lock allowing the function to modify bokeh models directly.
- Parameters:
func (callable) – The callable to wrap
- Returns:
wrapper – Function wrapped to execute without a Document lock.
- Return type:
callable
embed
Module#
Various utilities for recording and embedding state in a rendered app.
- panel.io.embed.embed_state(panel, model, doc, max_states=1000, max_opts=3, json=False, json_prefix='', save_path='./', load_path=None, progress=True, states={})[source]#
Embeds the state of the application on a State model which allows exporting a static version of an app. This works by finding all widgets with a predefined set of options and evaluating the cross product of the widget values and recording the resulting events to be replayed when exported. The state is recorded on a State model which is attached as an additional root on the Document.
- Parameters:
panel (panel.reactive.Reactive) – The Reactive component being exported
model (bokeh.model.Model) – The bokeh model being exported
doc (bokeh.document.Document) – The bokeh Document being exported
max_states (int (default=1000)) – The maximum number of states to export
max_opts (int (default=3)) – The max number of ticks sampled in a continuous widget like a slider
json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for JSON filename
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=True)) – Whether to report progress
states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
handlers
Module#

- class panel.io.handlers.MarkdownHandler(*args, **kwargs)[source]#
Bases:
PanelCodeHandler
Modify Bokeh documents by creating Dashboard from a Markdown file.
- property error: str | None#
If the handler fails, may contain a related error message.
- property error_detail: str | None#
If the handler fails, may contain a traceback or other details.
- property failed: bool#
True
if the handler failed to modify the doc
- modify_document(doc: Document)[source]#
Modify an application document in a specified manner.
When a Bokeh server session is initiated, the Bokeh server asks the Application for a new Document to service the session. To do this, the Application first creates a new empty Document, then it passes this Document to the
modify_document
method of each of its handlers. When all handlers have updated the Document, it is used to service the user session.Subclasses must implement this method
- Parameters:
doc (Document) – A Bokeh Document to update in-place
- Returns:
Document
- on_server_loaded(server_context: ServerContext) None [source]#
Execute code when the server is first started.
Subclasses may implement this method to provide for any one-time initialization that is necessary after the server starts, but before any sessions are created.
- Parameters:
server_context (ServerContext) –
- on_server_unloaded(server_context: ServerContext) None [source]#
Execute code when the server cleanly exits. (Before stopping the server’s
IOLoop
.)Subclasses may implement this method to provide for any one-time tear down that is necessary before the server exits.
- Parameters:
server_context (ServerContext) –
Warning
In practice this code may not run, since servers are often killed by a signal.
- async on_session_created(session_context: SessionContext) None [source]#
Execute code when a new session is created.
Subclasses may implement this method to provide for any per-session initialization that is necessary before
modify_doc
is called for the session.- Parameters:
session_context (SessionContext) –
- async on_session_destroyed(session_context: SessionContext) None [source]#
Execute code when a session is destroyed.
Subclasses may implement this method to provide for any per-session tear-down that is necessary when sessions are destroyed.
- Parameters:
session_context (SessionContext) –
- process_request(request: HTTPServerRequest) dict[str, Any] [source]#
Processes incoming HTTP request returning a dictionary of additional data to add to the session_context.
- Parameters:
request – HTTP request
- Returns:
A dictionary of JSON serializable data to be included on the session context.
- property safe_to_fork: bool#
Whether it is still safe for the Bokeh server to fork new workers.
False
if the code has already been executed.
- class panel.io.handlers.NotebookHandler(*, filename: Union[str, PathLike[str]], argv: list[str] = [], package: module | None = None)[source]#
Bases:
PanelCodeHandler
Modify Bokeh documents by creating Dashboard from a notebook file.
- property error: str | None#
If the handler fails, may contain a related error message.
- property error_detail: str | None#
If the handler fails, may contain a traceback or other details.
- property failed: bool#
True
if the handler failed to modify the doc
- modify_document(doc: Document) None [source]#
Run Bokeh application code to update a
Document
- Parameters:
(Document) (doc) –
- on_server_loaded(server_context: ServerContext) None [source]#
Execute code when the server is first started.
Subclasses may implement this method to provide for any one-time initialization that is necessary after the server starts, but before any sessions are created.
- Parameters:
server_context (ServerContext) –
- on_server_unloaded(server_context: ServerContext) None [source]#
Execute code when the server cleanly exits. (Before stopping the server’s
IOLoop
.)Subclasses may implement this method to provide for any one-time tear down that is necessary before the server exits.
- Parameters:
server_context (ServerContext) –
Warning
In practice this code may not run, since servers are often killed by a signal.
- async on_session_created(session_context: SessionContext) None [source]#
Execute code when a new session is created.
Subclasses may implement this method to provide for any per-session initialization that is necessary before
modify_doc
is called for the session.- Parameters:
session_context (SessionContext) –
- async on_session_destroyed(session_context: SessionContext) None [source]#
Execute code when a session is destroyed.
Subclasses may implement this method to provide for any per-session tear-down that is necessary when sessions are destroyed.
- Parameters:
session_context (SessionContext) –
- process_request(request: HTTPServerRequest) dict[str, Any] [source]#
Processes incoming HTTP request returning a dictionary of additional data to add to the session_context.
- Parameters:
request – HTTP request
- Returns:
A dictionary of JSON serializable data to be included on the session context.
- property safe_to_fork: bool#
Whether it is still safe for the Bokeh server to fork new workers.
False
if the code has already been executed.
- class panel.io.handlers.PanelCodeHandler(*, source: str, filename: Union[str, PathLike[str]], argv: list[str] = [], package: module | None = None)[source]#
Bases:
CodeHandler
Modify Bokeh documents by creating Dashboard from code.
Additionally this subclass adds support for the ability to:
Log session launch, load and destruction
Capture document_ready events to track when app is loaded.
Add profiling support
Ensure that state.curdoc is set
Reload the application module if autoreload is enabled
Track modules loaded during app execution to enable autoreloading
- property error: str | None#
If the handler fails, may contain a related error message.
- property error_detail: str | None#
If the handler fails, may contain a traceback or other details.
- property failed: bool#
True
if the handler failed to modify the doc
- modify_document(doc: Document)[source]#
Modify an application document in a specified manner.
When a Bokeh server session is initiated, the Bokeh server asks the Application for a new Document to service the session. To do this, the Application first creates a new empty Document, then it passes this Document to the
modify_document
method of each of its handlers. When all handlers have updated the Document, it is used to service the user session.Subclasses must implement this method
- Parameters:
doc (Document) – A Bokeh Document to update in-place
- Returns:
Document
- on_server_loaded(server_context: ServerContext) None [source]#
Execute code when the server is first started.
Subclasses may implement this method to provide for any one-time initialization that is necessary after the server starts, but before any sessions are created.
- Parameters:
server_context (ServerContext) –
- on_server_unloaded(server_context: ServerContext) None [source]#
Execute code when the server cleanly exits. (Before stopping the server’s
IOLoop
.)Subclasses may implement this method to provide for any one-time tear down that is necessary before the server exits.
- Parameters:
server_context (ServerContext) –
Warning
In practice this code may not run, since servers are often killed by a signal.
- async on_session_created(session_context: SessionContext) None [source]#
Execute code when a new session is created.
Subclasses may implement this method to provide for any per-session initialization that is necessary before
modify_doc
is called for the session.- Parameters:
session_context (SessionContext) –
- async on_session_destroyed(session_context: SessionContext) None [source]#
Execute code when a session is destroyed.
Subclasses may implement this method to provide for any per-session tear-down that is necessary when sessions are destroyed.
- Parameters:
session_context (SessionContext) –
- process_request(request: HTTPServerRequest) dict[str, Any] [source]#
Processes incoming HTTP request returning a dictionary of additional data to add to the session_context.
- Parameters:
request – HTTP request
- Returns:
A dictionary of JSON serializable data to be included on the session context.
- property safe_to_fork: bool#
Whether it is still safe for the Bokeh server to fork new workers.
False
if the code has already been executed.
- class panel.io.handlers.PanelCodeRunner(source: str, path: Union[str, PathLike[str]], argv: list[str], package: module | None = None)[source]#
Bases:
CodeRunner
- property doc: str | None#
Contents of docstring, if code contains one.
- property error: str | None#
If code execution fails, may contain a related error message.
- property error_detail: str | None#
If code execution fails, may contain a traceback or other details.
- property failed: bool#
True
if code execution failed
- property path: Union[str, PathLike[str]]#
The path that new modules will be configured with.
- reset_run_errors() None [source]#
Clears any transient error conditions from a previous run.
- Returns
None
- run(module: module, post_check: Optional[Callable[[], None]] = None) None [source]#
Execute the configured source code in a module and run any post checks.
See bokeh.application.handlers.code_runner for original implementation.
- property source: str#
The configured source code that will be executed when
run
is called.
- class panel.io.handlers.ScriptHandler(*, filename: Union[str, PathLike[str]], argv: list[str] = [], package: module | None = None)[source]#
Bases:
PanelCodeHandler
Modify Bokeh documents by creating Dashboard from a Python script.
- property error: str | None#
If the handler fails, may contain a related error message.
- property error_detail: str | None#
If the handler fails, may contain a traceback or other details.
- property failed: bool#
True
if the handler failed to modify the doc
- modify_document(doc: Document)[source]#
Modify an application document in a specified manner.
When a Bokeh server session is initiated, the Bokeh server asks the Application for a new Document to service the session. To do this, the Application first creates a new empty Document, then it passes this Document to the
modify_document
method of each of its handlers. When all handlers have updated the Document, it is used to service the user session.Subclasses must implement this method
- Parameters:
doc (Document) – A Bokeh Document to update in-place
- Returns:
Document
- on_server_loaded(server_context: ServerContext) None [source]#
Execute code when the server is first started.
Subclasses may implement this method to provide for any one-time initialization that is necessary after the server starts, but before any sessions are created.
- Parameters:
server_context (ServerContext) –
- on_server_unloaded(server_context: ServerContext) None [source]#
Execute code when the server cleanly exits. (Before stopping the server’s
IOLoop
.)Subclasses may implement this method to provide for any one-time tear down that is necessary before the server exits.
- Parameters:
server_context (ServerContext) –
Warning
In practice this code may not run, since servers are often killed by a signal.
- async on_session_created(session_context: SessionContext) None [source]#
Execute code when a new session is created.
Subclasses may implement this method to provide for any per-session initialization that is necessary before
modify_doc
is called for the session.- Parameters:
session_context (SessionContext) –
- async on_session_destroyed(session_context: SessionContext) None [source]#
Execute code when a session is destroyed.
Subclasses may implement this method to provide for any per-session tear-down that is necessary when sessions are destroyed.
- Parameters:
session_context (SessionContext) –
- process_request(request: HTTPServerRequest) dict[str, Any] [source]#
Processes incoming HTTP request returning a dictionary of additional data to add to the session_context.
- Parameters:
request – HTTP request
- Returns:
A dictionary of JSON serializable data to be included on the session context.
- property safe_to_fork: bool#
Whether it is still safe for the Bokeh server to fork new workers.
False
if the code has already been executed.
- panel.io.handlers.capture_code_cell(cell)[source]#
Parses a code cell and generates wrapper code to capture the return value of the cell and any other outputs published inside the cell.
- panel.io.handlers.extract_code(filehandle: IO, supported_syntax: tuple[str, ...] = ('{pyodide}', 'python')) str [source]#
Extracts Panel application code from a Markdown file.
- panel.io.handlers.parse_notebook(filename: str | os.PathLike | IO, preamble: list[str] | None = None)[source]#
Parses a notebook on disk and returns a script.
- Parameters:
filename (str | os.PathLike) – The notebook file to parse.
preamble (list[str]) – Any lines of code to prepend to the parsed code output.
- Returns:
nb (nbformat.NotebookNode) – nbformat dictionary-like representation of the notebook
code (str) – The parsed and converted script
cell_layouts (dict) – Dictionary containing the layout and positioning of cells.
ipywidget
Module#

- class panel.io.ipywidget.MessageSentBuffers[source]#
Bases:
TypedDict
- clear() None. Remove all items from D. #
- copy() a shallow copy of D #
- fromkeys(value=None, /)#
Create a new dictionary with keys from iterable and values set to value.
- get(key, default=None, /)#
Return the value for key if key is in the dictionary, else default.
- items() a set-like object providing a view on D's items #
- keys() a set-like object providing a view on D's keys #
- pop(k[, d]) v, remove specified key and return the corresponding value. #
If the key is not found, return the default if given; otherwise, raise a KeyError.
- popitem()#
Remove and return a (key, value) pair as a 2-tuple.
Pairs are returned in LIFO (last-in, first-out) order. Raises KeyError if the dict is empty.
- setdefault(key, default=None, /)#
Insert key with a value of default if key is not in the dictionary.
Return the value for key if key is in the dictionary, else default.
- update([E, ]**F) None. Update D from dict/iterable E and F. #
If E is present and has a .keys() method, then does: for k in E: D[k] = E[k] If E is present and lacks a .keys() method, then does: for k, v in E: D[k] = v In either case, this is followed by: for k in F: D[k] = F[k]
- values() an object providing a view on D's values #
- class panel.io.ipywidget.MessageSentEventPatched(document: Document, msg_type: str, msg_data: Any | bytes, setter: Setter | None = None, callback_invoker: Invoker | None = None)[source]#
Bases:
MessageSentEvent
Patches MessageSentEvent with fix that ensures MessageSent event does not define msg_data (which is an assumption in BokehJS Document.apply_json_patch.)
- dispatch(receiver: Any) None [source]#
Dispatch handling of this event to a receiver.
This method will invoke
receiver._document_patched
if it exists.
- class panel.io.ipywidget.PanelKernel(**kwargs: Any)[source]#
Bases:
Kernel
- add_traits(**traits: Any) None [source]#
Dynamically add trait attributes to the HasTraits instance.
- classmethod class_config_rst_doc() str [source]#
Generate rST documentation for this class’ config options.
Excludes traits defined on parent classes.
- classmethod class_config_section(classes: Optional[Sequence[type[traitlets.traitlets.HasTraits]]] = None) str [source]#
Get the config section for this class.
- Parameters:
classes (list, optional) – The list of other classes in the config file. Used to reduce redundant information.
- classmethod class_get_help(inst: traitlets.traitlets.HasTraits | None = None) str [source]#
Get the help string for this class in ReST format.
If inst is given, its current trait values will be used in place of class defaults.
- classmethod class_get_trait_help(trait: TraitType[Any, Any], inst: traitlets.traitlets.HasTraits | None = None, helptext: str | None = None) str [source]#
Get the helptext string for a single trait.
- Parameters:
inst – If given, its current trait values will be used in place of the class default.
helptext – If not given, uses the help attribute of the current trait.
- classmethod class_own_trait_events(name: str) dict[str, traitlets.traitlets.EventHandler] [source]#
Get a dict of all event handlers defined on this class, not a parent.
Works like
event_handlers
, except for excluding traits from parents.
- classmethod class_own_traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a dict of all the traitlets defined on this class, not a parent.
Works like class_traits, except for excluding traits from parents.
- classmethod class_print_help(inst: traitlets.traitlets.HasTraits | None = None) None [source]#
Get the help string for a single trait and print it.
- classmethod class_trait_names(**metadata: Any) list[str] [source]#
Get a list of all the names of this class’ traits.
This method is just like the
trait_names()
method, but is unbound.
- classmethod class_traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a
dict
of all the traits of this class. The dictionary is keyed on the name and the values are the TraitType objects.This method is just like the
traits()
method, but is unbound.The TraitTypes returned don’t know anything about the values that the various HasTrait’s instances are holding.
The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- property cross_validation_lock: Any#
A contextmanager for running a block with our cross validation lock set to True.
At the end of the block, the lock’s value is restored to its value prior to entering the block.
- debug_just_my_code#
Set to False if you want to debug python standard and dependent libraries.
- async dispatch_queue()[source]#
Coroutine to preserve order of message handling
Ensures that only one message is processing at a time, even when the handler is async
- do_execute(code, silent, store_history=True, user_expressions=None, allow_stdin=False, *, cell_meta=None, cell_id=None)[source]#
Execute user code. Must be overridden by subclasses.
- do_history(hist_access_type, output, raw, session=None, start=None, stop=None, n=None, pattern=None, unique=False)[source]#
Override in subclasses to access history.
- do_inspect(code, cursor_pos, detail_level=0, omit_sections=())[source]#
Override in subclasses to allow introspection.
- async do_one_iteration()[source]#
Process a single shell message
Any pending control messages will be flushed as well
Changed in version 5: This is now a coroutine
- do_shutdown(restart)[source]#
Override in subclasses to do things when the frontend shuts down the kernel.
- finish_metadata(parent, metadata, reply_content)[source]#
Finish populating metadata.
Run after completing an execution request.
- get_parent(channel=None)[source]#
Get the parent request associated with a channel.
New in version 6.
- Parameters:
channel (str) – the name of the channel (‘shell’ or ‘control’)
- Returns:
message – the parent message for the most recent request on the channel.
- Return type:
dict
- getpass(prompt='', stream=None)[source]#
Forward getpass to frontends
- Raises:
StdinNotImplementedError if active frontend doesn't support stdin. –
- hold_trait_notifications() Any [source]#
Context manager for bundling trait change notifications and cross validation.
Use this when doing multiple trait assignments (init, config), to avoid race conditions in trait notifiers requesting other trait values. All trait notifications will fire after all values have been assigned.
- classmethod instance(*args: Any, **kwargs: Any) CT [source]#
Returns a global instance of this class.
This method create a new instance if none have previously been created and returns a previously created instance is one already exists.
The arguments and keyword arguments passed to this method are passed on to the
__init__()
method of the class upon instantiation.Examples
Create a singleton class using instance, and retrieve it:
>>> from traitlets.config.configurable import SingletonConfigurable >>> class Foo(SingletonConfigurable): pass >>> foo = Foo.instance() >>> foo == Foo.instance() True
Create a subclass that is retrieved using the base class instance:
>>> class Bar(SingletonConfigurable): pass >>> class Bam(Bar): pass >>> bam = Bam.instance() >>> bam == Bar.instance() True
- observe(handler: Callable[[...], Any], names: Union[Sentinel, str, Iterable[traitlets.utils.sentinel.Sentinel | str]] = traitlets.All, type: traitlets.utils.sentinel.Sentinel | str = 'change') None [source]#
Setup a handler to be called when a trait changes.
This is used to setup dynamic notifications of trait changes.
- Parameters:
handler (callable) – A callable that is called when a trait changes. Its signature should be
handler(change)
, wherechange
is a dictionary. The change dictionary at least holds a ‘type’ key. *type
: the type of notification. Other keys may be passed depending on the value of ‘type’. In the case where type is ‘change’, we also have the following keys: *owner
: the HasTraits instance *old
: the old value of the modified trait attribute *new
: the new value of the modified trait attribute *name
: the name of the modified trait attribute.names (list, str, All) – If names is All, the handler will apply to all traits. If a list of str, handler will apply to all names in the list. If a str, the handler will apply just to that name.
type (str, All (default: 'change')) – The type of notification to filter by. If equal to All, then all notifications are passed to the observe handler.
- on_trait_change(handler: traitlets.traitlets.EventHandler | None = None, name: traitlets.utils.sentinel.Sentinel | str | None = None, remove: bool = False) None [source]#
DEPRECATED: Setup a handler to be called when a trait changes.
This is used to setup dynamic notifications of trait changes.
Static handlers can be created by creating methods on a HasTraits subclass with the naming convention ‘_[traitname]_changed’. Thus, to create static handler for the trait ‘a’, create the method _a_changed(self, name, old, new) (fewer arguments can be used, see below).
If remove is True and handler is not specified, all change handlers for the specified name are uninstalled.
- Parameters:
handler (callable, None) – A callable that is called when a trait changes. Its signature can be handler(), handler(name), handler(name, new), handler(name, old, new), or handler(name, old, new, self).
name (list, str, None) – If None, the handler will apply to all traits. If a list of str, handler will apply to all names in the list. If a str, the handler will apply just to that name.
remove (bool) – If False (the default), then install the handler. If True then unintall it.
- raw_input(prompt='')[source]#
Forward raw_input to frontends
- Raises:
StdinNotImplementedError if active frontend doesn't support stdin. –
- record_ports(ports)[source]#
Record the ports that this kernel is using.
The creator of the Kernel instance must call this methods if they want the
connect_request()
method to return the port numbers.
- send_response(stream, msg_or_type, content=None, ident=None, buffers=None, track=False, header=None, metadata=None, channel=None)[source]#
Send a response to the message we’re currently processing.
This accepts all the parameters of
jupyter_client.session.Session.send()
exceptparent
.This relies on
set_parent()
having been called for the current message.
- set_parent(ident, parent, channel='shell')[source]#
Set the current parent request
Side effects (IOPub messages) and replies are associated with the request that caused them via the parent_header.
The parent identity is used to route input_request messages on the stdin channel.
- set_trait(name: str, value: Any) None [source]#
Forcibly sets trait attribute, including read-only attributes.
- shell_streams: List[t.Any]#
Deprecated shell_streams alias. Use shell_stream
Changed in version 6.0: shell_streams is deprecated. Use shell_stream.
- should_handle(stream, msg, idents)[source]#
Check whether a shell-channel message should be handled
Allows subclasses to prevent handling of certain messages (e.g. aborted requests).
- stop_on_error_timeout#
time (in seconds) to wait for messages to arrive when aborting queued requests after an error.
Requests that arrive within this window after an error will be cancelled.
Increase in the event of unusually slow network causing significant delays, which can manifest as e.g. “Run all” in a notebook aborting some, but not all, messages after an error.
- trait_defaults(*names: str, **metadata: Any) dict[str, Any] | traitlets.utils.sentinel.Sentinel [source]#
Return a trait’s default value or a dictionary of them
Notes
Dynamically generated default values may depend on the current state of the object.
- classmethod trait_events(name: str | None = None) dict[str, traitlets.traitlets.EventHandler] [source]#
Get a
dict
of all the event handlers of this class.- Parameters:
name (str (default: None)) – The name of a trait of this class. If name is
None
then all the event handlers of this class will be returned instead.- Return type:
The event handlers associated with a trait name, or all event handlers.
- trait_has_value(name: str) bool [source]#
Returns True if the specified trait has a value.
This will return false even if
getattr
would return a dynamically generated default value. These default values will be recognized as existing only after they have been generated.Example
class MyClass(HasTraits): i = Int() mc = MyClass() assert not mc.trait_has_value("i") mc.i # generates a default value assert mc.trait_has_value("i")
- trait_metadata(traitname: str, key: str, default: Any = None) Any [source]#
Get metadata values for trait by key.
- trait_values(**metadata: Any) dict[str, Any] [source]#
A
dict
of trait names and their values.The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- Return type:
A
dict
of trait names and their values.
Notes
Trait values are retrieved via
getattr
, any exceptions raised by traits or the operations they may trigger will result in the absence of a trait value in the resultdict
.
- traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a
dict
of all the traits of this class. The dictionary is keyed on the name and the values are the TraitType objects.The TraitTypes returned don’t know anything about the values that the various HasTrait’s instances are holding.
The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- unobserve(handler: Callable[[...], Any], names: Union[Sentinel, str, Iterable[traitlets.utils.sentinel.Sentinel | str]] = traitlets.All, type: traitlets.utils.sentinel.Sentinel | str = 'change') None [source]#
Remove a trait change handler.
This is used to unregister handlers to trait change notifications.
- Parameters:
handler (callable) – The callable called when a trait attribute changes.
names (list, str, All (default: All)) – The names of the traits for which the specified handler should be uninstalled. If names is All, the specified handler is uninstalled from the list of notifiers corresponding to all changes.
type (str or All (default: 'change')) – The type of notification to filter by. If All, the specified handler is uninstalled from the list of notifiers corresponding to all types.
- class panel.io.ipywidget.PanelSessionWebsocket(**kwargs: Any)[source]#
Bases:
SessionWebsocket
- add_traits(**traits: Any) None [source]#
Dynamically add trait attributes to the HasTraits instance.
- buffer_threshold#
Threshold (in bytes) beyond which an object’s buffer should be extracted to avoid pickling.
- check_pid#
Whether to check PID to protect against calls after fork.
This check can be disabled if fork-safety is handled elsewhere.
- classmethod class_config_rst_doc() str [source]#
Generate rST documentation for this class’ config options.
Excludes traits defined on parent classes.
- classmethod class_config_section(classes: Optional[Sequence[type[traitlets.traitlets.HasTraits]]] = None) str [source]#
Get the config section for this class.
- Parameters:
classes (list, optional) – The list of other classes in the config file. Used to reduce redundant information.
- classmethod class_get_help(inst: traitlets.traitlets.HasTraits | None = None) str [source]#
Get the help string for this class in ReST format.
If inst is given, its current trait values will be used in place of class defaults.
- classmethod class_get_trait_help(trait: TraitType[Any, Any], inst: traitlets.traitlets.HasTraits | None = None, helptext: str | None = None) str [source]#
Get the helptext string for a single trait.
- Parameters:
inst – If given, its current trait values will be used in place of the class default.
helptext – If not given, uses the help attribute of the current trait.
- classmethod class_own_trait_events(name: str) dict[str, traitlets.traitlets.EventHandler] [source]#
Get a dict of all event handlers defined on this class, not a parent.
Works like
event_handlers
, except for excluding traits from parents.
- classmethod class_own_traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a dict of all the traitlets defined on this class, not a parent.
Works like class_traits, except for excluding traits from parents.
- classmethod class_print_help(inst: traitlets.traitlets.HasTraits | None = None) None [source]#
Get the help string for a single trait and print it.
- classmethod class_trait_names(**metadata: Any) list[str] [source]#
Get a list of all the names of this class’ traits.
This method is just like the
trait_names()
method, but is unbound.
- classmethod class_traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a
dict
of all the traits of this class. The dictionary is keyed on the name and the values are the TraitType objects.This method is just like the
traits()
method, but is unbound.The TraitTypes returned don’t know anything about the values that the various HasTrait’s instances are holding.
The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- clone() Session [source]#
Create a copy of this Session
Useful when connecting multiple times to a given kernel. This prevents a shared digest_history warning about duplicate digests due to multiple connections to IOPub in the same process.
New in version 5.1.
- copy_threshold#
Threshold (in bytes) beyond which a buffer should be sent without copying.
- property cross_validation_lock: Any#
A contextmanager for running a block with our cross validation lock set to True.
At the end of the block, the lock’s value is restored to its value prior to entering the block.
- debug#
Debug output in the Session
- deserialize(msg_list: list[bytes] | list[zmq.sugar.frame.Frame], content: bool = True, copy: bool = True) dict[str, Any] [source]#
Unserialize a msg_list to a nested message dict.
This is roughly the inverse of serialize. The serialize/deserialize methods work with full message lists, whereas pack/unpack work with the individual message parts in the message list.
- Parameters:
msg_list (list of bytes or Message objects) – The list of message parts of the form [HMAC,p_header,p_parent, p_metadata,p_content,buffer1,buffer2,…].
content (bool (True)) – Whether to unpack the content dict (True), or leave it packed (False).
copy (bool (True)) – Whether msg_list contains bytes (True) or the non-copying Message objects in each place (False).
- Returns:
msg – The nested message dict with top-level keys [header, parent_header, content, buffers]. The buffers are returned as memoryviews.
- Return type:
dict
- digest_history_size#
The maximum number of digests to remember.
The digest history will be culled when it exceeds this value.
- feed_identities(msg_list: list[bytes] | list[zmq.sugar.frame.Frame], copy: bool = True) tuple[list[bytes], list[bytes] | list[zmq.sugar.frame.Frame]] [source]#
Split the identities from the rest of the message.
Feed until DELIM is reached, then return the prefix as idents and remainder as msg_list. This is easily broken by setting an IDENT to DELIM, but that would be silly.
- Parameters:
msg_list (a list of Message or bytes objects) – The message to be split.
copy (bool) – flag determining whether the arguments are bytes or Messages
- Returns:
(idents, msg_list) – idents will always be a list of bytes, each of which is a ZMQ identity. msg_list will be a list of bytes or zmq.Messages of the form [HMAC,p_header,p_parent,p_content,buffer1,buffer2,…] and should be unpackable/unserializable via self.deserialize at this point.
- Return type:
two lists
- hold_trait_notifications() Any [source]#
Context manager for bundling trait change notifications and cross validation.
Use this when doing multiple trait assignments (init, config), to avoid race conditions in trait notifiers requesting other trait values. All trait notifications will fire after all values have been assigned.
- item_threshold#
The maximum number of items for a container to be introspected for custom serialization. Containers larger than this are pickled outright.
- key#
execution key, for signing messages.
- keyfile#
path to file containing execution key.
- metadata#
Metadata dictionary, which serves as the default top-level metadata dict for each message.
- msg(msg_type: str, content: dict | None = None, parent: dict[str, Any] | None = None, header: dict[str, Any] | None = None, metadata: dict[str, Any] | None = None) dict[str, Any] [source]#
Return the nested message dict.
This format is different from what is sent over the wire. The serialize/deserialize methods converts this nested message dict to the wire format, which is a list of message parts.
- observe(handler: Callable[[...], Any], names: Union[Sentinel, str, Iterable[traitlets.utils.sentinel.Sentinel | str]] = traitlets.All, type: traitlets.utils.sentinel.Sentinel | str = 'change') None [source]#
Setup a handler to be called when a trait changes.
This is used to setup dynamic notifications of trait changes.
- Parameters:
handler (callable) – A callable that is called when a trait changes. Its signature should be
handler(change)
, wherechange
is a dictionary. The change dictionary at least holds a ‘type’ key. *type
: the type of notification. Other keys may be passed depending on the value of ‘type’. In the case where type is ‘change’, we also have the following keys: *owner
: the HasTraits instance *old
: the old value of the modified trait attribute *new
: the new value of the modified trait attribute *name
: the name of the modified trait attribute.names (list, str, All) – If names is All, the handler will apply to all traits. If a list of str, handler will apply to all names in the list. If a str, the handler will apply just to that name.
type (str, All (default: 'change')) – The type of notification to filter by. If equal to All, then all notifications are passed to the observe handler.
- on_trait_change(handler: traitlets.traitlets.EventHandler | None = None, name: traitlets.utils.sentinel.Sentinel | str | None = None, remove: bool = False) None [source]#
DEPRECATED: Setup a handler to be called when a trait changes.
This is used to setup dynamic notifications of trait changes.
Static handlers can be created by creating methods on a HasTraits subclass with the naming convention ‘_[traitname]_changed’. Thus, to create static handler for the trait ‘a’, create the method _a_changed(self, name, old, new) (fewer arguments can be used, see below).
If remove is True and handler is not specified, all change handlers for the specified name are uninstalled.
- Parameters:
handler (callable, None) – A callable that is called when a trait changes. Its signature can be handler(), handler(name), handler(name, new), handler(name, old, new), or handler(name, old, new, self).
name (list, str, None) – If None, the handler will apply to all traits. If a list of str, handler will apply to all names in the list. If a str, the handler will apply just to that name.
remove (bool) – If False (the default), then install the handler. If True then unintall it.
- packer#
The name of the packer for serializing messages. Should be one of ‘json’, ‘pickle’, or an import name for a custom callable serializer.
- recv(socket: Socket, mode: int = Flag.DONTWAIT, content: bool = True, copy: bool = True) tuple[list[bytes] | None, dict[str, Any] | None] [source]#
Receive and unpack a message.
- Parameters:
socket (ZMQStream or Socket) – The socket or stream to use in receiving.
- Returns:
[idents] is a list of idents and msg is a nested message dict of same format as self.msg returns.
- Return type:
[idents], msg
- send(stream, msg_type, content=None, parent=None, ident=None, buffers=None, track=False, header=None, metadata=None)[source]#
Build and send a message via stream or socket.
The message format used by this function internally is as follows:
- [ident1,ident2,…,DELIM,HMAC,p_header,p_parent,p_content,
buffer1,buffer2,…]
The serialize/deserialize methods convert the nested message dict into this format.
- Parameters:
stream (zmq.Socket or ZMQStream) – The socket-like object used to send the data.
msg_or_type (str or Message/dict) – Normally, msg_or_type will be a msg_type unless a message is being sent more than once. If a header is supplied, this can be set to None and the msg_type will be pulled from the header.
content (dict or None) – The content of the message (ignored if msg_or_type is a message).
header (dict or None) – The header dict for the message (ignored if msg_to_type is a message).
parent (Message or dict or None) – The parent or parent header describing the parent of this message (ignored if msg_or_type is a message).
ident (bytes or list of bytes) – The zmq.IDENTITY routing path.
metadata (dict or None) – The metadata describing the message
buffers (list or None) – The already-serialized buffers to be appended to the message.
track (bool) – Whether to track. Only for use with Sockets, because ZMQStream objects cannot track messages.
- Returns:
msg – The constructed message.
- Return type:
dict
- send_raw(stream: Socket, msg_list: list, flags: int = 0, copy: bool = True, ident: bytes | list[bytes] | None = None) None [source]#
Send a raw message via ident path.
This method is used to send a already serialized message.
- Parameters:
stream (ZMQStream or Socket) – The ZMQ stream or socket to use for sending the message.
msg_list (list) – The serialized list of messages to send. This only includes the [p_header,p_parent,p_metadata,p_content,buffer1,buffer2,…] portion of the message.
ident (ident or list) – A single ident or a list of idents to use in sending.
- serialize(msg: dict[str, Any], ident: list[bytes] | bytes | None = None) list[bytes] [source]#
Serialize the message components to bytes.
This is roughly the inverse of deserialize. The serialize/deserialize methods work with full message lists, whereas pack/unpack work with the individual message parts in the message list.
- Parameters:
msg (dict or Message) – The next message dict as returned by the self.msg method.
- Returns:
msg_list –
The list of bytes objects to be sent with the format:
[ident1, ident2, ..., DELIM, HMAC, p_header, p_parent, p_metadata, p_content, buffer1, buffer2, ...]
In this list, the
p_*
entities are the packed or serialized versions, so if JSON is used, these are utf8 encoded JSON strings.- Return type:
list
- session#
The UUID identifying this session.
- set_trait(name: str, value: Any) None [source]#
Forcibly sets trait attribute, including read-only attributes.
- sign(msg_list: list) bytes [source]#
Sign a message with HMAC digest. If no auth, return b’’.
- Parameters:
msg_list (list) – The [p_header,p_parent,p_content] part of the message list.
- signature_scheme#
The digest scheme used to construct the message signatures. Must have the form ‘hmac-HASH’.
- trait_defaults(*names: str, **metadata: Any) dict[str, Any] | traitlets.utils.sentinel.Sentinel [source]#
Return a trait’s default value or a dictionary of them
Notes
Dynamically generated default values may depend on the current state of the object.
- classmethod trait_events(name: str | None = None) dict[str, traitlets.traitlets.EventHandler] [source]#
Get a
dict
of all the event handlers of this class.- Parameters:
name (str (default: None)) – The name of a trait of this class. If name is
None
then all the event handlers of this class will be returned instead.- Return type:
The event handlers associated with a trait name, or all event handlers.
- trait_has_value(name: str) bool [source]#
Returns True if the specified trait has a value.
This will return false even if
getattr
would return a dynamically generated default value. These default values will be recognized as existing only after they have been generated.Example
class MyClass(HasTraits): i = Int() mc = MyClass() assert not mc.trait_has_value("i") mc.i # generates a default value assert mc.trait_has_value("i")
- trait_metadata(traitname: str, key: str, default: Any = None) Any [source]#
Get metadata values for trait by key.
- trait_values(**metadata: Any) dict[str, Any] [source]#
A
dict
of trait names and their values.The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- Return type:
A
dict
of trait names and their values.
Notes
Trait values are retrieved via
getattr
, any exceptions raised by traits or the operations they may trigger will result in the absence of a trait value in the resultdict
.
- traits(**metadata: Any) dict[str, traitlets.traitlets.TraitType[Any, Any]] [source]#
Get a
dict
of all the traits of this class. The dictionary is keyed on the name and the values are the TraitType objects.The TraitTypes returned don’t know anything about the values that the various HasTrait’s instances are holding.
The metadata kwargs allow functions to be passed in which filter traits based on metadata values. The functions should take a single value as an argument and return a boolean. If any function returns False, then the trait is not included in the output. If a metadata key doesn’t exist, None will be passed to the function.
- unobserve(handler: Callable[[...], Any], names: Union[Sentinel, str, Iterable[traitlets.utils.sentinel.Sentinel | str]] = traitlets.All, type: traitlets.utils.sentinel.Sentinel | str = 'change') None [source]#
Remove a trait change handler.
This is used to unregister handlers to trait change notifications.
- Parameters:
handler (callable) – The callable called when a trait attribute changes.
names (list, str, All (default: All)) – The names of the traits for which the specified handler should be uninstalled. If names is All, the specified handler is uninstalled from the list of notifiers corresponding to all changes.
type (str or All (default: 'change')) – The type of notification to filter by. If All, the specified handler is uninstalled from the list of notifiers corresponding to all types.
- unobserve_all(name: str | Any = traitlets.All) None [source]#
Remove trait change handlers of any type for the specified name. If name is not specified, removes all trait notifiers.
- unpacker#
The name of the unpacker for unserializing messages. Only used with custom functions for packer.
- username#
Username for the Session. Default is your system username.
- class panel.io.ipywidget.TempComm(target_name: str = 'comm', data: Optional[Dict[str, Any]] = None, metadata: Optional[Dict[str, Any]] = None, buffers: Optional[List[bytes]] = None, comm_id: str | None = None, primary: bool = True, target_module: str | None = None, topic: bytes | None = None, _open_data: Optional[Dict[str, Any]] = None, _close_data: Optional[Dict[str, Any]] = None, **kwargs: Any)[source]#
Bases:
BaseComm
- close(data: Optional[Dict[str, Any]] = None, metadata: Optional[Dict[str, Any]] = None, buffers: Optional[List[bytes]] = None, deleting: bool = False) None [source]#
Close the frontend-side version of this comm
- on_close(callback: Optional[Callable[[Dict[str, Any]], None]]) None [source]#
Register a callback for comm_close
Will be called with the data of the close message.
Call on_close(None) to disable an existing callback.
- on_msg(callback: Optional[Callable[[Dict[str, Any]], None]]) None [source]#
Register a callback for comm_msg
Will be called with the data of any comm_msg messages.
Call on_msg(None) to disable an existing callback.
jupyter_executor
Module#

- class panel.io.jupyter_executor.JupyterServerSession(session_id: ID, document: Document, io_loop: IOLoop | None = None, token: str | None = None)[source]#
Bases:
ServerSession
- classmethod patch(message: msg.patch_doc, connection: ServerConnection) msg.ok [source]#
Handle a PATCH-DOC, return a Future with work to be scheduled.
- classmethod pull(message: msg.pull_doc_req, connection: ServerConnection) msg.pull_doc_reply [source]#
Handle a PULL-DOC, return a Future with work to be scheduled.
- classmethod push(message: msg.push_doc, connection: ServerConnection) msg.ok [source]#
Handle a PUSH-DOC, return a Future with work to be scheduled.
- request_expiration() None [source]#
Used in test suite for now. Forces immediate expiration if no connections.
- subscribe(connection: ServerConnection) None [source]#
This should only be called by
ServerConnection.subscribe_session
or our book-keeping will be broken
- property token: str#
A JWT token to authenticate the session.
- class panel.io.jupyter_executor.PanelExecutor(path, token, root_url)[source]#
Bases:
WSHandler
The PanelExecutor is intended to be run inside a kernel where it runs a Panel application renders the HTML and then establishes a Jupyter Comm channel to communicate with the PanelWSProxy in order to send and receive messages to and from the frontend.
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_origin(origin: str) bool [source]#
Implement a check_origin policy for Tornado to call.
The supplied origin will be compared to the Bokeh server allowlist. If the origin is not allow, an error will be logged and
False
will be returned.- Parameters:
origin (str) – The URL of the connection origin
- Returns:
bool, True if the connection is allowed, False otherwise
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- close(code: Optional[int] = None, reason: Optional[str] = None) None [source]#
Closes this Web Socket.
Once the close handshake is successful the socket will be closed.
code
may be a numeric status code, taken from the values defined in RFC 6455 section 7.4.1.reason
may be a textual message about why the connection is closing. These values are made available to the client, but are not otherwise interpreted by the websocket protocol.Changed in version 4.0: Added the
code
andreason
arguments.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_compression_options() dict[str, Any] | None [source]#
Override to return compression options for the connection.
If this method returns None (the default), compression will be disabled. If it returns a dict (even an empty one), it will be enabled. The contents of the dict may be used to control the following compression options:
compression_level
specifies the compression level.mem_level
specifies the amount of memory used for the internal compression state.These parameters are documented in details here: https://docs.python.org/3.6/library/zlib.html#zlib.compressobj
New in version 4.1.
Changed in version 4.5: Added
compression_level
andmem_level
.
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url()[source]#
Delegates to``get_login_url`` method of the auth provider, or the
login_url
attribute.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- property max_message_size: int#
Maximum allowed message size.
If the remote peer sends a message larger than this, the connection will be closed.
Default is 10MiB.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- async on_message(fragment: str | bytes) None [source]#
Process an individual wire protocol fragment.
The websocket RFC specifies opcodes for distinguishing text frames from binary frames. Tornado passes us either a text or binary string depending on that opcode, we have to look at the type of the fragment to see what we got.
- Parameters:
fragment (unicode or bytes) – wire fragment to process
- ping(data: Union[str, bytes] = b'') None [source]#
Send ping frame to the remote end.
The data argument allows a small amount of data (up to 125 bytes) to be sent as a part of the ping message. Note that not all websocket implementations expose this data to applications.
Consider using the
websocket_ping_interval
application setting instead of sending pings manually.Changed in version 5.1: The data argument is now optional.
- property ping_interval: Optional[float]#
The interval for websocket keep-alive pings.
Set websocket_ping_interval = 0 to disable pings.
- property ping_timeout: Optional[float]#
If no ping is received in this many seconds, close the websocket connection (VPNs, etc. can fail to cleanly close ws connections). Default is max of 3 pings or 30 seconds.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render() Mimebundle [source]#
Renders the application to an IPython.display.HTML object to be served by the PanelJupyterHandler.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- select_subprotocol(subprotocols: list[str]) str | None [source]#
Override to implement subprotocol negotiation.
subprotocols
is a list of strings identifying the subprotocols proposed by the client. This method may be overridden to return one of those strings to select it, orNone
to not select a subprotocol.Failure to select a subprotocol does not automatically abort the connection, although clients may close the connection if none of their proposed subprotocols was selected.
The list may be empty, in which case this method must return None. This method is always called exactly once even if no subprotocols were proposed so that the handler can be advised of this fact.
Changed in version 5.1: Previously, this method was called with a list containing an empty string instead of an empty list if no subprotocols were proposed by the client.
- property selected_subprotocol: Optional[str]#
The subprotocol returned by select_subprotocol.
New in version 5.1.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- async send_message(message: Message[Any]) None [source]#
Send a Bokeh Server protocol message to the connected client.
- Parameters:
message (Message) – a message to send
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_nodelay(value: bool) None [source]#
Set the no-delay flag for this stream.
By default, small messages may be delayed and/or combined to minimize the number of packets sent. This can sometimes cause 200-500ms delays due to the interaction between Nagle’s algorithm and TCP delayed ACKs. To reduce this delay (at the expense of possibly increasing bandwidth usage), call
self.set_nodelay(True)
once the websocket connection is established.See .BaseIOStream.set_nodelay for additional details.
New in version 3.1.
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- async write_message(message: Union[bytes, str, Dict[str, Any]], binary: bool = False, locked: bool = True) None [source]#
Override parent write_message with a version that acquires a write lock before writing.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
jupyter_server_extension
Module#

The Panel jupyter_server_extension implements Jupyter RequestHandlers that allow a Panel application to run inside Jupyter kernels. This allows Panel applications to be served in the kernel (and therefore the environment) they were written for.
The PanelJupyterHandler may be given the path to a notebook, .py file or Lumen .yaml file. It will then attempt to resolve the appropriate kernel based on the kernelspec or a query parameter. Once the kernel has been provisioned the handler will execute a code snippet on the kernel which creates a PanelExecutor. The PanelExecutor creates a bokeh.server.session.ServerSession and connects it to a Jupyter Comm. The PanelJupyterHandler will then serve the result of executor.render().
Once the frontend has rendered the HTML it will send a request to open a WebSocket will be sent to the PanelWSProxy. This in turns forwards any messages it receives via the kernel.shell_channel and the Comm to the PanelExecutor. This way we proxy any WS messages being sent to and from the server to the kernel.
- class panel.io.jupyter_server_extension.PanelBaseHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
JupyterHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- property allow_credentials: bool#
Whether to set Access-Control-Allow-Credentials
- property allow_origin: str#
Normal Access-Control-Allow-Origin
- property allow_origin_pat: str | None#
Regular expression version of allow_origin
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_host() bool [source]#
Check the host header if remote access disallowed.
Returns True if the request should continue, False otherwise.
- check_origin(origin_to_satisfy_tornado: str = '') bool [source]#
Check Origin for cross-site API requests, including websockets
Copied from WebSocket with changes:
allow unspecified host/origin (e.g. scripts)
allow token-authenticated requests
- check_referer() bool [source]#
Check Referer for cross-site requests. Disables requests to certain endpoints with external or missing Referer. If set, allow_origin settings are applied to the Referer to whitelist specific cross-origin sites. Used on GET for api endpoints and /files/ to block cross-site inclusion (XSSI).
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property content_security_policy: str#
The default Content-Security-Policy header
Can be overridden by defining Content-Security-Policy in settings[‘headers’]
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- force_clear_cookie(name: str, path: str = '/', domain: str | None = None) None [source]#
Force a cookie clear.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property jinja_template_vars: dict[str, Any]#
User-supplied values to supply to jinja templates.
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- property log: Logger#
use the Jupyter log by default, falling back on tornado’s logger
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- property logged_in: bool#
Is a user currently logged in?
- property login_available: bool#
May a user proceed to log in?
This returns True if login capability is available, irrespective of whether the user is already logged in or not.
- property login_handler: Any#
Return the login handler for this application, if any.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_attachment_header(filename: str) None [source]#
Set Content-Disposition: attachment header
As a method to ensure handling of filename encoding
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_cors_headers() None [source]#
Add CORS headers, if defined
Now that current_user is async (jupyter-server 2.0), must be called at the end of prepare(), instead of in set_default_headers.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- skip_check_origin() bool [source]#
Ask my login_handler if I should skip the origin_check
For example: in the default LoginHandler, if a request is token-authenticated, origin checking should be skipped.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- property token: str | None#
Return the login token for this application, if any.
- property token_authenticated: bool#
Have I been authenticated with a token?
- property version_hash: str#
The version hash to use for cache hints for static files
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.jupyter_server_extension.PanelJupyterHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
PanelBaseHandler
The PanelJupyterHandler expects to be given a path to a notebook, .py file or Lumen .yaml file. Based on the kernelspec in the notebook or the kernel query parameter it will then provision a Jupyter kernel to run the Panel application in.
Once the kernel is launched it will instantiate a PanelExecutor inside the kernel and serve the HTML returned by it. If successful it will store the kernel and comm_id on panel.state.
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- property allow_credentials: bool#
Whether to set Access-Control-Allow-Credentials
- property allow_origin: str#
Normal Access-Control-Allow-Origin
- property allow_origin_pat: str | None#
Regular expression version of allow_origin
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_host() bool [source]#
Check the host header if remote access disallowed.
Returns True if the request should continue, False otherwise.
- check_origin(origin_to_satisfy_tornado: str = '') bool [source]#
Check Origin for cross-site API requests, including websockets
Copied from WebSocket with changes:
allow unspecified host/origin (e.g. scripts)
allow token-authenticated requests
- check_referer() bool [source]#
Check Referer for cross-site requests. Disables requests to certain endpoints with external or missing Referer. If set, allow_origin settings are applied to the Referer to whitelist specific cross-origin sites. Used on GET for api endpoints and /files/ to block cross-site inclusion (XSSI).
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property content_security_policy: str#
The default Content-Security-Policy header
Can be overridden by defining Content-Security-Policy in settings[‘headers’]
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- force_clear_cookie(name: str, path: str = '/', domain: str | None = None) None [source]#
Force a cookie clear.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property jinja_template_vars: dict[str, Any]#
User-supplied values to supply to jinja templates.
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- property log: Logger#
use the Jupyter log by default, falling back on tornado’s logger
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- property logged_in: bool#
Is a user currently logged in?
- property login_available: bool#
May a user proceed to log in?
This returns True if login capability is available, irrespective of whether the user is already logged in or not.
- property login_handler: Any#
Return the login handler for this application, if any.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_attachment_header(filename: str) None [source]#
Set Content-Disposition: attachment header
As a method to ensure handling of filename encoding
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_cors_headers() None [source]#
Add CORS headers, if defined
Now that current_user is async (jupyter-server 2.0), must be called at the end of prepare(), instead of in set_default_headers.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- skip_check_origin() bool [source]#
Ask my login_handler if I should skip the origin_check
For example: in the default LoginHandler, if a request is token-authenticated, origin checking should be skipped.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- property token: str | None#
Return the login token for this application, if any.
- property token_authenticated: bool#
Have I been authenticated with a token?
- property version_hash: str#
The version hash to use for cache hints for static files
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.jupyter_server_extension.PanelLayoutHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
PanelBaseHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- property allow_credentials: bool#
Whether to set Access-Control-Allow-Credentials
- property allow_origin: str#
Normal Access-Control-Allow-Origin
- property allow_origin_pat: str | None#
Regular expression version of allow_origin
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_host() bool [source]#
Check the host header if remote access disallowed.
Returns True if the request should continue, False otherwise.
- check_origin(origin_to_satisfy_tornado: str = '') bool [source]#
Check Origin for cross-site API requests, including websockets
Copied from WebSocket with changes:
allow unspecified host/origin (e.g. scripts)
allow token-authenticated requests
- check_referer() bool [source]#
Check Referer for cross-site requests. Disables requests to certain endpoints with external or missing Referer. If set, allow_origin settings are applied to the Referer to whitelist specific cross-origin sites. Used on GET for api endpoints and /files/ to block cross-site inclusion (XSSI).
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property content_security_policy: str#
The default Content-Security-Policy header
Can be overridden by defining Content-Security-Policy in settings[‘headers’]
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- force_clear_cookie(name: str, path: str = '/', domain: str | None = None) None [source]#
Force a cookie clear.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property jinja_template_vars: dict[str, Any]#
User-supplied values to supply to jinja templates.
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- property log: Logger#
use the Jupyter log by default, falling back on tornado’s logger
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- property logged_in: bool#
Is a user currently logged in?
- property login_available: bool#
May a user proceed to log in?
This returns True if login capability is available, irrespective of whether the user is already logged in or not.
- property login_handler: Any#
Return the login handler for this application, if any.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_attachment_header(filename: str) None [source]#
Set Content-Disposition: attachment header
As a method to ensure handling of filename encoding
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_cors_headers() None [source]#
Add CORS headers, if defined
Now that current_user is async (jupyter-server 2.0), must be called at the end of prepare(), instead of in set_default_headers.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- skip_check_origin() bool [source]#
Ask my login_handler if I should skip the origin_check
For example: in the default LoginHandler, if a request is token-authenticated, origin checking should be skipped.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- property token: str | None#
Return the login token for this application, if any.
- property token_authenticated: bool#
Have I been authenticated with a token?
- property version_hash: str#
The version hash to use for cache hints for static files
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.jupyter_server_extension.PanelWSProxy(tornado_app, *args, **kw)[source]#
Bases:
WSHandler
,JupyterHandler
The PanelWSProxy serves as a proxy between the frontend and the Jupyter kernel that is running the Panel application. It send and receives Bokeh protocol messages via a Jupyter Comm.
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- property allow_credentials: bool#
Whether to set Access-Control-Allow-Credentials
- property allow_origin: str#
Normal Access-Control-Allow-Origin
- property allow_origin_pat: str | None#
Regular expression version of allow_origin
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_host() bool [source]#
Check the host header if remote access disallowed.
Returns True if the request should continue, False otherwise.
- check_origin(origin: str) bool [source]#
Implement a check_origin policy for Tornado to call.
The supplied origin will be compared to the Bokeh server allowlist. If the origin is not allow, an error will be logged and
False
will be returned.- Parameters:
origin (str) – The URL of the connection origin
- Returns:
bool, True if the connection is allowed, False otherwise
- check_referer() bool [source]#
Check Referer for cross-site requests. Disables requests to certain endpoints with external or missing Referer. If set, allow_origin settings are applied to the Referer to whitelist specific cross-origin sites. Used on GET for api endpoints and /files/ to block cross-site inclusion (XSSI).
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- close(code: Optional[int] = None, reason: Optional[str] = None) None [source]#
Closes this Web Socket.
Once the close handshake is successful the socket will be closed.
code
may be a numeric status code, taken from the values defined in RFC 6455 section 7.4.1.reason
may be a textual message about why the connection is closing. These values are made available to the client, but are not otherwise interpreted by the websocket protocol.Changed in version 4.0: Added the
code
andreason
arguments.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property content_security_policy: str#
The default Content-Security-Policy header
Can be overridden by defining Content-Security-Policy in settings[‘headers’]
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- force_clear_cookie(name: str, path: str = '/', domain: str | None = None) None [source]#
Force a cookie clear.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_compression_options() dict[str, Any] | None [source]#
Override to return compression options for the connection.
If this method returns None (the default), compression will be disabled. If it returns a dict (even an empty one), it will be enabled. The contents of the dict may be used to control the following compression options:
compression_level
specifies the compression level.mem_level
specifies the amount of memory used for the internal compression state.These parameters are documented in details here: https://docs.python.org/3.6/library/zlib.html#zlib.compressobj
New in version 4.1.
Changed in version 4.5: Added
compression_level
andmem_level
.
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url()[source]#
Delegates to``get_login_url`` method of the auth provider, or the
login_url
attribute.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property jinja_template_vars: dict[str, Any]#
User-supplied values to supply to jinja templates.
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- property log: Logger#
use the Jupyter log by default, falling back on tornado’s logger
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- property logged_in: bool#
Is a user currently logged in?
- property login_available: bool#
May a user proceed to log in?
This returns True if login capability is available, irrespective of whether the user is already logged in or not.
- property login_handler: Any#
Return the login handler for this application, if any.
- property max_message_size: int#
Maximum allowed message size.
If the remote peer sends a message larger than this, the connection will be closed.
Default is 10MiB.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- async on_message(fragment: str | bytes) None [source]#
Process an individual wire protocol fragment.
The websocket RFC specifies opcodes for distinguishing text frames from binary frames. Tornado passes us either a text or binary string depending on that opcode, we have to look at the type of the fragment to see what we got.
- Parameters:
fragment (unicode or bytes) – wire fragment to process
- ping(data: Union[str, bytes] = b'') None [source]#
Send ping frame to the remote end.
The data argument allows a small amount of data (up to 125 bytes) to be sent as a part of the ping message. Note that not all websocket implementations expose this data to applications.
Consider using the
websocket_ping_interval
application setting instead of sending pings manually.Changed in version 5.1: The data argument is now optional.
- property ping_interval: Optional[float]#
The interval for websocket keep-alive pings.
Set websocket_ping_interval = 0 to disable pings.
- property ping_timeout: Optional[float]#
If no ping is received in this many seconds, close the websocket connection (VPNs, etc. can fail to cleanly close ws connections). Default is max of 3 pings or 30 seconds.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- select_subprotocol(subprotocols: list[str]) str | None [source]#
Override to implement subprotocol negotiation.
subprotocols
is a list of strings identifying the subprotocols proposed by the client. This method may be overridden to return one of those strings to select it, orNone
to not select a subprotocol.Failure to select a subprotocol does not automatically abort the connection, although clients may close the connection if none of their proposed subprotocols was selected.
The list may be empty, in which case this method must return None. This method is always called exactly once even if no subprotocols were proposed so that the handler can be advised of this fact.
Changed in version 5.1: Previously, this method was called with a list containing an empty string instead of an empty list if no subprotocols were proposed by the client.
- property selected_subprotocol: Optional[str]#
The subprotocol returned by select_subprotocol.
New in version 5.1.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- async send_message(message: Message[Any]) None [source]#
Send a Bokeh Server protocol message to the connected client.
- Parameters:
message (Message) – a message to send
- set_attachment_header(filename: str) None [source]#
Set Content-Disposition: attachment header
As a method to ensure handling of filename encoding
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_cors_headers() None [source]#
Add CORS headers, if defined
Now that current_user is async (jupyter-server 2.0), must be called at the end of prepare(), instead of in set_default_headers.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_nodelay(value: bool) None [source]#
Set the no-delay flag for this stream.
By default, small messages may be delayed and/or combined to minimize the number of packets sent. This can sometimes cause 200-500ms delays due to the interaction between Nagle’s algorithm and TCP delayed ACKs. To reduce this delay (at the expense of possibly increasing bandwidth usage), call
self.set_nodelay(True)
once the websocket connection is established.See .BaseIOStream.set_nodelay for additional details.
New in version 3.1.
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- skip_check_origin() bool [source]#
Ask my login_handler if I should skip the origin_check
For example: in the default LoginHandler, if a request is token-authenticated, origin checking should be skipped.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- property token: str | None#
Return the login token for this application, if any.
- property token_authenticated: bool#
Have I been authenticated with a token?
- property version_hash: str#
The version hash to use for cache hints for static files
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- async write_message(message: bytes | str | dict[str, Any], binary: bool = False, locked: bool = True) None [source]#
Override parent write_message with a version that acquires a write lock before writing.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- async panel.io.jupyter_server_extension.ensure_async(obj: Union[Awaitable, Any]) Any [source]#
Convert a non-awaitable object to a coroutine if needed, and await it if it was not already awaited.
- panel.io.jupyter_server_extension.generate_executor(path: str, token: str, root_url: str) str [source]#
Generates the code to instantiate a PanelExecutor that is to be be run on the kernel to start a server session.
- Parameters:
path (str) – The path of the Panel application to execute.
token (str) – The Bokeh JWT token containing the session_id, request arguments, headers and cookies.
root_url (str) – The root_url the server is running on.
- Return type:
The code to be executed inside the kernel.
liveness
Module#

- class panel.io.liveness.LivenessHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
RequestHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_current_user() Any [source]#
Override to determine the current user from, e.g., a cookie.
This method may not be a coroutine.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- prepare() Optional[Awaitable[None]] [source]#
Called at the beginning of a request before get/post/etc.
Override this method to perform common initialization regardless of the request method.
Asynchronous support: Use
async def
or decorate this method with .gen.coroutine to make it asynchronous. If this method returns anAwaitable
execution will not proceed until theAwaitable
is done.New in version 3.1: Asynchronous support.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
loading
Module#
This module contains functionality to make any Panel component look like it is loading and disabled.
- panel.io.loading.start_loading_spinner(*objects)[source]#
Changes the appearance of the specified panel objects to indicate that they are loading.
This is done by
adding a small spinner on top
graying out the panel
disabling the panel
and changing the mouse cursor to a spinner when hovering over the panel
- Parameters:
objects (tuple) – The panels to add the loading indicator to.
location
Module#

Defines the Location widget which allows changing the href of the window.
- class panel.io.location.Location(*, hash, pathname, reload, search, name)[source]#
Bases:
Syncable
The Location component can be made available in a server context to provide read and write access to the URL components in the browser.
href
= param.String(allow_refs=False, constant=True, default=’’, label=’Href’, nested_refs=False, readonly=True, rx=<param.reactive.reactive_ops object at 0x147f96650>)The full url, e.g. ‘https://localhost:80?color=blue#interact’
hostname
= param.String(allow_refs=False, constant=True, default=’’, label=’Hostname’, nested_refs=False, readonly=True, rx=<param.reactive.reactive_ops object at 0x147f97450>)hostname in window.location e.g. ‘panel.holoviz.org’
pathname
= param.String(allow_refs=False, default=’’, label=’Pathname’, nested_refs=False, regex=’^$|[\/]|srcdoc$’, rx=<param.reactive.reactive_ops object at 0x147de29d0>)pathname in window.location e.g. ‘/user_guide/Interact.html’
protocol
= param.String(allow_refs=False, constant=True, default=’’, label=’Protocol’, nested_refs=False, readonly=True, rx=<param.reactive.reactive_ops object at 0x147f96590>)protocol in window.location e.g. ‘http:’ or ‘https:’
port
= param.String(allow_refs=False, constant=True, default=’’, label=’Port’, nested_refs=False, readonly=True, rx=<param.reactive.reactive_ops object at 0x147f94d90>)port in window.location e.g. ‘80’
search
= param.String(allow_refs=False, default=’’, label=’Search’, nested_refs=False, regex=’^$|\?’, rx=<param.reactive.reactive_ops object at 0x147f96410>)search in window.location e.g. ‘?color=blue’
hash
= param.String(allow_refs=False, default=’’, label=’Hash’, nested_refs=False, regex=’^$|#’, rx=<param.reactive.reactive_ops object at 0x147f97450>)hash in window.location e.g. ‘#interact’
reload
= param.Boolean(allow_refs=False, default=False, label=’Reload’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x147f973d0>)Reload the page when the location is updated. For multipage apps this should be set to True, For single page apps this should be set to False
- get_root(doc: Optional[Document] = None, comm: Optional[Comm] = None, preprocess: bool = True) Model [source]#
Returns the root model and applies pre-processing hooks
- Parameters:
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
comm (pyviz_comms.Comm) – Optional pyviz_comms when working in notebook
preprocess (boolean (default=True)) – Whether to run preprocessing hooks
- Return type:
Returns the bokeh model corresponding to this panel object
- sync(parameterized: Parameterized, parameters: Optional[Union[List[str], Dict[str, str]]] = None, on_error: Optional[Callable[[Dict[str, Any]], None]] = None) None [source]#
Syncs the parameters of a Parameterized object with the query parameters in the URL. If no parameters are supplied all parameters except the name are synced.
- Parameters:
(param.Parameterized) (parameterized) – The Parameterized object to sync query parameters with
dict) (parameters (list or) – A list or dictionary specifying parameters to sync. If a dictionary is supplied it should define a mapping from the Parameterized’s parameters to the names of the query parameters.
on_error ((callable):) – Callback when syncing Parameterized with URL parameters fails. The callback is passed a dictionary of parameter values, which could not be applied.
- unsync(parameterized: Parameterized, parameters: Optional[List[str]] = None) None [source]#
Unsyncs the parameters of the Parameterized with the query params in the URL. If no parameters are supplied all parameters except the name are unsynced.
- Parameters:
(param.Parameterized) (parameterized) – The Parameterized object to unsync query parameters with
(list) (parameters) – A list of parameters to unsync.
logging
Module#
mime_render
Module#

Utilities for executing Python code and rendering the resulting output using a similar MIME-type based rendering system as implemented by IPython.
Attempts to limit the actual MIME types that have to be rendered on to a minimum simplifying frontend implementation:
application/bokeh: Model JSON representation
text/plain: HTML escaped string output
text/html: HTML code to insert into the DOM
- class panel.io.mime_render.WriteCallbackStream(on_write=None, escape=True)[source]#
Bases:
StringIO
- close()#
Close the IO object.
Attempting any further operation after the object is closed will raise a ValueError.
This method has no effect if the file is already closed.
- detach()#
Separate the underlying buffer from the TextIOBase and return it.
After the underlying buffer has been detached, the TextIO is in an unusable state.
- encoding#
Encoding of the text stream.
Subclasses should override.
- errors#
The error setting of the decoder or encoder.
Subclasses should override.
- fileno()#
Returns underlying file descriptor if one exists.
OSError is raised if the IO object does not use a file descriptor.
- flush()#
Flush write buffers, if applicable.
This is not implemented for read-only and non-blocking streams.
- getvalue()#
Retrieve the entire contents of the object.
- isatty()#
Return whether this is an ‘interactive’ stream.
Return False if it can’t be determined.
- newlines#
- read(size=-1, /)#
Read at most size characters, returned as a string.
If the argument is negative or omitted, read until EOF is reached. Return an empty string at EOF.
- readable()#
Returns True if the IO object can be read.
- readline(size=-1, /)#
Read until newline or EOF.
Returns an empty string if EOF is hit immediately.
- readlines(hint=-1, /)#
Return a list of lines from the stream.
hint can be specified to control the number of lines read: no more lines will be read if the total size (in bytes/characters) of all lines so far exceeds hint.
- seek(pos, whence=0, /)#
Change stream position.
- Seek to character offset pos relative to position indicated by whence:
0 Start of stream (the default). pos should be >= 0; 1 Current position - pos must be 0; 2 End of stream - pos must be 0.
Returns the new absolute position.
- seekable()#
Returns True if the IO object can be seeked.
- tell()#
Tell the current file position.
- truncate(pos=None, /)#
Truncate size to pos.
The pos argument defaults to the current file position, as returned by tell(). The current file position is unchanged. Returns the new absolute position.
- writable()#
Returns True if the IO object can be written.
- write(s)[source]#
Write string to file.
Returns the number of characters written, which is always equal to the length of the string.
- writelines(lines, /)#
Write a list of lines to stream.
Line separators are not added, so it is usual for each of the lines provided to have a line separator at the end.
- panel.io.mime_render.exec_with_return(code: str, global_context: Dict[str, Any] = None, stdout: Any = None, stderr: Any = None) Any [source]#
Executes a code snippet and returns the resulting output of the last line.
- Parameters:
code (str) – The code to execute
global_context (Dict[str, Any]) – The globals to inject into the execution context.
stdout (io.StringIO) – The stream to redirect stdout to.
stderr (io.StringIO) – The stream to redirect stderr to.
- Return type:
The return value of the executed code.
- panel.io.mime_render.find_requirements(code: str) List[str] [source]#
Finds the packages required in a string of code.
- Parameters:
code (str) – the Python code to run.
- Returns:
A list of package names that are to be installed for the code to be able to run.
- Return type:
List[str]
Examples
>>> code = "import numpy as np; import scipy.stats" >>> find_imports(code) ['numpy', 'scipy']
model
Module#

Utilities for manipulating bokeh models.
- panel.io.model.add_to_doc(obj: Model, doc: Document, hold: bool = False, skip: Optional[Set[Model]] = None)[source]#
Adds a model to the supplied Document removing it from any existing Documents.
- panel.io.model.bokeh_repr(obj: Model, depth: int = 0, ignored: Optional[Iterable[str]] = None) str [source]#
Returns a string repr for a bokeh model, useful for recreating panel objects using pure bokeh.
- class panel.io.model.comparable_array[source]#
Bases:
ndarray
Array subclass that allows comparisons.
- T#
View of the transposed array.
Same as
self.transpose()
.Examples
>>> a = np.array([[1, 2], [3, 4]]) >>> a array([[1, 2], [3, 4]]) >>> a.T array([[1, 3], [2, 4]])
>>> a = np.array([1, 2, 3, 4]) >>> a array([1, 2, 3, 4]) >>> a.T array([1, 2, 3, 4])
See also
- all(axis=None, out=None, keepdims=False, *, where=True)#
Returns True if all elements evaluate to True.
Refer to numpy.all for full documentation.
See also
numpy.all
equivalent function
- any(axis=None, out=None, keepdims=False, *, where=True)#
Returns True if any of the elements of a evaluate to True.
Refer to numpy.any for full documentation.
See also
numpy.any
equivalent function
- argmax(axis=None, out=None, *, keepdims=False)#
Return indices of the maximum values along the given axis.
Refer to numpy.argmax for full documentation.
See also
numpy.argmax
equivalent function
- argmin(axis=None, out=None, *, keepdims=False)#
Return indices of the minimum values along the given axis.
Refer to numpy.argmin for detailed documentation.
See also
numpy.argmin
equivalent function
- argpartition(kth, axis=-1, kind='introselect', order=None)#
Returns the indices that would partition this array.
Refer to numpy.argpartition for full documentation.
New in version 1.8.0.
See also
numpy.argpartition
equivalent function
- argsort(axis=-1, kind=None, order=None)#
Returns the indices that would sort this array.
Refer to numpy.argsort for full documentation.
See also
numpy.argsort
equivalent function
- astype(dtype, order='K', casting='unsafe', subok=True, copy=True)#
Copy of the array, cast to a specified type.
- Parameters:
dtype (str or dtype) – Typecode or data-type to which the array is cast.
order ({'C', 'F', 'A', 'K'}, optional) – Controls the memory layout order of the result. ‘C’ means C order, ‘F’ means Fortran order, ‘A’ means ‘F’ order if all the arrays are Fortran contiguous, ‘C’ order otherwise, and ‘K’ means as close to the order the array elements appear in memory as possible. Default is ‘K’.
casting ({'no', 'equiv', 'safe', 'same_kind', 'unsafe'}, optional) –
Controls what kind of data casting may occur. Defaults to ‘unsafe’ for backwards compatibility.
’no’ means the data types should not be cast at all.
’equiv’ means only byte-order changes are allowed.
’safe’ means only casts which can preserve values are allowed.
’same_kind’ means only safe casts or casts within a kind, like float64 to float32, are allowed.
’unsafe’ means any data conversions may be done.
subok (bool, optional) – If True, then sub-classes will be passed-through (default), otherwise the returned array will be forced to be a base-class array.
copy (bool, optional) – By default, astype always returns a newly allocated array. If this is set to false, and the dtype, order, and subok requirements are satisfied, the input array is returned instead of a copy.
- Returns:
arr_t – Unless copy is False and the other conditions for returning the input array are satisfied (see description for copy input parameter), arr_t is a new array of the same shape as the input array, with dtype, order given by dtype, order.
- Return type:
ndarray
Notes
Changed in version 1.17.0: Casting between a simple data type and a structured one is possible only for “unsafe” casting. Casting to multiple fields is allowed, but casting from multiple fields is not.
Changed in version 1.9.0: Casting from numeric to string types in ‘safe’ casting mode requires that the string dtype length is long enough to store the max integer/float value converted.
- Raises:
ComplexWarning – When casting from complex to float or int. To avoid this, one should use
a.real.astype(t)
.
Examples
>>> x = np.array([1, 2, 2.5]) >>> x array([1. , 2. , 2.5])
>>> x.astype(int) array([1, 2, 2])
- base#
Base object if memory is from some other object.
Examples
The base of an array that owns its memory is None:
>>> x = np.array([1,2,3,4]) >>> x.base is None True
Slicing creates a view, whose memory is shared with x:
>>> y = x[2:] >>> y.base is x True
- byteswap(inplace=False)#
Swap the bytes of the array elements
Toggle between low-endian and big-endian data representation by returning a byteswapped array, optionally swapped in-place. Arrays of byte-strings are not swapped. The real and imaginary parts of a complex number are swapped individually.
- Parameters:
inplace (bool, optional) – If
True
, swap bytes in-place, default isFalse
.- Returns:
out – The byteswapped array. If inplace is
True
, this is a view to self.- Return type:
ndarray
Examples
>>> A = np.array([1, 256, 8755], dtype=np.int16) >>> list(map(hex, A)) ['0x1', '0x100', '0x2233'] >>> A.byteswap(inplace=True) array([ 256, 1, 13090], dtype=int16) >>> list(map(hex, A)) ['0x100', '0x1', '0x3322']
Arrays of byte-strings are not swapped
>>> A = np.array([b'ceg', b'fac']) >>> A.byteswap() array([b'ceg', b'fac'], dtype='|S3')
A.newbyteorder().byteswap()
produces an array with the same valuesbut different representation in memory
>>> A = np.array([1, 2, 3]) >>> A.view(np.uint8) array([1, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0], dtype=uint8) >>> A.newbyteorder().byteswap(inplace=True) array([1, 2, 3]) >>> A.view(np.uint8) array([0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 3], dtype=uint8)
- choose(choices, out=None, mode='raise')#
Use an index array to construct a new array from a set of choices.
Refer to numpy.choose for full documentation.
See also
numpy.choose
equivalent function
- clip(min=None, max=None, out=None, **kwargs)#
Return an array whose values are limited to
[min, max]
. One of max or min must be given.Refer to numpy.clip for full documentation.
See also
numpy.clip
equivalent function
- compress(condition, axis=None, out=None)#
Return selected slices of this array along given axis.
Refer to numpy.compress for full documentation.
See also
numpy.compress
equivalent function
- conj()#
Complex-conjugate all elements.
Refer to numpy.conjugate for full documentation.
See also
numpy.conjugate
equivalent function
- conjugate()#
Return the complex conjugate, element-wise.
Refer to numpy.conjugate for full documentation.
See also
numpy.conjugate
equivalent function
- copy(order='C')#
Return a copy of the array.
- Parameters:
order ({'C', 'F', 'A', 'K'}, optional) – Controls the memory layout of the copy. ‘C’ means C-order, ‘F’ means F-order, ‘A’ means ‘F’ if a is Fortran contiguous, ‘C’ otherwise. ‘K’ means match the layout of a as closely as possible. (Note that this function and
numpy.copy()
are very similar but have different default values for their order= arguments, and this function always passes sub-classes through.)
See also
numpy.copy
Similar function with different default behavior
numpy.copyto
Notes
This function is the preferred method for creating an array copy. The function
numpy.copy()
is similar, but it defaults to using order ‘K’, and will not pass sub-classes through by default.Examples
>>> x = np.array([[1,2,3],[4,5,6]], order='F')
>>> y = x.copy()
>>> x.fill(0)
>>> x array([[0, 0, 0], [0, 0, 0]])
>>> y array([[1, 2, 3], [4, 5, 6]])
>>> y.flags['C_CONTIGUOUS'] True
- ctypes#
An object to simplify the interaction of the array with the ctypes module.
This attribute creates an object that makes it easier to use arrays when calling shared libraries with the ctypes module. The returned object has, among others, data, shape, and strides attributes (see Notes below) which themselves return ctypes objects that can be used as arguments to a shared library.
- Parameters:
None –
- Returns:
c – Possessing attributes data, shape, strides, etc.
- Return type:
Python object
See also
numpy.ctypeslib
Notes
Below are the public attributes of this object which were documented in “Guide to NumPy” (we have omitted undocumented public attributes, as well as documented private attributes):
- _ctypes.data
A pointer to the memory area of the array as a Python integer. This memory area may contain data that is not aligned, or not in correct byte-order. The memory area may not even be writeable. The array flags and data-type of this array should be respected when passing this attribute to arbitrary C-code to avoid trouble that can include Python crashing. User Beware! The value of this attribute is exactly the same as
self._array_interface_['data'][0]
.Note that unlike
data_as
, a reference will not be kept to the array: code likectypes.c_void_p((a + b).ctypes.data)
will result in a pointer to a deallocated array, and should be spelt(a + b).ctypes.data_as(ctypes.c_void_p)
- _ctypes.shape
A ctypes array of length self.ndim where the basetype is the C-integer corresponding to
dtype('p')
on this platform (see ~numpy.ctypeslib.c_intp). This base-type could be ctypes.c_int, ctypes.c_long, or ctypes.c_longlong depending on the platform. The ctypes array contains the shape of the underlying array.- Type:
(c_intp*self.ndim)
- _ctypes.strides
A ctypes array of length self.ndim where the basetype is the same as for the shape attribute. This ctypes array contains the strides information from the underlying array. This strides information is important for showing how many bytes must be jumped to get to the next element in the array.
- Type:
(c_intp*self.ndim)
- _ctypes.data_as(obj)
Return the data pointer cast to a particular c-types object. For example, calling
self._as_parameter_
is equivalent toself.data_as(ctypes.c_void_p)
. Perhaps you want to use the data as a pointer to a ctypes array of floating-point data:self.data_as(ctypes.POINTER(ctypes.c_double))
.The returned pointer will keep a reference to the array.
- _ctypes.shape_as(obj)
Return the shape tuple as an array of some other c-types type. For example:
self.shape_as(ctypes.c_short)
.
- _ctypes.strides_as(obj)
Return the strides tuple as an array of some other c-types type. For example:
self.strides_as(ctypes.c_longlong)
.
If the ctypes module is not available, then the ctypes attribute of array objects still returns something useful, but ctypes objects are not returned and errors may be raised instead. In particular, the object will still have the
as_parameter
attribute which will return an integer equal to the data attribute.Examples
>>> import ctypes >>> x = np.array([[0, 1], [2, 3]], dtype=np.int32) >>> x array([[0, 1], [2, 3]], dtype=int32) >>> x.ctypes.data 31962608 # may vary >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint32)) <__main__.LP_c_uint object at 0x7ff2fc1fc200> # may vary >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint32)).contents c_uint(0) >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint64)).contents c_ulong(4294967296) >>> x.ctypes.shape <numpy.core._internal.c_long_Array_2 object at 0x7ff2fc1fce60> # may vary >>> x.ctypes.strides <numpy.core._internal.c_long_Array_2 object at 0x7ff2fc1ff320> # may vary
- cumprod(axis=None, dtype=None, out=None)#
Return the cumulative product of the elements along the given axis.
Refer to numpy.cumprod for full documentation.
See also
numpy.cumprod
equivalent function
- cumsum(axis=None, dtype=None, out=None)#
Return the cumulative sum of the elements along the given axis.
Refer to numpy.cumsum for full documentation.
See also
numpy.cumsum
equivalent function
- data#
Python buffer object pointing to the start of the array’s data.
- diagonal(offset=0, axis1=0, axis2=1)#
Return specified diagonals. In NumPy 1.9 the returned array is a read-only view instead of a copy as in previous NumPy versions. In a future version the read-only restriction will be removed.
Refer to
numpy.diagonal()
for full documentation.See also
numpy.diagonal
equivalent function
- dtype#
Data-type of the array’s elements.
Warning
Setting
arr.dtype
is discouraged and may be deprecated in the future. Setting will replace thedtype
without modifying the memory (see also ndarray.view and ndarray.astype).- Parameters:
None –
- Returns:
d
- Return type:
numpy dtype object
See also
ndarray.astype
Cast the values contained in the array to a new data-type.
ndarray.view
Create a view of the same data but a different data-type.
numpy.dtype
Examples
>>> x array([[0, 1], [2, 3]]) >>> x.dtype dtype('int32') >>> type(x.dtype) <type 'numpy.dtype'>
- dump(file)#
Dump a pickle of the array to the specified file. The array can be read back with pickle.load or numpy.load.
- Parameters:
file (str or Path) –
A string naming the dump file.
Changed in version 1.17.0: pathlib.Path objects are now accepted.
- dumps()#
Returns the pickle of the array as a string. pickle.loads will convert the string back to an array.
- Parameters:
None –
- fill(value)#
Fill the array with a scalar value.
- Parameters:
value (scalar) – All elements of a will be assigned this value.
Examples
>>> a = np.array([1, 2]) >>> a.fill(0) >>> a array([0, 0]) >>> a = np.empty(2) >>> a.fill(1) >>> a array([1., 1.])
Fill expects a scalar value and always behaves the same as assigning to a single array element. The following is a rare example where this distinction is important:
>>> a = np.array([None, None], dtype=object) >>> a[0] = np.array(3) >>> a array([array(3), None], dtype=object) >>> a.fill(np.array(3)) >>> a array([array(3), array(3)], dtype=object)
Where other forms of assignments will unpack the array being assigned:
>>> a[...] = np.array(3) >>> a array([3, 3], dtype=object)
- flags#
Information about the memory layout of the array.
- C_CONTIGUOUS(C)#
The data is in a single, C-style contiguous segment.
- F_CONTIGUOUS(F)#
The data is in a single, Fortran-style contiguous segment.
- OWNDATA(O)#
The array owns the memory it uses or borrows it from another object.
- WRITEABLE(W)#
The data area can be written to. Setting this to False locks the data, making it read-only. A view (slice, etc.) inherits WRITEABLE from its base array at creation time, but a view of a writeable array may be subsequently locked while the base array remains writeable. (The opposite is not true, in that a view of a locked array may not be made writeable. However, currently, locking a base object does not lock any views that already reference it, so under that circumstance it is possible to alter the contents of a locked array via a previously created writeable view onto it.) Attempting to change a non-writeable array raises a RuntimeError exception.
- ALIGNED(A)#
The data and all elements are aligned appropriately for the hardware.
- WRITEBACKIFCOPY(X)#
This array is a copy of some other array. The C-API function PyArray_ResolveWritebackIfCopy must be called before deallocating to the base array will be updated with the contents of this array.
- FNC#
F_CONTIGUOUS and not C_CONTIGUOUS.
- FORC#
F_CONTIGUOUS or C_CONTIGUOUS (one-segment test).
- BEHAVED(B)#
ALIGNED and WRITEABLE.
- CARRAY(CA)#
BEHAVED and C_CONTIGUOUS.
- FARRAY(FA)#
BEHAVED and F_CONTIGUOUS and not C_CONTIGUOUS.
Notes
The flags object can be accessed dictionary-like (as in
a.flags['WRITEABLE']
), or by using lowercased attribute names (as ina.flags.writeable
). Short flag names are only supported in dictionary access.Only the WRITEBACKIFCOPY, WRITEABLE, and ALIGNED flags can be changed by the user, via direct assignment to the attribute or dictionary entry, or by calling ndarray.setflags.
The array flags cannot be set arbitrarily:
WRITEBACKIFCOPY can only be set
False
.ALIGNED can only be set
True
if the data is truly aligned.WRITEABLE can only be set
True
if the array owns its own memory or the ultimate owner of the memory exposes a writeable buffer interface or is a string.
Arrays can be both C-style and Fortran-style contiguous simultaneously. This is clear for 1-dimensional arrays, but can also be true for higher dimensional arrays.
Even for contiguous arrays a stride for a given dimension
arr.strides[dim]
may be arbitrary ifarr.shape[dim] == 1
or the array has no elements. It does not generally hold thatself.strides[-1] == self.itemsize
for C-style contiguous arrays orself.strides[0] == self.itemsize
for Fortran-style contiguous arrays is true.
- flat#
A 1-D iterator over the array.
This is a numpy.flatiter instance, which acts similarly to, but is not a subclass of, Python’s built-in iterator object.
Examples
>>> x = np.arange(1, 7).reshape(2, 3) >>> x array([[1, 2, 3], [4, 5, 6]]) >>> x.flat[3] 4 >>> x.T array([[1, 4], [2, 5], [3, 6]]) >>> x.T.flat[3] 5 >>> type(x.flat) <class 'numpy.flatiter'>
An assignment example:
>>> x.flat = 3; x array([[3, 3, 3], [3, 3, 3]]) >>> x.flat[[1,4]] = 1; x array([[3, 1, 3], [3, 1, 3]])
- flatten(order='C')#
Return a copy of the array collapsed into one dimension.
- Parameters:
order ({'C', 'F', 'A', 'K'}, optional) – ‘C’ means to flatten in row-major (C-style) order. ‘F’ means to flatten in column-major (Fortran- style) order. ‘A’ means to flatten in column-major order if a is Fortran contiguous in memory, row-major order otherwise. ‘K’ means to flatten a in the order the elements occur in memory. The default is ‘C’.
- Returns:
y – A copy of the input array, flattened to one dimension.
- Return type:
ndarray
Examples
>>> a = np.array([[1,2], [3,4]]) >>> a.flatten() array([1, 2, 3, 4]) >>> a.flatten('F') array([1, 3, 2, 4])
- getfield(dtype, offset=0)#
Returns a field of the given array as a certain type.
A field is a view of the array data with a given data-type. The values in the view are determined by the given type and the offset into the current array in bytes. The offset needs to be such that the view dtype fits in the array dtype; for example an array of dtype complex128 has 16-byte elements. If taking a view with a 32-bit integer (4 bytes), the offset needs to be between 0 and 12 bytes.
- Parameters:
dtype (str or dtype) – The data type of the view. The dtype size of the view can not be larger than that of the array itself.
offset (int) – Number of bytes to skip before beginning the element view.
Examples
>>> x = np.diag([1.+1.j]*2) >>> x[1, 1] = 2 + 4.j >>> x array([[1.+1.j, 0.+0.j], [0.+0.j, 2.+4.j]]) >>> x.getfield(np.float64) array([[1., 0.], [0., 2.]])
By choosing an offset of 8 bytes we can select the complex part of the array for our view:
>>> x.getfield(np.float64, offset=8) array([[1., 0.], [0., 4.]])
- imag#
The imaginary part of the array.
Examples
>>> x = np.sqrt([1+0j, 0+1j]) >>> x.imag array([ 0. , 0.70710678]) >>> x.imag.dtype dtype('float64')
- item(*args)#
Copy an element of an array to a standard Python scalar and return it.
- Parameters:
*args (Arguments (variable number and type)) –
none: in this case, the method only works for arrays with one element (a.size == 1), which element is copied into a standard Python scalar object and returned.
int_type: this argument is interpreted as a flat index into the array, specifying which element to copy and return.
tuple of int_types: functions as does a single int_type argument, except that the argument is interpreted as an nd-index into the array.
- Returns:
z – A copy of the specified element of the array as a suitable Python scalar
- Return type:
Standard Python scalar object
Notes
When the data type of a is longdouble or clongdouble, item() returns a scalar array object because there is no available Python scalar that would not lose information. Void arrays return a buffer object for item(), unless fields are defined, in which case a tuple is returned.
item is very similar to a[args], except, instead of an array scalar, a standard Python scalar is returned. This can be useful for speeding up access to elements of the array and doing arithmetic on elements of the array using Python’s optimized math.
Examples
>>> np.random.seed(123) >>> x = np.random.randint(9, size=(3, 3)) >>> x array([[2, 2, 6], [1, 3, 6], [1, 0, 1]]) >>> x.item(3) 1 >>> x.item(7) 0 >>> x.item((0, 1)) 2 >>> x.item((2, 2)) 1
- itemset(*args)#
Insert scalar into an array (scalar is cast to array’s dtype, if possible)
There must be at least 1 argument, and define the last argument as item. Then,
a.itemset(*args)
is equivalent to but faster thana[args] = item
. The item should be a scalar value and args must select a single item in the array a.- Parameters:
*args (Arguments) – If one argument: a scalar, only used in case a is of size 1. If two arguments: the last argument is the value to be set and must be a scalar, the first argument specifies a single array element location. It is either an int or a tuple.
Notes
Compared to indexing syntax, itemset provides some speed increase for placing a scalar into a particular location in an ndarray, if you must do this. However, generally this is discouraged: among other problems, it complicates the appearance of the code. Also, when using itemset (and item) inside a loop, be sure to assign the methods to a local variable to avoid the attribute look-up at each loop iteration.
Examples
>>> np.random.seed(123) >>> x = np.random.randint(9, size=(3, 3)) >>> x array([[2, 2, 6], [1, 3, 6], [1, 0, 1]]) >>> x.itemset(4, 0) >>> x.itemset((2, 2), 9) >>> x array([[2, 2, 6], [1, 0, 6], [1, 0, 9]])
- itemsize#
Length of one array element in bytes.
Examples
>>> x = np.array([1,2,3], dtype=np.float64) >>> x.itemsize 8 >>> x = np.array([1,2,3], dtype=np.complex128) >>> x.itemsize 16
- max(axis=None, out=None, keepdims=False, initial=<no value>, where=True)#
Return the maximum along a given axis.
Refer to numpy.amax for full documentation.
See also
numpy.amax
equivalent function
- mean(axis=None, dtype=None, out=None, keepdims=False, *, where=True)#
Returns the average of the array elements along given axis.
Refer to numpy.mean for full documentation.
See also
numpy.mean
equivalent function
- min(axis=None, out=None, keepdims=False, initial=<no value>, where=True)#
Return the minimum along a given axis.
Refer to numpy.amin for full documentation.
See also
numpy.amin
equivalent function
- nbytes#
Total bytes consumed by the elements of the array.
Notes
Does not include memory consumed by non-element attributes of the array object.
See also
sys.getsizeof
Memory consumed by the object itself without parents in case view. This does include memory consumed by non-element attributes.
Examples
>>> x = np.zeros((3,5,2), dtype=np.complex128) >>> x.nbytes 480 >>> np.prod(x.shape) * x.itemsize 480
- ndim#
Number of array dimensions.
Examples
>>> x = np.array([1, 2, 3]) >>> x.ndim 1 >>> y = np.zeros((2, 3, 4)) >>> y.ndim 3
- newbyteorder(new_order='S', /)#
Return the array with the same data viewed with a different byte order.
Equivalent to:
arr.view(arr.dtype.newbytorder(new_order))
Changes are also made in all fields and sub-arrays of the array data type.
- Parameters:
new_order (string, optional) –
Byte order to force; a value from the byte order specifications below. new_order codes can be any of:
’S’ - swap dtype from current to opposite endian
{‘<’, ‘little’} - little endian
{‘>’, ‘big’} - big endian
{‘=’, ‘native’} - native order, equivalent to sys.byteorder
{‘|’, ‘I’} - ignore (no change to byte order)
The default value (‘S’) results in swapping the current byte order.
- Returns:
new_arr – New array object with the dtype reflecting given change to the byte order.
- Return type:
array
- nonzero()#
Return the indices of the elements that are non-zero.
Refer to numpy.nonzero for full documentation.
See also
numpy.nonzero
equivalent function
- partition(kth, axis=-1, kind='introselect', order=None)#
Rearranges the elements in the array in such a way that the value of the element in kth position is in the position it would be in a sorted array. All elements smaller than the kth element are moved before this element and all equal or greater are moved behind it. The ordering of the elements in the two partitions is undefined.
New in version 1.8.0.
- Parameters:
kth (int or sequence of ints) –
Element index to partition by. The kth element value will be in its final sorted position and all smaller elements will be moved before it and all equal or greater elements behind it. The order of all elements in the partitions is undefined. If provided with a sequence of kth it will partition all elements indexed by kth of them into their sorted position at once.
Deprecated since version 1.22.0: Passing booleans as index is deprecated.
axis (int, optional) – Axis along which to sort. Default is -1, which means sort along the last axis.
kind ({'introselect'}, optional) – Selection algorithm. Default is ‘introselect’.
order (str or list of str, optional) – When a is an array with fields defined, this argument specifies which fields to compare first, second, etc. A single field can be specified as a string, and not all fields need to be specified, but unspecified fields will still be used, in the order in which they come up in the dtype, to break ties.
See also
numpy.partition
Return a partitioned copy of an array.
argpartition
Indirect partition.
sort
Full sort.
Notes
See
np.partition
for notes on the different algorithms.Examples
>>> a = np.array([3, 4, 2, 1]) >>> a.partition(3) >>> a array([2, 1, 3, 4])
>>> a.partition((1, 3)) >>> a array([1, 2, 3, 4])
- prod(axis=None, dtype=None, out=None, keepdims=False, initial=1, where=True)#
Return the product of the array elements over the given axis
Refer to numpy.prod for full documentation.
See also
numpy.prod
equivalent function
- ptp(axis=None, out=None, keepdims=False)#
Peak to peak (maximum - minimum) value along a given axis.
Refer to numpy.ptp for full documentation.
See also
numpy.ptp
equivalent function
- put(indices, values, mode='raise')#
Set
a.flat[n] = values[n]
for all n in indices.Refer to numpy.put for full documentation.
See also
numpy.put
equivalent function
- ravel([order])#
Return a flattened array.
Refer to numpy.ravel for full documentation.
See also
numpy.ravel
equivalent function
ndarray.flat
a flat iterator on the array.
- real#
The real part of the array.
Examples
>>> x = np.sqrt([1+0j, 0+1j]) >>> x.real array([ 1. , 0.70710678]) >>> x.real.dtype dtype('float64')
See also
numpy.real
equivalent function
- repeat(repeats, axis=None)#
Repeat elements of an array.
Refer to numpy.repeat for full documentation.
See also
numpy.repeat
equivalent function
- reshape(shape, order='C')#
Returns an array containing the same data with a new shape.
Refer to numpy.reshape for full documentation.
See also
numpy.reshape
equivalent function
Notes
Unlike the free function numpy.reshape, this method on ndarray allows the elements of the shape parameter to be passed in as separate arguments. For example,
a.reshape(10, 11)
is equivalent toa.reshape((10, 11))
.
- resize(new_shape, refcheck=True)#
Change shape and size of array in-place.
- Parameters:
new_shape (tuple of ints, or n ints) – Shape of resized array.
refcheck (bool, optional) – If False, reference count will not be checked. Default is True.
- Return type:
None
- Raises:
ValueError – If a does not own its own data or references or views to it exist, and the data memory must be changed. PyPy only: will always raise if the data memory must be changed, since there is no reliable way to determine if references or views to it exist.
SystemError – If the order keyword argument is specified. This behaviour is a bug in NumPy.
See also
resize
Return a new array with the specified shape.
Notes
This reallocates space for the data area if necessary.
Only contiguous arrays (data elements consecutive in memory) can be resized.
The purpose of the reference count check is to make sure you do not use this array as a buffer for another Python object and then reallocate the memory. However, reference counts can increase in other ways so if you are sure that you have not shared the memory for this array with another Python object, then you may safely set refcheck to False.
Examples
Shrinking an array: array is flattened (in the order that the data are stored in memory), resized, and reshaped:
>>> a = np.array([[0, 1], [2, 3]], order='C') >>> a.resize((2, 1)) >>> a array([[0], [1]])
>>> a = np.array([[0, 1], [2, 3]], order='F') >>> a.resize((2, 1)) >>> a array([[0], [2]])
Enlarging an array: as above, but missing entries are filled with zeros:
>>> b = np.array([[0, 1], [2, 3]]) >>> b.resize(2, 3) # new_shape parameter doesn't have to be a tuple >>> b array([[0, 1, 2], [3, 0, 0]])
Referencing an array prevents resizing…
>>> c = a >>> a.resize((1, 1)) Traceback (most recent call last): ... ValueError: cannot resize an array that references or is referenced ...
Unless refcheck is False:
>>> a.resize((1, 1), refcheck=False) >>> a array([[0]]) >>> c array([[0]])
- round(decimals=0, out=None)#
Return a with each element rounded to the given number of decimals.
Refer to numpy.around for full documentation.
See also
numpy.around
equivalent function
- searchsorted(v, side='left', sorter=None)#
Find indices where elements of v should be inserted in a to maintain order.
For full documentation, see numpy.searchsorted
See also
numpy.searchsorted
equivalent function
- setfield(val, dtype, offset=0)#
Put a value into a specified place in a field defined by a data-type.
Place val into a’s field defined by dtype and beginning offset bytes into the field.
- Parameters:
val (object) – Value to be placed in field.
dtype (dtype object) – Data-type of the field in which to place val.
offset (int, optional) – The number of bytes into the field at which to place val.
- Return type:
None
See also
Examples
>>> x = np.eye(3) >>> x.getfield(np.float64) array([[1., 0., 0.], [0., 1., 0.], [0., 0., 1.]]) >>> x.setfield(3, np.int32) >>> x.getfield(np.int32) array([[3, 3, 3], [3, 3, 3], [3, 3, 3]], dtype=int32) >>> x array([[1.0e+000, 1.5e-323, 1.5e-323], [1.5e-323, 1.0e+000, 1.5e-323], [1.5e-323, 1.5e-323, 1.0e+000]]) >>> x.setfield(np.eye(3), np.int32) >>> x array([[1., 0., 0.], [0., 1., 0.], [0., 0., 1.]])
- setflags(write=None, align=None, uic=None)#
Set array flags WRITEABLE, ALIGNED, WRITEBACKIFCOPY, respectively.
These Boolean-valued flags affect how numpy interprets the memory area used by a (see Notes below). The ALIGNED flag can only be set to True if the data is actually aligned according to the type. The WRITEBACKIFCOPY and flag can never be set to True. The flag WRITEABLE can only be set to True if the array owns its own memory, or the ultimate owner of the memory exposes a writeable buffer interface, or is a string. (The exception for string is made so that unpickling can be done without copying memory.)
- Parameters:
write (bool, optional) – Describes whether or not a can be written to.
align (bool, optional) – Describes whether or not a is aligned properly for its type.
uic (bool, optional) – Describes whether or not a is a copy of another “base” array.
Notes
Array flags provide information about how the memory area used for the array is to be interpreted. There are 7 Boolean flags in use, only four of which can be changed by the user: WRITEBACKIFCOPY, WRITEABLE, and ALIGNED.
WRITEABLE (W) the data area can be written to;
ALIGNED (A) the data and strides are aligned appropriately for the hardware (as determined by the compiler);
WRITEBACKIFCOPY (X) this array is a copy of some other array (referenced by .base). When the C-API function PyArray_ResolveWritebackIfCopy is called, the base array will be updated with the contents of this array.
All flags can be accessed using the single (upper case) letter as well as the full name.
Examples
>>> y = np.array([[3, 1, 7], ... [2, 0, 0], ... [8, 5, 9]]) >>> y array([[3, 1, 7], [2, 0, 0], [8, 5, 9]]) >>> y.flags C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : True WRITEABLE : True ALIGNED : True WRITEBACKIFCOPY : False >>> y.setflags(write=0, align=0) >>> y.flags C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : True WRITEABLE : False ALIGNED : False WRITEBACKIFCOPY : False >>> y.setflags(uic=1) Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: cannot set WRITEBACKIFCOPY flag to True
- shape#
Tuple of array dimensions.
The shape property is usually used to get the current shape of an array, but may also be used to reshape the array in-place by assigning a tuple of array dimensions to it. As with numpy.reshape, one of the new shape dimensions can be -1, in which case its value is inferred from the size of the array and the remaining dimensions. Reshaping an array in-place will fail if a copy is required.
Warning
Setting
arr.shape
is discouraged and may be deprecated in the future. Using ndarray.reshape is the preferred approach.Examples
>>> x = np.array([1, 2, 3, 4]) >>> x.shape (4,) >>> y = np.zeros((2, 3, 4)) >>> y.shape (2, 3, 4) >>> y.shape = (3, 8) >>> y array([[ 0., 0., 0., 0., 0., 0., 0., 0.], [ 0., 0., 0., 0., 0., 0., 0., 0.], [ 0., 0., 0., 0., 0., 0., 0., 0.]]) >>> y.shape = (3, 6) Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: total size of new array must be unchanged >>> np.zeros((4,2))[::2].shape = (-1,) Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: Incompatible shape for in-place modification. Use `.reshape()` to make a copy with the desired shape.
See also
numpy.shape
Equivalent getter function.
numpy.reshape
Function similar to setting
shape
.ndarray.reshape
Method similar to setting
shape
.
- size#
Number of elements in the array.
Equal to
np.prod(a.shape)
, i.e., the product of the array’s dimensions.Notes
a.size returns a standard arbitrary precision Python integer. This may not be the case with other methods of obtaining the same value (like the suggested
np.prod(a.shape)
, which returns an instance ofnp.int_
), and may be relevant if the value is used further in calculations that may overflow a fixed size integer type.Examples
>>> x = np.zeros((3, 5, 2), dtype=np.complex128) >>> x.size 30 >>> np.prod(x.shape) 30
- sort(axis=-1, kind=None, order=None)#
Sort an array in-place. Refer to numpy.sort for full documentation.
- Parameters:
axis (int, optional) – Axis along which to sort. Default is -1, which means sort along the last axis.
kind ({'quicksort', 'mergesort', 'heapsort', 'stable'}, optional) –
Sorting algorithm. The default is ‘quicksort’. Note that both ‘stable’ and ‘mergesort’ use timsort under the covers and, in general, the actual implementation will vary with datatype. The ‘mergesort’ option is retained for backwards compatibility.
Changed in version 1.15.0: The ‘stable’ option was added.
order (str or list of str, optional) – When a is an array with fields defined, this argument specifies which fields to compare first, second, etc. A single field can be specified as a string, and not all fields need be specified, but unspecified fields will still be used, in the order in which they come up in the dtype, to break ties.
See also
numpy.sort
Return a sorted copy of an array.
numpy.argsort
Indirect sort.
numpy.lexsort
Indirect stable sort on multiple keys.
numpy.searchsorted
Find elements in sorted array.
numpy.partition
Partial sort.
Notes
See numpy.sort for notes on the different sorting algorithms.
Examples
>>> a = np.array([[1,4], [3,1]]) >>> a.sort(axis=1) >>> a array([[1, 4], [1, 3]]) >>> a.sort(axis=0) >>> a array([[1, 3], [1, 4]])
Use the order keyword to specify a field to use when sorting a structured array:
>>> a = np.array([('a', 2), ('c', 1)], dtype=[('x', 'S1'), ('y', int)]) >>> a.sort(order='y') >>> a array([(b'c', 1), (b'a', 2)], dtype=[('x', 'S1'), ('y', '<i8')])
- squeeze(axis=None)#
Remove axes of length one from a.
Refer to numpy.squeeze for full documentation.
See also
numpy.squeeze
equivalent function
- std(axis=None, dtype=None, out=None, ddof=0, keepdims=False, *, where=True)#
Returns the standard deviation of the array elements along given axis.
Refer to numpy.std for full documentation.
See also
numpy.std
equivalent function
- strides#
Tuple of bytes to step in each dimension when traversing an array.
The byte offset of element
(i[0], i[1], ..., i[n])
in an array a is:offset = sum(np.array(i) * a.strides)
A more detailed explanation of strides can be found in the “ndarray.rst” file in the NumPy reference guide.
Warning
Setting
arr.strides
is discouraged and may be deprecated in the future. numpy.lib.stride_tricks.as_strided should be preferred to create a new view of the same data in a safer way.Notes
Imagine an array of 32-bit integers (each 4 bytes):
x = np.array([[0, 1, 2, 3, 4], [5, 6, 7, 8, 9]], dtype=np.int32)
This array is stored in memory as 40 bytes, one after the other (known as a contiguous block of memory). The strides of an array tell us how many bytes we have to skip in memory to move to the next position along a certain axis. For example, we have to skip 4 bytes (1 value) to move to the next column, but 20 bytes (5 values) to get to the same position in the next row. As such, the strides for the array x will be
(20, 4)
.See also
numpy.lib.stride_tricks.as_strided
Examples
>>> y = np.reshape(np.arange(2*3*4), (2,3,4)) >>> y array([[[ 0, 1, 2, 3], [ 4, 5, 6, 7], [ 8, 9, 10, 11]], [[12, 13, 14, 15], [16, 17, 18, 19], [20, 21, 22, 23]]]) >>> y.strides (48, 16, 4) >>> y[1,1,1] 17 >>> offset=sum(y.strides * np.array((1,1,1))) >>> offset/y.itemsize 17
>>> x = np.reshape(np.arange(5*6*7*8), (5,6,7,8)).transpose(2,3,1,0) >>> x.strides (32, 4, 224, 1344) >>> i = np.array([3,5,2,2]) >>> offset = sum(i * x.strides) >>> x[3,5,2,2] 813 >>> offset / x.itemsize 813
- sum(axis=None, dtype=None, out=None, keepdims=False, initial=0, where=True)#
Return the sum of the array elements over the given axis.
Refer to numpy.sum for full documentation.
See also
numpy.sum
equivalent function
- swapaxes(axis1, axis2)#
Return a view of the array with axis1 and axis2 interchanged.
Refer to numpy.swapaxes for full documentation.
See also
numpy.swapaxes
equivalent function
- take(indices, axis=None, out=None, mode='raise')#
Return an array formed from the elements of a at the given indices.
Refer to numpy.take for full documentation.
See also
numpy.take
equivalent function
- tobytes(order='C')#
Construct Python bytes containing the raw data bytes in the array.
Constructs Python bytes showing a copy of the raw contents of data memory. The bytes object is produced in C-order by default. This behavior is controlled by the
order
parameter.New in version 1.9.0.
- Parameters:
order ({'C', 'F', 'A'}, optional) – Controls the memory layout of the bytes object. ‘C’ means C-order, ‘F’ means F-order, ‘A’ (short for Any) means ‘F’ if a is Fortran contiguous, ‘C’ otherwise. Default is ‘C’.
- Returns:
s – Python bytes exhibiting a copy of a’s raw data.
- Return type:
bytes
See also
frombuffer
Inverse of this operation, construct a 1-dimensional array from Python bytes.
Examples
>>> x = np.array([[0, 1], [2, 3]], dtype='<u2') >>> x.tobytes() b'\x00\x00\x01\x00\x02\x00\x03\x00' >>> x.tobytes('C') == x.tobytes() True >>> x.tobytes('F') b'\x00\x00\x02\x00\x01\x00\x03\x00'
- tofile(fid, sep='', format='%s')#
Write array to a file as text or binary (default).
Data is always written in ‘C’ order, independent of the order of a. The data produced by this method can be recovered using the function fromfile().
- Parameters:
fid (file or str or Path) –
An open file object, or a string containing a filename.
Changed in version 1.17.0: pathlib.Path objects are now accepted.
sep (str) – Separator between array items for text output. If “” (empty), a binary file is written, equivalent to
file.write(a.tobytes())
.format (str) – Format string for text file output. Each entry in the array is formatted to text by first converting it to the closest Python type, and then using “format” % item.
Notes
This is a convenience function for quick storage of array data. Information on endianness and precision is lost, so this method is not a good choice for files intended to archive data or transport data between machines with different endianness. Some of these problems can be overcome by outputting the data as text files, at the expense of speed and file size.
When fid is a file object, array contents are directly written to the file, bypassing the file object’s
write
method. As a result, tofile cannot be used with files objects supporting compression (e.g., GzipFile) or file-like objects that do not supportfileno()
(e.g., BytesIO).
- tolist()#
Return the array as an
a.ndim
-levels deep nested list of Python scalars.Return a copy of the array data as a (nested) Python list. Data items are converted to the nearest compatible builtin Python type, via the ~numpy.ndarray.item function.
If
a.ndim
is 0, then since the depth of the nested list is 0, it will not be a list at all, but a simple Python scalar.- Parameters:
none –
- Returns:
y – The possibly nested list of array elements.
- Return type:
object, or list of object, or list of list of object, or …
Notes
The array may be recreated via
a = np.array(a.tolist())
, although this may sometimes lose precision.Examples
For a 1D array,
a.tolist()
is almost the same aslist(a)
, except thattolist
changes numpy scalars to Python scalars:>>> a = np.uint32([1, 2]) >>> a_list = list(a) >>> a_list [1, 2] >>> type(a_list[0]) <class 'numpy.uint32'> >>> a_tolist = a.tolist() >>> a_tolist [1, 2] >>> type(a_tolist[0]) <class 'int'>
Additionally, for a 2D array,
tolist
applies recursively:>>> a = np.array([[1, 2], [3, 4]]) >>> list(a) [array([1, 2]), array([3, 4])] >>> a.tolist() [[1, 2], [3, 4]]
The base case for this recursion is a 0D array:
>>> a = np.array(1) >>> list(a) Traceback (most recent call last): ... TypeError: iteration over a 0-d array >>> a.tolist() 1
- tostring(order='C')#
A compatibility alias for tobytes, with exactly the same behavior.
Despite its name, it returns bytes not strs.
Deprecated since version 1.19.0.
- trace(offset=0, axis1=0, axis2=1, dtype=None, out=None)#
Return the sum along diagonals of the array.
Refer to numpy.trace for full documentation.
See also
numpy.trace
equivalent function
- transpose(*axes)#
Returns a view of the array with axes transposed.
Refer to numpy.transpose for full documentation.
- Parameters:
axes (None, tuple of ints, or n ints) –
None or no argument: reverses the order of the axes.
tuple of ints: i in the j-th place in the tuple means that the array’s i-th axis becomes the transposed array’s j-th axis.
n ints: same as an n-tuple of the same ints (this form is intended simply as a “convenience” alternative to the tuple form).
- Returns:
p – View of the array with its axes suitably permuted.
- Return type:
ndarray
See also
transpose
Equivalent function.
ndarray.T
Array property returning the array transposed.
ndarray.reshape
Give a new shape to an array without changing its data.
Examples
>>> a = np.array([[1, 2], [3, 4]]) >>> a array([[1, 2], [3, 4]]) >>> a.transpose() array([[1, 3], [2, 4]]) >>> a.transpose((1, 0)) array([[1, 3], [2, 4]]) >>> a.transpose(1, 0) array([[1, 3], [2, 4]])
>>> a = np.array([1, 2, 3, 4]) >>> a array([1, 2, 3, 4]) >>> a.transpose() array([1, 2, 3, 4])
- var(axis=None, dtype=None, out=None, ddof=0, keepdims=False, *, where=True)#
Returns the variance of the array elements, along given axis.
Refer to numpy.var for full documentation.
See also
numpy.var
equivalent function
- view([dtype][, type])#
New view of array with the same data.
Note
Passing None for
dtype
is different from omitting the parameter, since the former invokesdtype(None)
which is an alias fordtype('float_')
.- Parameters:
dtype (data-type or ndarray sub-class, optional) – Data-type descriptor of the returned view, e.g., float32 or int16. Omitting it results in the view having the same data-type as a. This argument can also be specified as an ndarray sub-class, which then specifies the type of the returned object (this is equivalent to setting the
type
parameter).type (Python type, optional) – Type of the returned view, e.g., ndarray or matrix. Again, omission of the parameter results in type preservation.
Notes
a.view()
is used two different ways:a.view(some_dtype)
ora.view(dtype=some_dtype)
constructs a view of the array’s memory with a different data-type. This can cause a reinterpretation of the bytes of memory.a.view(ndarray_subclass)
ora.view(type=ndarray_subclass)
just returns an instance of ndarray_subclass that looks at the same array (same shape, dtype, etc.) This does not cause a reinterpretation of the memory.For
a.view(some_dtype)
, ifsome_dtype
has a different number of bytes per entry than the previous dtype (for example, converting a regular array to a structured array), then the last axis ofa
must be contiguous. This axis will be resized in the result.Changed in version 1.23.0: Only the last axis needs to be contiguous. Previously, the entire array had to be C-contiguous.
Examples
>>> x = np.array([(1, 2)], dtype=[('a', np.int8), ('b', np.int8)])
Viewing array data using a different type and dtype:
>>> y = x.view(dtype=np.int16, type=np.matrix) >>> y matrix([[513]], dtype=int16) >>> print(type(y)) <class 'numpy.matrix'>
Creating a view on a structured array so it can be used in calculations
>>> x = np.array([(1, 2),(3,4)], dtype=[('a', np.int8), ('b', np.int8)]) >>> xv = x.view(dtype=np.int8).reshape(-1,2) >>> xv array([[1, 2], [3, 4]], dtype=int8) >>> xv.mean(0) array([2., 3.])
Making changes to the view changes the underlying array
>>> xv[0,1] = 20 >>> x array([(1, 20), (3, 4)], dtype=[('a', 'i1'), ('b', 'i1')])
Using a view to convert an array to a recarray:
>>> z = x.view(np.recarray) >>> z.a array([1, 3], dtype=int8)
Views share data:
>>> x[0] = (9, 10) >>> z[0] (9, 10)
Views that change the dtype size (bytes per entry) should normally be avoided on arrays defined by slices, transposes, fortran-ordering, etc.:
>>> x = np.array([[1, 2, 3], [4, 5, 6]], dtype=np.int16) >>> y = x[:, ::2] >>> y array([[1, 3], [4, 6]], dtype=int16) >>> y.view(dtype=[('width', np.int16), ('length', np.int16)]) Traceback (most recent call last): ... ValueError: To change to a dtype of a different size, the last axis must be contiguous >>> z = y.copy() >>> z.view(dtype=[('width', np.int16), ('length', np.int16)]) array([[(1, 3)], [(4, 6)]], dtype=[('width', '<i2'), ('length', '<i2')])
However, views that change dtype are totally fine for arrays with a contiguous last axis, even if the rest of the axes are not C-contiguous:
>>> x = np.arange(2 * 3 * 4, dtype=np.int8).reshape(2, 3, 4) >>> x.transpose(1, 0, 2).view(np.int16) array([[[ 256, 770], [3340, 3854]], [[1284, 1798], [4368, 4882]], [[2312, 2826], [5396, 5910]]], dtype=int16)
- panel.io.model.diff(doc: Document, binary: bool = True, events: Optional[List[DocumentChangedEvent]] = None) Message[Any] | None [source]#
Returns a json diff required to update an existing plot with the latest plot data.
- panel.io.model.hold(doc: Document, policy: HoldPolicyType = 'combine', comm: Comm | None = None)[source]#
Context manager that holds events on a particular Document allowing them all to be collected and dispatched when the context manager exits. This allows multiple events on the same object to be combined if the policy is set to ‘combine’.
- Parameters:
doc (Document) – The Bokeh Document to hold events on.
policy (HoldPolicyType) – One of ‘combine’, ‘collect’ or None determining whether events setting the same property are combined or accumulated to be dispatched when the context manager exits.
comm (Comm) – The Comm to dispatch events on when the context manager exits.
- panel.io.model.monkeypatch_events(events: List[DocumentChangedEvent]) None [source]#
Patch events applies patches to events that are to be dispatched avoiding various issues in Bokeh.
notebook
Module#

Various utilities for loading JS dependencies and rendering plots inside the Jupyter notebook.
- class panel.io.notebook.JupyterCommJSBinary(id=None, on_msg=None, on_error=None, on_stdout=None, on_open=None)[source]#
Bases:
JupyterCommJS
Extends pyviz_comms.JupyterCommJS with support for repacking binary buffers.
Parameters inherited from:
pyviz_comms.Comm
: id
- class panel.io.notebook.JupyterCommManagerBinary[source]#
Bases:
JupyterCommManager
- client_comm[source]#
alias of
JupyterCommJSBinary
- panel.io.notebook.ipywidget(obj: Any, doc=None, **kwargs: Any)[source]#
Returns an ipywidget model which renders the Panel object.
Requires jupyter_bokeh to be installed.
- Parameters:
obj (object) – Any Panel object or object which can be rendered with Panel
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
**kwargs (dict) – Keyword arguments passed to the pn.panel utility function
- Return type:
Returns an ipywidget model which renders the Panel object.
- panel.io.notebook.mime_renderer(obj)[source]#
Generates a function that will render the supplied object as a mimebundle, e.g. to monkey-patch a _repr_mimebundle_ method onto an existing object.
- panel.io.notebook.mimebundle_to_html(bundle: Dict[str, Any]) str [source]#
Converts a MIME bundle into HTML.
- panel.io.notebook.push(doc: Document, comm: Comm, binary: bool = True, msg: any = None) None [source]#
Pushes events stored on the document across the provided comm.
- panel.io.notebook.push_notebook(*objs: Viewable) None [source]#
A utility for pushing updates to the frontend given a Panel object. This is required when modifying any Bokeh object directly in a notebook session.
- Parameters:
objs (panel.viewable.Viewable) –
- panel.io.notebook.render_embed(panel, max_states: int = 1000, max_opts: int = 3, json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = True, states: Dict[Widget, List[Any]] = {}) None [source]#
Renders a static version of a panel in a notebook by evaluating the set of states defined by the widgets in the model. Note this will only work well for simple apps with a relatively small state space.
- Parameters:
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for JSON filename
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=False)) – Whether to report progress
states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
- panel.io.notebook.render_mimebundle(model: Model, doc: Document, comm: Comm, manager: Optional['CommManager'] = None, location: Optional['Location'] = None, resources: str = 'cdn') Tuple[Dict[str, str], Dict[str, Dict[str, str]]] [source]#
Displays bokeh output inside a notebook using the PyViz display and comms machinery.
- panel.io.notebook.require_components()[source]#
Returns JS snippet to load the required dependencies in the classic notebook using REQUIRE JS.
- panel.io.notebook.send(comm: Comm, msg: any)[source]#
Sends a bokeh message across a pyviz_comms.Comm.
- panel.io.notebook.show_server(panel: Any, notebook_url: str, port: int = 0) Server [source]#
Displays a bokeh server inline in the notebook.
- Parameters:
panel (Viewable) – Panel Viewable object to launch a server for
notebook_url (str) – The URL of the running Jupyter notebook server
port (int (optional, default=0)) – Allows specifying a specific port
server_id (str) – Unique ID to identify the server with
- Returns:
server
- Return type:
bokeh.server.Server
notifications
Module#

- class panel.io.notifications.Notification(*, _destroyed, background, duration, icon, message, notification_area, notification_type, name)[source]#
Bases:
Parameterized
background
= param.Color(allow_None=True, allow_named=True, allow_refs=False, label=’Background’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148767a10>)duration
= param.Integer(allow_refs=False, constant=True, default=3000, inclusive_bounds=(True, True), label=’Duration’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14854e390>)icon
= param.String(allow_None=True, allow_refs=False, label=’Icon’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148616710>)message
= param.String(allow_refs=False, constant=True, default=’’, label=’Message’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148767fd0>)notification_area
= param.Parameter(allow_None=True, allow_refs=False, constant=True, label=’Notification area’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148701f50>)notification_type
= param.String(allow_None=True, allow_refs=False, constant=True, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148767fd0>)_destroyed
= param.Boolean(allow_refs=False, default=False, label=’ destroyed’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148701f50>)
- class panel.io.notifications.NotificationArea(*, types, _clear, js_events, notifications, position, loading, align, aspect_ratio, css_classes, design, height, height_policy, margin, max_height, max_width, min_height, min_width, sizing_mode, styles, stylesheets, tags, visible, width, width_policy, name)[source]#
Bases:
NotificationAreaBase
Parameters inherited from:
panel.viewable.Layoutable
: align, aspect_ratio, css_classes, design, height, min_width, min_height, max_width, max_height, margin, styles, stylesheets, tags, width, width_policy, height_policy, sizing_mode, visiblepanel.viewable.Viewable
: loadingpanel.io.notifications.NotificationAreaBase
: js_events, notifications, position, _cleartypes
= param.List(allow_refs=False, bounds=(0, None), default=[{‘type’: ‘warning’, ‘background’: ‘#ffc107’, ‘icon’: {‘className’: ‘fas fa-exclamation-triangle’, ‘tagName’: ‘i’, ‘color’: ‘white’}}, {‘type’: ‘info’, ‘background’: ‘#007bff’, ‘icon’: {‘className’: ‘fas fa-info-circle’, ‘tagName’: ‘i’, ‘color’: ‘white’}}], label=’Types’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14811e950>)- clone(**params) Viewable [source]#
Makes a copy of the object sharing the same parameters.
- Parameters:
params (Keyword arguments override the parameters on the clone.) –
- Return type:
Cloned Viewable object
- controls(parameters: List[str] = [], jslink: bool = True, **kwargs) Panel [source]#
Creates a set of widgets which allow manipulating the parameters on this instance. By default all parameters which support linking are exposed, but an explicit list of parameters can be provided.
- Parameters:
parameters (list(str)) – An explicit list of parameters to return controls for.
jslink (bool) – Whether to use jslinks instead of Python based links. This does not allow using all types of parameters.
kwargs (dict) – Additional kwargs to pass to the Param pane(s) used to generate the controls widgets.
- Return type:
A layout of the controls
- embed(max_states: int = 1000, max_opts: int = 3, json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = False, states={}) None [source]#
Renders a static version of a panel in a notebook by evaluating the set of states defined by the widgets in the model. Note this will only work well for simple apps with a relatively small state space.
- Parameters:
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for JSON filename
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=False)) – Whether to report progress
states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
- get_root(doc: Optional[Document] = None, comm: Optional[Comm] = None, preprocess: bool = True) Model [source]#
Returns the root model and applies pre-processing hooks
- Parameters:
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
comm (pyviz_comms.Comm) – Optional pyviz_comms when working in notebook
preprocess (boolean (default=True)) – Whether to run preprocessing hooks
- Return type:
Returns the bokeh model corresponding to this panel object
- jscallback(args: Dict[str, Any] = {}, **callbacks: str) Callback [source]#
Allows defining a JS callback to be triggered when a property changes on the source object. The keyword arguments define the properties that trigger a callback and the JS code that gets executed.
- Parameters:
args (dict) – A mapping of objects to make available to the JS callback
**callbacks (dict) – A mapping between properties on the source model and the code to execute when that property changes
- Returns:
callback – The Callback which can be used to disable the callback.
- Return type:
- jslink(target: JSLinkTarget, code: Dict[str, str] = None, args: Optional[Dict] = None, bidirectional: bool = False, **links: str) Link [source]#
Links properties on the this Reactive object to those on the target Reactive object in JS code.
Supports two modes, either specify a mapping between the source and target model properties as keywords or provide a dictionary of JS code snippets which maps from the source parameter to a JS code snippet which is executed when the property changes.
- Parameters:
target (panel.viewable.Viewable | bokeh.model.Model | holoviews.core.dimension.Dimensioned) – The target to link the value to.
code (dict) – Custom code which will be executed when the widget value changes.
args (dict) – A mapping of objects to make available to the JS callback
bidirectional (boolean) – Whether to link source and target bi-directionally
**links (dict) – A mapping between properties on the source model and the target model property to link it to.
- Returns:
link – The GenericLink which can be used unlink the widget and the target model.
- Return type:
GenericLink
- link(target: Parameterized, callbacks: Optional[Dict[str, Union[str, Callable]]] = None, bidirectional: bool = False, **links: str) Watcher [source]#
Links the parameters on this Reactive object to attributes on the target Parameterized object.
Supports two modes, either specify a mapping between the source and target object parameters as keywords or provide a dictionary of callbacks which maps from the source parameter to a callback which is triggered when the parameter changes.
- Parameters:
target (param.Parameterized) – The target object of the link.
callbacks (dict | None) – Maps from a parameter in the source object to a callback.
bidirectional (bool) – Whether to link source and target bi-directionally
**links (dict) – Maps between parameters on this object to the parameters on the supplied object.
- on_event(node: str, event: str, callback: Callable) None [source]#
Registers a callback to be executed when the specified DOM event is triggered on the named node. Note that the named node must be declared in the HTML. To create a named node you must give it an id of the form id=”name”, where name will be the node identifier.
- Parameters:
node (str) – Named node in the HTML identifiable via id of the form id=”name”.
event (str) – Name of the DOM event to add an event listener to.
callback (callable) – A callable which will be given the DOMEvent object.
- save(filename: str | os.PathLike | IO, title: Optional[str] = None, resources: bokeh.resources.Resources | None = None, template: str | jinja2.environment.Template | None = None, template_variables: Dict[str, Any] = {}, embed: bool = False, max_states: int = 1000, max_opts: int = 3, embed_json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = True, embed_states: Dict[Any, Any] = {}, as_png: bool | None = None, **kwargs) None [source]#
Saves Panel objects to file.
- Parameters:
filename (str or file-like object) – Filename to save the plot to
title (string) – Optional title for the plot
resources (bokeh resources) – One of the valid bokeh.resources (e.g. CDN or INLINE)
template – passed to underlying io.save
template_variables – passed to underlying io.save
embed (bool) – Whether the state space should be embedded in the saved file.
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
embed_json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for the auto-generated json directory
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=True)) – Whether to report progress
embed_states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
as_png (boolean (default=None)) – To save as a .png. If None save_png will be true if filename is string and ends with png.
- select(selector: Optional[Union[type, Callable[[Viewable], bool]]] = None) List[Viewable] [source]#
Iterates over the Viewable and any potential children in the applying the Selector.
- Parameters:
selector (type or callable or None) – The selector allows selecting a subset of Viewables by declaring a type or callable function to filter by.
- Returns:
viewables
- Return type:
list(Viewable)
- send(message, duration=3000, type=None, background=None, icon=None)[source]#
Sends a notification to the frontend.
- servable(title: Optional[str] = None, location: bool | 'Location' = True, area: str = 'main', target: Optional[str] = None) ServableMixin [source]#
Serves the object or adds it to the configured pn.state.template if in a panel serve context, writes to the DOM if in a pyodide context and returns the Panel object to allow it to display itself in a notebook context.
- Parameters:
title (str) – A string title to give the Document (if served as an app)
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
area (str (deprecated)) – The area of a template to add the component too. Only has an effect if pn.config.template has been set.
target (str) – Target area to write to. If a template has been configured on pn.config.template this refers to the target area in the template while in pyodide this refers to the ID of the DOM node to write to.
- Return type:
The Panel object itself
- server_doc(doc: Optional[Document] = None, title: Optional[str] = None, location: bool | 'Location' = True) Document [source]#
Returns a serveable bokeh Document with the panel attached
- Parameters:
doc (bokeh.Document (optional)) – The bokeh Document to attach the panel to as a root, defaults to bokeh.io.curdoc()
title (str) – A string title to give the Document
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
- Returns:
doc – The bokeh document the panel was attached to
- Return type:
bokeh.Document
- show(title: Optional[str] = None, port: int = 0, address: Optional[str] = None, websocket_origin: Optional[str] = None, threaded: bool = False, verbose: bool = True, open: bool = True, location: bool | 'Location' = True, **kwargs) StoppableThread' | 'Server [source]#
Starts a Bokeh server and displays the Viewable in a new tab.
- Parameters:
title (str | None) – A string title to give the Document (if served as an app)
port (int (optional, default=0)) – Allows specifying a specific port
address (str) – The address the server should listen on for HTTP requests.
websocket_origin (str or list(str) (optional)) – A list of hosts that can connect to the websocket. This is typically required when embedding a server app in an external web site. If None, “localhost” is used.
threaded (boolean (optional, default=False)) – Whether to launch the Server on a separate thread, allowing interactive use.
verbose (boolean (optional, default=True)) – Whether to print the address and port
open (boolean (optional, default=True)) – Whether to open the server in a new browser tab
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
- Returns:
server – Returns the Bokeh server instance or the thread the server was launched on (if threaded=True)
- Return type:
bokeh.server.Server or panel.io.server.StoppableThread
- class panel.io.notifications.NotificationAreaBase(*, _clear, js_events, notifications, position, loading, align, aspect_ratio, css_classes, design, height, height_policy, margin, max_height, max_width, min_height, min_width, sizing_mode, styles, stylesheets, tags, visible, width, width_policy, name)[source]#
Bases:
ReactiveHTML
Parameters inherited from:
panel.viewable.Layoutable
: align, aspect_ratio, css_classes, design, height, min_width, min_height, max_width, max_height, margin, styles, stylesheets, tags, width, width_policy, height_policy, sizing_mode, visiblepanel.viewable.Viewable
: loadingjs_events
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Js events’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x148767490>)A dictionary that configures notifications for specific Bokeh Document events, e.g.: {‘connection_lost’: {‘type’: ‘error’, ‘message’: ‘Connection Lost!’, ‘duration’: 5}} will trigger a warning on the Bokeh ConnectionLost event.
notifications
= param.List(allow_refs=False, bounds=(0, None), class_=<class ‘panel.io.notifications.Notification’>, default=[], item_type=<class ‘panel.io.notifications.Notification’>, label=’Notifications’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14872ff50>)position
= param.Selector(allow_refs=False, default=’bottom-right’, label=’Position’, names={}, nested_refs=False, objects=[‘bottom-right’, ‘bottom-left’, ‘bottom-center’, ‘top-left’, ‘top-right’, ‘top-center’, ‘center-center’, ‘center-left’, ‘center-right’], rx=<param.reactive.reactive_ops object at 0x148770490>)_clear
= param.Integer(allow_refs=False, default=0, inclusive_bounds=(True, True), label=’ clear’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14872f690>)- clone(**params) Viewable [source]#
Makes a copy of the object sharing the same parameters.
- Parameters:
params (Keyword arguments override the parameters on the clone.) –
- Return type:
Cloned Viewable object
- controls(parameters: List[str] = [], jslink: bool = True, **kwargs) Panel [source]#
Creates a set of widgets which allow manipulating the parameters on this instance. By default all parameters which support linking are exposed, but an explicit list of parameters can be provided.
- Parameters:
parameters (list(str)) – An explicit list of parameters to return controls for.
jslink (bool) – Whether to use jslinks instead of Python based links. This does not allow using all types of parameters.
kwargs (dict) – Additional kwargs to pass to the Param pane(s) used to generate the controls widgets.
- Return type:
A layout of the controls
- embed(max_states: int = 1000, max_opts: int = 3, json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = False, states={}) None [source]#
Renders a static version of a panel in a notebook by evaluating the set of states defined by the widgets in the model. Note this will only work well for simple apps with a relatively small state space.
- Parameters:
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for JSON filename
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=False)) – Whether to report progress
states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
- get_root(doc: Optional[Document] = None, comm: Optional[Comm] = None, preprocess: bool = True) Model [source]#
Returns the root model and applies pre-processing hooks
- Parameters:
doc (bokeh.Document) – Bokeh document the bokeh model will be attached to.
comm (pyviz_comms.Comm) – Optional pyviz_comms when working in notebook
preprocess (boolean (default=True)) – Whether to run preprocessing hooks
- Return type:
Returns the bokeh model corresponding to this panel object
- jscallback(args: Dict[str, Any] = {}, **callbacks: str) Callback [source]#
Allows defining a JS callback to be triggered when a property changes on the source object. The keyword arguments define the properties that trigger a callback and the JS code that gets executed.
- Parameters:
args (dict) – A mapping of objects to make available to the JS callback
**callbacks (dict) – A mapping between properties on the source model and the code to execute when that property changes
- Returns:
callback – The Callback which can be used to disable the callback.
- Return type:
- jslink(target: JSLinkTarget, code: Dict[str, str] = None, args: Optional[Dict] = None, bidirectional: bool = False, **links: str) Link [source]#
Links properties on the this Reactive object to those on the target Reactive object in JS code.
Supports two modes, either specify a mapping between the source and target model properties as keywords or provide a dictionary of JS code snippets which maps from the source parameter to a JS code snippet which is executed when the property changes.
- Parameters:
target (panel.viewable.Viewable | bokeh.model.Model | holoviews.core.dimension.Dimensioned) – The target to link the value to.
code (dict) – Custom code which will be executed when the widget value changes.
args (dict) – A mapping of objects to make available to the JS callback
bidirectional (boolean) – Whether to link source and target bi-directionally
**links (dict) – A mapping between properties on the source model and the target model property to link it to.
- Returns:
link – The GenericLink which can be used unlink the widget and the target model.
- Return type:
GenericLink
- link(target: Parameterized, callbacks: Optional[Dict[str, Union[str, Callable]]] = None, bidirectional: bool = False, **links: str) Watcher [source]#
Links the parameters on this Reactive object to attributes on the target Parameterized object.
Supports two modes, either specify a mapping between the source and target object parameters as keywords or provide a dictionary of callbacks which maps from the source parameter to a callback which is triggered when the parameter changes.
- Parameters:
target (param.Parameterized) – The target object of the link.
callbacks (dict | None) – Maps from a parameter in the source object to a callback.
bidirectional (bool) – Whether to link source and target bi-directionally
**links (dict) – Maps between parameters on this object to the parameters on the supplied object.
- on_event(node: str, event: str, callback: Callable) None [source]#
Registers a callback to be executed when the specified DOM event is triggered on the named node. Note that the named node must be declared in the HTML. To create a named node you must give it an id of the form id=”name”, where name will be the node identifier.
- Parameters:
node (str) – Named node in the HTML identifiable via id of the form id=”name”.
event (str) – Name of the DOM event to add an event listener to.
callback (callable) – A callable which will be given the DOMEvent object.
- save(filename: str | os.PathLike | IO, title: Optional[str] = None, resources: bokeh.resources.Resources | None = None, template: str | jinja2.environment.Template | None = None, template_variables: Dict[str, Any] = {}, embed: bool = False, max_states: int = 1000, max_opts: int = 3, embed_json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = True, embed_states: Dict[Any, Any] = {}, as_png: bool | None = None, **kwargs) None [source]#
Saves Panel objects to file.
- Parameters:
filename (str or file-like object) – Filename to save the plot to
title (string) – Optional title for the plot
resources (bokeh resources) – One of the valid bokeh.resources (e.g. CDN or INLINE)
template – passed to underlying io.save
template_variables – passed to underlying io.save
embed (bool) – Whether the state space should be embedded in the saved file.
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
embed_json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for the auto-generated json directory
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=True)) – Whether to report progress
embed_states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
as_png (boolean (default=None)) – To save as a .png. If None save_png will be true if filename is string and ends with png.
- select(selector: Optional[Union[type, Callable[[Viewable], bool]]] = None) List[Viewable] [source]#
Iterates over the Viewable and any potential children in the applying the Selector.
- Parameters:
selector (type or callable or None) – The selector allows selecting a subset of Viewables by declaring a type or callable function to filter by.
- Returns:
viewables
- Return type:
list(Viewable)
- send(message, duration=3000, type=None, background=None, icon=None)[source]#
Sends a notification to the frontend.
- servable(title: Optional[str] = None, location: bool | 'Location' = True, area: str = 'main', target: Optional[str] = None) ServableMixin [source]#
Serves the object or adds it to the configured pn.state.template if in a panel serve context, writes to the DOM if in a pyodide context and returns the Panel object to allow it to display itself in a notebook context.
- Parameters:
title (str) – A string title to give the Document (if served as an app)
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
area (str (deprecated)) – The area of a template to add the component too. Only has an effect if pn.config.template has been set.
target (str) – Target area to write to. If a template has been configured on pn.config.template this refers to the target area in the template while in pyodide this refers to the ID of the DOM node to write to.
- Return type:
The Panel object itself
- server_doc(doc: Optional[Document] = None, title: Optional[str] = None, location: bool | 'Location' = True) Document [source]#
Returns a serveable bokeh Document with the panel attached
- Parameters:
doc (bokeh.Document (optional)) – The bokeh Document to attach the panel to as a root, defaults to bokeh.io.curdoc()
title (str) – A string title to give the Document
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
- Returns:
doc – The bokeh document the panel was attached to
- Return type:
bokeh.Document
- show(title: Optional[str] = None, port: int = 0, address: Optional[str] = None, websocket_origin: Optional[str] = None, threaded: bool = False, verbose: bool = True, open: bool = True, location: bool | 'Location' = True, **kwargs) StoppableThread' | 'Server [source]#
Starts a Bokeh server and displays the Viewable in a new tab.
- Parameters:
title (str | None) – A string title to give the Document (if served as an app)
port (int (optional, default=0)) – Allows specifying a specific port
address (str) – The address the server should listen on for HTTP requests.
websocket_origin (str or list(str) (optional)) – A list of hosts that can connect to the websocket. This is typically required when embedding a server app in an external web site. If None, “localhost” is used.
threaded (boolean (optional, default=False)) – Whether to launch the Server on a separate thread, allowing interactive use.
verbose (boolean (optional, default=True)) – Whether to print the address and port
open (boolean (optional, default=True)) – Whether to open the server in a new browser tab
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
- Returns:
server – Returns the Bokeh server instance or the thread the server was launched on (if threaded=True)
- Return type:
bokeh.server.Server or panel.io.server.StoppableThread
profile
Module#
- panel.io.profile.profile(name, engine='pyinstrument')[source]#
A decorator which may be added to any function to record profiling output.
- Parameters:
name (str) – A unique name for the profiling session.
engine (str) – The profiling engine, e.g. ‘pyinstrument’, ‘snakeviz’ or ‘memray’
- panel.io.profile.profile_ctx(engine='pyinstrument')[source]#
A context manager which profiles the body of the with statement with the supplied profiling engine and returns the profiling object in a list.
- Parameters:
engine (str) – The profiling engine, e.g. ‘pyinstrument’, ‘snakeviz’ or ‘memray’
- Returns:
sessions – A list containing the profiling session.
- Return type:
list
pyodide
Module#
reload
Module#
- panel.io.reload.file_is_in_folder_glob(filepath, folderpath_glob)[source]#
Test whether a file is in some folder with globbing support.
- Parameters:
filepath (str) – A file path.
folderpath_glob (str) – A path to a folder that may include globbing.
- panel.io.reload.record_modules(applications=None, handler=None)[source]#
Records modules which are currently imported.
resources
Module#
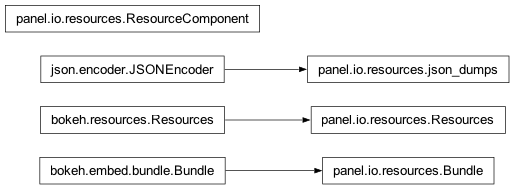
Patches bokeh resources to make it easy to add external JS and CSS resources via the panel.config object.
- class panel.io.resources.ResourceComponent[source]#
Bases:
object
Mix-in class for components that define a set of resources that have to be resolved.
- resolve_resources(cdn: bool | Literal['auto'] = 'auto', extras: dict[str, dict[str, str]] | None = None) ResourcesType [source]#
Resolves the resources required for this component.
- Parameters:
cdn (bool | Literal['auto']) – Whether to load resources from CDN or local server. If set to ‘auto’ value will be automatically determine based on global settings.
extras (dict[str, dict[str, str]] | None) – Additional resources to add to the bundle. Valid resource types include js, js_modules and css.
- Return type:
Dictionary containing JS and CSS resources.
- class panel.io.resources.Resources(*args, absolute=False, notebook=False, **kwargs)[source]#
Bases:
Resources
- panel.io.resources.component_resource_path(component, attr, path)[source]#
Generates a canonical URL for a component resource.
To be used in conjunction with the panel.io.server.ComponentResourceHandler which allows dynamically resolving resources defined on components.
- class panel.io.resources.json_dumps(*, skipkeys=False, ensure_ascii=True, check_circular=True, allow_nan=True, sort_keys=False, indent=None, separators=None, default=None)[source]#
Bases:
JSONEncoder
- default(obj)[source]#
Implement this method in a subclass such that it returns a serializable object for
o
, or calls the base implementation (to raise aTypeError
).For example, to support arbitrary iterators, you could implement default like this:
def default(self, o): try: iterable = iter(o) except TypeError: pass else: return list(iterable) # Let the base class default method raise the TypeError return JSONEncoder.default(self, o)
- panel.io.resources.patch_model_css(root, dist_url)[source]#
Temporary patch for Model.css property used by Panel to provide stylesheets for components.
ALERT: Should find better solution before official Bokeh 3.x compatible release
- panel.io.resources.process_raw_css(raw_css)[source]#
Converts old-style Bokeh<3 compatible CSS to Bokeh 3 compatible CSS.
- panel.io.resources.resolve_custom_path(obj, path: str | os.PathLike, relative: bool = False) pathlib.Path | None [source]#
Attempts to resolve a path relative to some component.
- Parameters:
obj (type | object) – The component to resolve the path relative to.
path (str | os.PathLike) – Absolute or relative path to a resource.
relative (bool) – Whether to return a relative path.
- Returns:
path
- Return type:
pathlib.Path | None
- panel.io.resources.resolve_stylesheet(cls, stylesheet: str, attribute: str | None = None)[source]#
Resolves a stylesheet definition, e.g. originating on a component Reactive._stylesheets or a Design.modifiers attribute. Stylesheets may be defined as one of the following:
Absolute URL defined with http(s) protocol
A path relative to the component
A raw css string
- Parameters:
cls (type | object) – Object or class defining the stylesheet
stylesheet (str) – The stylesheet definition
rest
Module#

- class panel.io.rest.BaseHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
RequestHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_current_user() Any [source]#
Override to determine the current user from, e.g., a cookie.
This method may not be a coroutine.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- prepare() Optional[Awaitable[None]] [source]#
Called at the beginning of a request before get/post/etc.
Override this method to perform common initialization regardless of the request method.
Asynchronous support: Use
async def
or decorate this method with .gen.coroutine to make it asynchronous. If this method returns anAwaitable
execution will not proceed until theAwaitable
is done.New in version 3.1: Asynchronous support.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code, **kwargs)[source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- exception panel.io.rest.HTTPError(status_code: int = 500, log_message: Optional[str] = None, *args: Any, **kwargs: Any)[source]#
Bases:
HTTPError
Custom HTTPError type
- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class panel.io.rest.ParamHandler(app, request, **kwargs)[source]#
Bases:
BaseHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_current_user() Any [source]#
Override to determine the current user from, e.g., a cookie.
This method may not be a coroutine.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- prepare() Optional[Awaitable[None]] [source]#
Called at the beginning of a request before get/post/etc.
Override this method to perform common initialization regardless of the request method.
Asynchronous support: Use
async def
or decorate this method with .gen.coroutine to make it asynchronous. If this method returns anAwaitable
execution will not proceed until theAwaitable
is done.New in version 3.1: Asynchronous support.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code, **kwargs)[source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- panel.io.rest.param_rest_provider(files, endpoint)[source]#
Returns a Param based REST API given the scripts or notebooks containing the tranquilized functions.
- Parameters:
files (list(str)) – A list of paths being served
endpoint (str) – The endpoint to serve the REST API on
- Return type:
A Tornado routing pattern containing the route and handler
- panel.io.rest.tranquilizer_rest_provider(files, endpoint)[source]#
Returns a Tranquilizer based REST API. Builds the API by evaluating the scripts and notebooks being served and finding all tranquilized functions inside them.
- Parameters:
files (list(str)) – A list of paths being served
endpoint (str) – The endpoint to serve the REST API on
- Return type:
A Tornado routing pattern containing the route and handler
save
Module#
Defines utilities to save panel objects to files as HTML or PNG.
- panel.io.save.save(panel: Viewable, filename: str | os.PathLike | IO, title: Optional[str] = None, resources: BkResources | None = None, template: Template | str | None = None, template_variables: Dict[str, Any] = None, embed: bool = False, max_states: int = 1000, max_opts: int = 3, embed_json: bool = False, json_prefix: str = '', save_path: str = './', load_path: Optional[str] = None, progress: bool = True, embed_states={}, as_png=None, **kwargs) None [source]#
Saves Panel objects to file.
- Parameters:
panel (Viewable) – The Panel Viewable to save to file
filename (str or file-like object) – Filename to save the plot to
title (str) – Optional title for the plot
resources (bokeh.resources.Resources) – One of the valid bokeh.resources (e.g. CDN or INLINE)
template (jinja2.Template | str) – template file, as used by bokeh.file_html. If None will use bokeh defaults
template_variables (Dict[str, Any]) – template_variables file dict, as used by bokeh.file_html
embed (bool) – Whether the state space should be embedded in the saved file.
max_states (int) – The maximum number of states to embed
max_opts (int) – The maximum number of states for a single widget
embed_json (boolean (default=True)) – Whether to export the data to json files
json_prefix (str (default='')) – Prefix for the randomly json directory
save_path (str (default='./')) – The path to save json files to
load_path (str (default=None)) – The path or URL the json files will be loaded from.
progress (boolean (default=True)) – Whether to report progress
embed_states (dict (default={})) – A dictionary specifying the widget values to embed for each widget
as_png (boolean (default=None)) – To save as a .png. If None save_png will be true if filename is string and ends with png.
- panel.io.save.save_png(model: Model, filename: str, resources=Resources(mode='cdn'), template=None, template_variables=None, timeout: int = 5) None [source]#
Saves a bokeh model to png
- Parameters:
model (bokeh.model.Model) – Model to save to png
filename (str) – Filename to save to
resources (str) – Resources
template – template file, as used by bokeh.file_html. If None will use bokeh defaults
template_variables – template_variables file dict, as used by bokeh.file_html
timeout (int) – The maximum amount of time (in seconds) to wait for
server
Module#

Utilities for creating bokeh Server instances.
- class panel.io.server.AutoloadJsHandler(tornado_app: BokehTornado, *args, **kw)[source]#
Bases:
AutoloadJsHandler
,SessionPrefixHandler
Implements a custom Tornado handler for the autoload JS chunk
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url()[source]#
Delegates to``get_login_url`` method of the auth provider, or the
login_url
attribute.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- async options(*args, **kwargs)[source]#
Browsers make OPTIONS requests under the hood before a GET request
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers()[source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.server.ComponentResourceHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
StaticFileHandler
A handler that serves local resources relative to a Python module. The handler resolves a specific Panel component by module reference and name, then resolves an attribute on that component to check if it contains the requested resource path.
/<endpoint>/<module>/<class>/<attribute>/<path>
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Sets the
Etag
header based on static url version.This allows efficient
If-None-Match
checks against cached versions, and sends the correctEtag
for a partial response (i.e. the sameEtag
as the full file).New in version 3.1.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- classmethod get_absolute_path(root: str, path: str) str [source]#
Returns the absolute location of
path
relative toroot
.root
is the path configured for this StaticFileHandler (in most cases thestatic_path
Application setting).This class method may be overridden in subclasses. By default it returns a filesystem path, but other strings may be used as long as they are unique and understood by the subclass’s overridden get_content.
New in version 3.1.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cache_time(path: str, modified: Optional[datetime], mime_type: str) int [source]#
Override to customize cache control behavior.
Return a positive number of seconds to make the result cacheable for that amount of time or 0 to mark resource as cacheable for an unspecified amount of time (subject to browser heuristics).
By default returns cache expiry of 10 years for resources requested with
v
argument.
- classmethod get_content(abspath: str, start: Optional[int] = None, end: Optional[int] = None) Generator[bytes, None, None] [source]#
Retrieve the content of the requested resource which is located at the given absolute path.
This class method may be overridden by subclasses. Note that its signature is different from other overridable class methods (no
settings
argument); this is deliberate to ensure thatabspath
is able to stand on its own as a cache key.This method should either return a byte string or an iterator of byte strings. The latter is preferred for large files as it helps reduce memory fragmentation.
New in version 3.1.
- get_content_size() int [source]#
Retrieve the total size of the resource at the given path.
This method may be overridden by subclasses.
New in version 3.1.
Changed in version 4.0: This method is now always called, instead of only when partial results are requested.
- get_content_type() str [source]#
Returns the
Content-Type
header to be used for this request.New in version 3.1.
- classmethod get_content_version(abspath: str) str [source]#
Returns a version string for the resource at the given path.
This class method may be overridden by subclasses. The default implementation is a SHA-512 hash of the file’s contents.
New in version 3.1.
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_current_user() Any [source]#
Override to determine the current user from, e.g., a cookie.
This method may not be a coroutine.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_modified_time() Optional[datetime] [source]#
Returns the time that
self.absolute_path
was last modified.May be overridden in subclasses. Should return a ~datetime.datetime object or None.
New in version 3.1.
Changed in version 6.4: Now returns an aware datetime object instead of a naive one. Subclasses that override this method may return either kind.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- classmethod get_version(settings: Dict[str, Any], path: str) Optional[str] [source]#
Generate the version string to be used in static URLs.
settings
is the Application.settings dictionary andpath
is the relative location of the requested asset on the filesystem. The returned value should be a string, orNone
if no version could be determined.Changed in version 3.1: This method was previously recommended for subclasses to override; get_content_version is now preferred as it allows the base class to handle caching of the result.
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- classmethod make_static_url(settings: Dict[str, Any], path: str, include_version: bool = True) str [source]#
Constructs a versioned url for the given path.
This method may be overridden in subclasses (but note that it is a class method rather than an instance method). Subclasses are only required to implement the signature
make_static_url(cls, settings, path)
; other keyword arguments may be passed through ~RequestHandler.static_url but are not standard.settings
is the Application.settings dictionary.path
is the static path being requested. The url returned should be relative to the current host.include_version
determines whether the generated URL should include the query string containing the version hash of the file corresponding to the givenpath
.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- prepare() Optional[Awaitable[None]] [source]#
Called at the beginning of a request before get/post/etc.
Override this method to perform common initialization regardless of the request method.
Asynchronous support: Use
async def
or decorate this method with .gen.coroutine to make it asynchronous. If this method returns anAwaitable
execution will not proceed until theAwaitable
is done.New in version 3.1: Asynchronous support.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_headers() None [source]#
Sets the content and caching headers on the response.
New in version 3.1.
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- should_return_304() bool [source]#
Returns True if the headers indicate that we should return 304.
New in version 3.1.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- validate_absolute_path(root: str, absolute_path: str) str [source]#
Validate and return the absolute path.
root
is the configured path for the StaticFileHandler, andpath
is the result of get_absolute_pathThis is an instance method called during request processing, so it may raise HTTPError or use methods like RequestHandler.redirect (return None after redirecting to halt further processing). This is where 404 errors for missing files are generated.
This method may modify the path before returning it, but note that any such modifications will not be understood by make_static_url.
In instance methods, this method’s result is available as
self.absolute_path
.New in version 3.1.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.server.DocHandler(tornado_app: BokehTornado, *args, **kw)[source]#
Bases:
LoginUrlMixin
,DocHandler
,SessionPrefixHandler
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url()[source]#
Delegates to``get_login_url`` method of the auth provider, or the
login_url
attribute.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.server.LoginUrlMixin[source]#
Bases:
object
Overrides the AuthRequestHandler.get_login_url implementation to correctly handle prefixes.
- class panel.io.server.ProxyFallbackHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
RequestHandler
A RequestHandler that wraps another HTTP server callback and proxies the subpath.
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_current_user() Any [source]#
Override to determine the current user from, e.g., a cookie.
This method may not be a coroutine.
- get_login_url() str [source]#
Override to customize the login URL based on the request.
By default, we use the
login_url
application setting.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- prepare()[source]#
Called at the beginning of a request before get/post/etc.
Override this method to perform common initialization regardless of the request method.
Asynchronous support: Use
async def
or decorate this method with .gen.coroutine to make it asynchronous. If this method returns anAwaitable
execution will not proceed until theAwaitable
is done.New in version 3.1: Asynchronous support.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(template_name: str, **kwargs: Any) Future[None] [source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.server.RootHandler(application: Application, request: HTTPServerRequest, **kwargs: Any)[source]#
Bases:
LoginUrlMixin
,RootHandler
Custom RootHandler that provides the CDN_DIST directory as a template variable.
- add_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Adds the given response header and value.
Unlike set_header, add_header may be called multiple times to return multiple values for the same header.
- check_etag_header() bool [source]#
Checks the
Etag
header against requests’sIf-None-Match
.Returns
True
if the request’s Etag matches and a 304 should be returned. For example:self.set_etag_header() if self.check_etag_header(): self.set_status(304) return
This method is called automatically when the request is finished, but may be called earlier for applications that override compute_etag and want to do an early check for
If-None-Match
before completing the request. TheEtag
header should be set (perhaps with set_etag_header) before calling this method.
- check_xsrf_cookie() None [source]#
Verifies that the
_xsrf
cookie matches the_xsrf
argument.To prevent cross-site request forgery, we set an
_xsrf
cookie and include the same value as a non-cookie field with allPOST
requests. If the two do not match, we reject the form submission as a potential forgery.The
_xsrf
value may be set as either a form field named_xsrf
or in a custom HTTP header namedX-XSRFToken
orX-CSRFToken
(the latter is accepted for compatibility with Django).See http://en.wikipedia.org/wiki/Cross-site_request_forgery
Changed in version 3.2.2: Added support for cookie version 2. Both versions 1 and 2 are supported.
- clear_all_cookies(**kwargs: Any) None [source]#
Attempt to delete all the cookies the user sent with this request.
See clear_cookie for more information on keyword arguments. Due to limitations of the cookie protocol, it is impossible to determine on the server side which values are necessary for the
domain
,path
,samesite
, orsecure
arguments, this method can only be successful if you consistently use the same values for these arguments when setting cookies.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2: Added the
path
anddomain
parameters.Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does.Deprecated since version 6.3: The increasingly complex rules governing cookies have made it impossible for a
clear_all_cookies
method to work reliably since all we know about cookies are their names. Applications should generally useclear_cookie
one at a time instead.
- clear_cookie(name: str, **kwargs: Any) None [source]#
Deletes the cookie with the given name.
This method accepts the same arguments as set_cookie, except for
expires
andmax_age
. Clearing a cookie requires the samedomain
andpath
arguments as when it was set. In some cases thesamesite
andsecure
arguments are also required to match. Other arguments are ignored.Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 6.3: Now accepts all keyword arguments that
set_cookie
does. Thesamesite
andsecure
flags have recently become required for clearingsamesite="none"
cookies.
- clear_header(name: str) None [source]#
Clears an outgoing header, undoing a previous set_header call.
Note that this method does not apply to multi-valued headers set by add_header.
- compute_etag() Optional[str] [source]#
Computes the etag header to be used for this request.
By default uses a hash of the content written so far.
May be overridden to provide custom etag implementations, or may return None to disable tornado’s default etag support.
- property cookies: Dict[str, Morsel]#
An alias for self.request.cookies <.httputil.HTTPServerRequest.cookies>.
- create_signed_value(name: str, value: Union[str, bytes], version: Optional[int] = None) bytes [source]#
Signs and timestamps a string so it cannot be forged.
Normally used via set_signed_cookie, but provided as a separate method for non-cookie uses. To decode a value not stored as a cookie use the optional value argument to get_signed_cookie.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.
- create_template_loader(template_path: str) BaseLoader [source]#
Returns a new template loader for the given path.
May be overridden by subclasses. By default returns a directory-based loader on the given path, using the
autoescape
andtemplate_whitespace
application settings. If atemplate_loader
application setting is supplied, uses that instead.
- property current_user: Any#
The authenticated user for this request.
This is set in one of two ways:
A subclass may override get_current_user(), which will be called automatically the first time
self.current_user
is accessed. get_current_user() will only be called once per request, and is cached for future access:def get_current_user(self): user_cookie = self.get_signed_cookie("user") if user_cookie: return json.loads(user_cookie) return None
It may be set as a normal variable, typically from an overridden prepare():
@gen.coroutine def prepare(self): user_id_cookie = self.get_signed_cookie("user_id") if user_id_cookie: self.current_user = yield load_user(user_id_cookie)
Note that prepare() may be a coroutine while get_current_user() may not, so the latter form is necessary if loading the user requires asynchronous operations.
The user object may be any type of the application’s choosing.
- data_received(chunk: bytes) Optional[Awaitable[None]] [source]#
Implement this method to handle streamed request data.
Requires the .stream_request_body decorator.
May be a coroutine for flow control.
- decode_argument(value: bytes, name: Optional[str] = None) str [source]#
Decodes an argument from the request.
The argument has been percent-decoded and is now a byte string. By default, this method decodes the argument as utf-8 and returns a unicode string, but this may be overridden in subclasses.
This method is used as a filter for both get_argument() and for values extracted from the url and passed to get()/post()/etc.
The name of the argument is provided if known, but may be None (e.g. for unnamed groups in the url regex).
- detach() IOStream [source]#
Take control of the underlying stream.
Returns the underlying .IOStream object and stops all further HTTP processing. Intended for implementing protocols like websockets that tunnel over an HTTP handshake.
This method is only supported when HTTP/1.1 is used.
New in version 5.1.
- finish(chunk: Optional[Union[str, bytes, dict]] = None) Future[None] [source]#
Finishes this response, ending the HTTP request.
Passing a
chunk
tofinish()
is equivalent to passing that chunk towrite()
and then callingfinish()
with no arguments.Returns a .Future which may optionally be awaited to track the sending of the response to the client. This .Future resolves when all the response data has been sent, and raises an error if the connection is closed before all data can be sent.
Changed in version 5.1: Now returns a .Future instead of
None
.
- flush(include_footers: bool = False) Future[None] [source]#
Flushes the current output buffer to the network.
Changed in version 4.0: Now returns a .Future if no callback is given.
Changed in version 6.0: The
callback
argument was removed.
- get_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the request more than once, we return the last value.
This method searches both the query and body arguments.
- get_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the arguments with the given name.
If the argument is not present, returns an empty list.
This method searches both the query and body arguments.
- get_body_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request body.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_body_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the body arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_browser_locale(default: str = 'en_US') Locale [source]#
Determines the user’s locale from
Accept-Language
header.See http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.4
- get_cookie(name: str, default: Optional[str] = None) Optional[str] [source]#
Returns the value of the request cookie with the given name.
If the named cookie is not present, returns
default
.This method only returns cookies that were present in the request. It does not see the outgoing cookies set by set_cookie in this handler.
- get_login_url()[source]#
Delegates to``get_login_url`` method of the auth provider, or the
login_url
attribute.
- get_query_argument(name: str, default: ~typing.Union[None, str, ~tornado.web._ArgDefaultMarker] = <tornado.web._ArgDefaultMarker object>, strip: bool = True) Optional[str] [source]#
Returns the value of the argument with the given name from the request query string.
If default is not provided, the argument is considered to be required, and we raise a MissingArgumentError if it is missing.
If the argument appears in the url more than once, we return the last value.
New in version 3.2.
- get_query_arguments(name: str, strip: bool = True) List[str] [source]#
Returns a list of the query arguments with the given name.
If the argument is not present, returns an empty list.
New in version 3.2.
- get_secure_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_secure_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie(name: str, value: Optional[str] = None, max_age_days: float = 31, min_version: Optional[int] = None) Optional[bytes] [source]#
Returns the given signed cookie if it validates, or None.
The decoded cookie value is returned as a byte string (unlike get_cookie).
Similar to get_cookie, this method only returns cookies that were present in the request. It does not see outgoing cookies set by set_signed_cookie in this handler.
Changed in version 3.2.1:
Added the
min_version
argument. Introduced cookie version 2; both versions 1 and 2 are accepted by default.Changed in version 6.3: Renamed from
get_secure_cookie
toget_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_signed_cookie_key_version(name: str, value: Optional[str] = None) Optional[int] [source]#
Returns the signing key version of the secure cookie.
The version is returned as int.
Changed in version 6.3: Renamed from
get_secure_cookie_key_version
toset_signed_cookie_key_version
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- get_template_namespace() Dict[str, Any] [source]#
Returns a dictionary to be used as the default template namespace.
May be overridden by subclasses to add or modify values.
The results of this method will be combined with additional defaults in the tornado.template module and keyword arguments to render or render_string.
- get_template_path() Optional[str] [source]#
Override to customize template path for each handler.
By default, we use the
template_path
application setting. Return None to load templates relative to the calling file.
- get_user_locale() Optional[Locale] [source]#
Override to determine the locale from the authenticated user.
If None is returned, we fall back to get_browser_locale().
This method should return a tornado.locale.Locale object, most likely obtained via a call like
tornado.locale.get("en")
- property locale: Locale#
The locale for the current session.
Determined by either get_user_locale, which you can override to set the locale based on, e.g., a user preference stored in a database, or get_browser_locale, which uses the
Accept-Language
header.
- log_exception(typ: Optional[Type[BaseException]], value: Optional[BaseException], tb: Optional[TracebackType]) None [source]#
Override to customize logging of uncaught exceptions.
By default logs instances of HTTPError as warnings without stack traces (on the
tornado.general
logger), and all other exceptions as errors with stack traces (on thetornado.application
logger).New in version 3.1.
- on_connection_close() None [source]#
Called in async handlers if the client closed the connection.
Override this to clean up resources associated with long-lived connections. Note that this method is called only if the connection was closed during asynchronous processing; if you need to do cleanup after every request override on_finish instead.
Proxies may keep a connection open for a time (perhaps indefinitely) after the client has gone away, so this method may not be called promptly after the end user closes their connection.
- on_finish() None [source]#
Called after the end of a request.
Override this method to perform cleanup, logging, etc. This method is a counterpart to prepare.
on_finish
may not produce any output, as it is called after the response has been sent to the client.
- redirect(url: str, permanent: bool = False, status: Optional[int] = None) None [source]#
Sends a redirect to the given (optionally relative) URL.
If the
status
argument is specified, that value is used as the HTTP status code; otherwise either 301 (permanent) or 302 (temporary) is chosen based on thepermanent
argument. The default is 302 (temporary).
- render(*args, **kwargs)[source]#
Renders the template with the given arguments as the response.
render()
callsfinish()
, so no other output methods can be called after it.Returns a .Future with the same semantics as the one returned by finish. Awaiting this .Future is optional.
Changed in version 5.1: Now returns a .Future instead of
None
.
- render_embed_css(css_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded css for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_embed_js(js_embed: Iterable[bytes]) bytes [source]#
Default method used to render the final embedded js for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_css(css_files: Iterable[str]) str [source]#
Default method used to render the final css links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_linked_js(js_files: Iterable[str]) str [source]#
Default method used to render the final js links for the rendered webpage.
Override this method in a sub-classed controller to change the output.
- render_string(template_name: str, **kwargs: Any) bytes [source]#
Generate the given template with the given arguments.
We return the generated byte string (in utf8). To generate and write a template as a response, use render() above.
- require_setting(name: str, feature: str = 'this feature') None [source]#
Raises an exception if the given app setting is not defined.
- send_error(status_code: int = 500, **kwargs: Any) None [source]#
Sends the given HTTP error code to the browser.
If flush() has already been called, it is not possible to send an error, so this method will simply terminate the response. If output has been written but not yet flushed, it will be discarded and replaced with the error page.
Override write_error() to customize the error page that is returned. Additional keyword arguments are passed through to write_error.
- set_cookie(name: str, value: Union[str, bytes], domain: Optional[str] = None, expires: Optional[Union[float, Tuple, datetime]] = None, path: str = '/', expires_days: Optional[float] = None, *, max_age: Optional[int] = None, httponly: bool = False, secure: bool = False, samesite: Optional[str] = None, **kwargs: Any) None [source]#
Sets an outgoing cookie name/value with the given options.
Newly-set cookies are not immediately visible via get_cookie; they are not present until the next request.
Most arguments are passed directly to http.cookies.Morsel directly. See https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Set-Cookie for more information.
expires
may be a numeric timestamp as returned by time.time, a time tuple as returned by time.gmtime, or a datetime.datetime object.expires_days
is provided as a convenience to set an expiration time in days from today (if both are set,expires
is used).Deprecated since version 6.3: Keyword arguments are currently accepted case-insensitively. In Tornado 7.0 this will be changed to only accept lowercase arguments.
- set_default_headers() None [source]#
Override this to set HTTP headers at the beginning of the request.
For example, this is the place to set a custom
Server
header. Note that setting such headers in the normal flow of request processing may not do what you want, since headers may be reset during error handling.
- set_etag_header() None [source]#
Sets the response’s Etag header using
self.compute_etag()
.Note: no header will be set if
compute_etag()
returnsNone
.This method is called automatically when the request is finished.
- set_header(name: str, value: Union[bytes, str, int, Integral, datetime]) None [source]#
Sets the given response header name and value.
All header values are converted to strings (datetime objects are formatted according to the HTTP specification for the
Date
header).
- set_secure_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_signed_cookie(name: str, value: Union[str, bytes], expires_days: Optional[float] = 30, version: Optional[int] = None, **kwargs: Any) None [source]#
Signs and timestamps a cookie so it cannot be forged.
You must specify the
cookie_secret
setting in your Application to use this method. It should be a long, random sequence of bytes to be used as the HMAC secret for the signature.To read a cookie set with this method, use get_signed_cookie().
Note that the
expires_days
parameter sets the lifetime of the cookie in the browser, but is independent of themax_age_days
parameter to get_signed_cookie. A value of None limits the lifetime to the current browser session.Secure cookies may contain arbitrary byte values, not just unicode strings (unlike regular cookies)
Similar to set_cookie, the effect of this method will not be seen until the following request.
Changed in version 3.2.1: Added the
version
argument. Introduced cookie version 2 and made it the default.Changed in version 6.3: Renamed from
set_secure_cookie
toset_signed_cookie
to avoid confusion with other uses of “secure” in cookie attributes and prefixes. The old name remains as an alias.
- set_status(status_code: int, reason: Optional[str] = None) None [source]#
Sets the status code for our response.
- Parameters:
status_code (int) – Response status code.
reason (str) – Human-readable reason phrase describing the status code. If
None
, it will be filled in from http.client.responses or “Unknown”.
Changed in version 5.0: No longer validates that the response code is in http.client.responses.
- property settings: Dict[str, Any]#
An alias for self.application.settings <Application.settings>.
- static_url(path: str, include_host: Optional[bool] = None, **kwargs: Any) str [source]#
Returns a static URL for the given relative static file path.
This method requires you set the
static_path
setting in your application (which specifies the root directory of your static files).This method returns a versioned url (by default appending
?v=<signature>
), which allows the static files to be cached indefinitely. This can be disabled by passinginclude_version=False
(in the default implementation; other static file implementations are not required to support this, but they may support other options).By default this method returns URLs relative to the current host, but if
include_host
is true the URL returned will be absolute. If this handler has aninclude_host
attribute, that value will be used as the default for all static_url calls that do not passinclude_host
as a keyword argument.
- write(chunk: Union[str, bytes, dict]) None [source]#
Writes the given chunk to the output buffer.
To write the output to the network, use the flush() method below.
If the given chunk is a dictionary, we write it as JSON and set the Content-Type of the response to be
application/json
. (if you want to send JSON as a differentContent-Type
, callset_header
after callingwrite()
).Note that lists are not converted to JSON because of a potential cross-site security vulnerability. All JSON output should be wrapped in a dictionary. More details at http://haacked.com/archive/2009/06/25/json-hijacking.aspx/ and facebook/tornado#1009
- write_error(status_code: int, **kwargs: Any) None [source]#
Override to implement custom error pages.
write_error
may call write, render, set_header, etc to produce output as usual.If this error was caused by an uncaught exception (including HTTPError), an
exc_info
triple will be available askwargs["exc_info"]
. Note that this exception may not be the “current” exception for purposes of methods likesys.exc_info()
ortraceback.format_exc
.
- xsrf_form_html() str [source]#
An HTML
<input/>
element to be included with all POST forms.It defines the
_xsrf
input value, which we check on all POST requests to prevent cross-site request forgery. If you have set thexsrf_cookies
application setting, you must include this HTML within all of your HTML forms.In a template, this method should be called with
{% module xsrf_form_html() %}
See check_xsrf_cookie() above for more information.
- property xsrf_token: bytes#
The XSRF-prevention token for the current user/session.
To prevent cross-site request forgery, we set an ‘_xsrf’ cookie and include the same ‘_xsrf’ value as an argument with all POST requests. If the two do not match, we reject the form submission as a potential forgery.
See http://en.wikipedia.org/wiki/Cross-site_request_forgery
This property is of type bytes, but it contains only ASCII characters. If a character string is required, there is no need to base64-encode it; just decode the byte string as UTF-8.
Changed in version 3.2.2: The xsrf token will now be have a random mask applied in every request, which makes it safe to include the token in pages that are compressed. See http://breachattack.com for more information on the issue fixed by this change. Old (version 1) cookies will be converted to version 2 when this method is called unless the
xsrf_cookie_version
Application setting is set to 1.Changed in version 4.3: The
xsrf_cookie_kwargs
Application setting may be used to supply additional cookie options (which will be passed directly to set_cookie). For example,xsrf_cookie_kwargs=dict(httponly=True, secure=True)
will set thesecure
andhttponly
flags on the_xsrf
cookie.
- class panel.io.server.Server(*args, **kwargs)[source]#
Bases:
Server
- property address: str | None#
The configured address that the server listens on for HTTP requests.
- get_session(app_path: str, session_id: ID) ServerSession [source]#
Get an active a session by name application path and session ID.
- Parameters:
app_path (str) – The configured application path for the application to return a session for.
session_id (str) – The session ID of the session to retrieve.
- Returns:
ServerSession
- get_sessions(app_path: str | None = None) list[ServerSession] [source]#
Gets all currently active sessions for applications.
- Parameters:
app_path (str, optional) – The configured application path for the application to return sessions for. If None, return active sessions for all applications. (default: None)
- Returns:
list[ServerSession]
- property index: str | None#
A path to a Jinja2 template to use for index at “/”
- property io_loop: IOLoop#
The Tornado
IOLoop
that this Bokeh Server is running on.
- property port: int | None#
The configured port number that the server listens on for HTTP requests.
- property prefix: str#
The configured URL prefix to use for all Bokeh server paths.
- run_until_shutdown() None [source]#
Run the Bokeh Server until shutdown is requested by the user, either via a Keyboard interrupt (Ctrl-C) or SIGTERM.
Calling this method will start the Tornado
IOLoop
and block all execution in the calling process.- Returns:
None
- show(app_path: str, browser: str | None = None, new: BrowserTarget = 'tab') None [source]#
Opens an app in a browser window or tab.
This method is useful for testing or running Bokeh server applications on a local machine but should not call when running Bokeh server for an actual deployment.
- Parameters:
app_path (str) – the app path to open The part of the URL after the hostname:port, with leading slash.
browser (str, optional) – browser to show with (default: None) For systems that support it, the browser argument allows specifying which browser to display in, e.g. “safari”, “firefox”, “opera”, “windows-default” (see the webbrowser module documentation in the standard lib for more details).
new (str, optional) – window or tab (default: “tab”) If
new
is ‘tab’, then opens a new tab. Ifnew
is ‘window’, then opens a new window.
- Returns:
None
- start() None [source]#
Install the Bokeh Server and its background tasks on a Tornado
IOLoop
.This method does not block and does not affect the state of the Tornado
IOLoop
You must start and stop the loop yourself, i.e. this method is typically useful when you are already explicitly managing anIOLoop
yourself.To start a Bokeh server and immediately “run forever” in a blocking manner, see
run_until_shutdown()
.
- stop(wait: bool = True) None [source]#
Stop the Bokeh Server.
This stops and removes all Bokeh Server
IOLoop
callbacks, as well as stops theHTTPServer
that this instance was configured with.- Parameters:
fast (bool) – Whether to wait for orderly cleanup (default: True)
- Returns:
None
- property unix_socket: str#
The configured unix socket that the server listens to.
- class panel.io.server.StoppableThread(io_loop: IOLoop, **kwargs)[source]#
Bases:
Thread
Thread class with a stop() method.
- property daemon#
A boolean value indicating whether this thread is a daemon thread.
This must be set before start() is called, otherwise RuntimeError is raised. Its initial value is inherited from the creating thread; the main thread is not a daemon thread and therefore all threads created in the main thread default to daemon = False.
The entire Python program exits when only daemon threads are left.
- getName()[source]#
Return a string used for identification purposes only.
This method is deprecated, use the name attribute instead.
- property ident#
Thread identifier of this thread or None if it has not been started.
This is a nonzero integer. See the get_ident() function. Thread identifiers may be recycled when a thread exits and another thread is created. The identifier is available even after the thread has exited.
- isDaemon()[source]#
Return whether this thread is a daemon.
This method is deprecated, use the daemon attribute instead.
- is_alive()[source]#
Return whether the thread is alive.
This method returns True just before the run() method starts until just after the run() method terminates. See also the module function enumerate().
- join(timeout=None)[source]#
Wait until the thread terminates.
This blocks the calling thread until the thread whose join() method is called terminates – either normally or through an unhandled exception or until the optional timeout occurs.
When the timeout argument is present and not None, it should be a floating point number specifying a timeout for the operation in seconds (or fractions thereof). As join() always returns None, you must call is_alive() after join() to decide whether a timeout happened – if the thread is still alive, the join() call timed out.
When the timeout argument is not present or None, the operation will block until the thread terminates.
A thread can be join()ed many times.
join() raises a RuntimeError if an attempt is made to join the current thread as that would cause a deadlock. It is also an error to join() a thread before it has been started and attempts to do so raises the same exception.
- property name#
A string used for identification purposes only.
It has no semantics. Multiple threads may be given the same name. The initial name is set by the constructor.
- property native_id#
Native integral thread ID of this thread, or None if it has not been started.
This is a non-negative integer. See the get_native_id() function. This represents the Thread ID as reported by the kernel.
- run() None [source]#
Method representing the thread’s activity.
You may override this method in a subclass. The standard run() method invokes the callable object passed to the object’s constructor as the target argument, if any, with sequential and keyword arguments taken from the args and kwargs arguments, respectively.
- setDaemon(daemonic)[source]#
Set whether this thread is a daemon.
This method is deprecated, use the .daemon property instead.
- panel.io.server.async_execute(func: Callable[[...], None]) None [source]#
Wrap async event loop scheduling to ensure that with_lock flag is propagated from function to partial wrapping it.
- panel.io.server.get_server(panel: TViewableFuncOrPath | Mapping[str, TViewableFuncOrPath], port: int = 0, address: Optional[str] = None, websocket_origin: Optional[str | list[str]] = None, loop: Optional[IOLoop] = None, show: bool = False, start: bool = False, title: bool = None, verbose: bool = False, location: bool | Location = True, admin: bool = False, static_dirs: Mapping[str, str] = {}, basic_auth: str = None, oauth_provider: Optional[str] = None, oauth_key: Optional[str] = None, oauth_secret: Optional[str] = None, oauth_redirect_uri: Optional[str] = None, oauth_extra_params: Mapping[str, str] = {}, oauth_error_template: Optional[str] = None, cookie_secret: Optional[str] = None, oauth_encryption_key: Optional[str] = None, oauth_jwt_user: Optional[str] = None, oauth_refresh_tokens: Optional[bool] = None, oauth_guest_endpoints: Optional[bool] = None, oauth_optional: Optional[bool] = None, login_endpoint: Optional[str] = None, logout_endpoint: Optional[str] = None, login_template: Optional[str] = None, logout_template: Optional[str] = None, session_history: Optional[int] = None, liveness: bool | str = False, warm: bool = False, **kwargs) Server [source]#
Returns a Server instance with this panel attached as the root app.
- Parameters:
panel (Viewable, function or {str: Viewable}) – A Panel object, a function returning a Panel object or a dictionary mapping from the URL slug to either.
port (int (optional, default=0)) – Allows specifying a specific port
address (str) – The address the server should listen on for HTTP requests.
websocket_origin (str or list(str) (optional)) –
A list of hosts that can connect to the websocket.
This is typically required when embedding a server app in an external web site.
If None, “localhost” is used.
loop (tornado.ioloop.IOLoop (optional, default=IOLoop.current())) – The tornado IOLoop to run the Server on.
show (boolean (optional, default=False)) – Whether to open the server in a new browser tab on start.
start (boolean(optional, default=False)) – Whether to start the Server.
title (str or {str: str} (optional, default=None)) – An HTML title for the application or a dictionary mapping from the URL slug to a customized title.
verbose (boolean (optional, default=False)) – Whether to report the address and port.
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
admin (boolean (default=False)) – Whether to enable the admin panel
static_dirs (dict (optional, default={})) – A dictionary of routes and local paths to serve as static file directories on those routes.
basic_auth (str (optional, default=None)) – Password or filepath to use with basic auth provider.
oauth_provider (str) – One of the available OAuth providers
oauth_key (str (optional, default=None)) – The public OAuth identifier
oauth_secret (str (optional, default=None)) – The client secret for the OAuth provider
oauth_redirect_uri (Optional[str] = None,) – Overrides the default OAuth redirect URI
oauth_jwt_user (Optional[str] = None,) – Key that identifies the user in the JWT id_token.
oauth_extra_params (dict (optional, default={})) – Additional information for the OAuth provider
oauth_error_template (str (optional, default=None)) – Jinja2 template used when displaying authentication errors.
cookie_secret (str (optional, default=None)) – A random secret string to sign cookies (required for OAuth)
oauth_encryption_key (str (optional, default=None)) – A random encryption key used for encrypting OAuth user information and access tokens.
oauth_guest_endpoints (list (optional, default=None)) – List of endpoints that can be accessed as a guest without authenticating.
oauth_optional (bool (optional, default=None)) – Whether the user will be forced to go through login flow or if they can access all applications as a guest.
oauth_refresh_tokens (bool (optional, default=None)) – Whether to automatically refresh OAuth access tokens when they expire.
login_endpoint (str (optional, default=None)) – Overrides the default login endpoint /login
logout_endpoint (str (optional, default=None)) – Overrides the default logout endpoint /logout
logout_template (str (optional, default=None)) – Jinja2 template served when viewing the login endpoint when authentication is enabled.
logout_template – Jinja2 template served when viewing the logout endpoint when authentication is enabled.
session_history (int (optional, default=None)) – The amount of session history to accumulate. If set to non-zero and non-None value will launch a REST endpoint at /rest/session_info, which returns information about the session history.
liveness (bool | str (optional, default=False)) – Whether to add a liveness endpoint. If a string is provided then this will be used as the endpoint, otherwise the endpoint will be hosted at /liveness.
warm (bool (optional, default=False)) – Whether to run the applications before serving them to ensure all imports and caches are fully warmed up before serving the app.
kwargs (dict) – Additional keyword arguments to pass to Server instance.
- Returns:
server – Bokeh Server instance running this panel
- Return type:
- panel.io.server.get_static_routes(static_dirs)[source]#
Returns a list of tornado routes of StaticFileHandlers given a dictionary of slugs and file paths to serve.
- panel.io.server.html_page_for_render_items(bundle: Bundle | tuple[str, str], docs_json: dict[ID, DocJson], render_items: list[RenderItem], title: str, template: Template | str | None = None, template_variables: dict[str, Any] = {}) str [source]#
Render an HTML page from a template and Bokeh render items.
- Parameters:
(tuple) (bundle) – A tuple containing (bokehjs, bokehcss)
(JSON-like) (docs_json) – Serialized Bokeh Document
(RenderItems) (render_items) – Specific items to render from the document and where
None) (title (str or) – A title for the HTML page. If None, DEFAULT_TITLE is used
None (template (str or Template or) – A Template to be used for the HTML page. If None, FILE is used.
optional) – A Template to be used for the HTML page. If None, FILE is used.
(dict (template_variables) – Any Additional variables to pass to the template
optional) – Any Additional variables to pass to the template
- Return type:
str
- panel.io.server.serve(panels: TViewableFuncOrPath | Mapping[str, TViewableFuncOrPath], port: int = 0, address: Optional[str] = None, websocket_origin: Optional[str | list[str]] = None, loop: Optional[IOLoop] = None, show: bool = True, start: bool = True, title: Optional[str] = None, verbose: bool = True, location: bool = True, threaded: bool = False, admin: bool = False, **kwargs) StoppableThread | Server [source]#
Allows serving one or more panel objects on a single server. The panels argument should be either a Panel object or a function returning a Panel object or a dictionary of these two. If a dictionary is supplied the keys represent the slugs at which each app is served, e.g. serve({‘app’: panel1, ‘app2’: panel2}) will serve apps at /app and /app2 on the server.
Reference: https://panel.holoviz.org/user_guide/Server_Configuration.html#serving-multiple-apps
- Parameters:
panel (Viewable, function or {str: Viewable or function}) – A Panel object, a function returning a Panel object or a dictionary mapping from the URL slug to either.
port (int (optional, default=0)) – Allows specifying a specific port
address (str) – The address the server should listen on for HTTP requests.
websocket_origin (str or list(str) (optional)) –
A list of hosts that can connect to the websocket.
This is typically required when embedding a server app in an external web site.
If None, “localhost” is used.
loop (tornado.ioloop.IOLoop (optional, default=IOLoop.current())) – The tornado IOLoop to run the Server on
show (boolean (optional, default=True)) – Whether to open the server in a new browser tab on start
start (boolean(optional, default=True)) – Whether to start the Server
title (str or {str: str} (optional, default=None)) – An HTML title for the application or a dictionary mapping from the URL slug to a customized title
verbose (boolean (optional, default=True)) – Whether to print the address and port
location (boolean or panel.io.location.Location) – Whether to create a Location component to observe and set the URL location.
threaded (boolean (default=False)) – Whether to start the server on a new Thread
admin (boolean (default=False)) – Whether to enable the admin panel
kwargs (dict) – Additional keyword arguments to pass to Server instance
session
Module#

- class panel.io.session.ServerSessionStub(session_id: ID, document: Document, io_loop: IOLoop | None = None, token: str | None = None)[source]#
Bases:
ServerSession
Stubs out ServerSession methods since the session is only used for warming up the cache.
- classmethod patch(message: msg.patch_doc, connection: ServerConnection) msg.ok [source]#
Handle a PATCH-DOC, return a Future with work to be scheduled.
- classmethod pull(message: msg.pull_doc_req, connection: ServerConnection) msg.pull_doc_reply [source]#
Handle a PULL-DOC, return a Future with work to be scheduled.
- classmethod push(message: msg.push_doc, connection: ServerConnection) msg.ok [source]#
Handle a PUSH-DOC, return a Future with work to be scheduled.
- request_expiration() None [source]#
Used in test suite for now. Forces immediate expiration if no connections.
- subscribe(connection: ServerConnection) None [source]#
This should only be called by
ServerConnection.subscribe_session
or our book-keeping will be broken
- property token: str#
A JWT token to authenticate the session.
state
Module#
Various utilities for recording and embedding state in a rendered app.